Given an array of size N. The array is initially all zeros. The task is to count. The number of 1's in the array after N moves. Each Nth step has an associated rule. The rule is -
The first move-change the element at position 1, 2, 3, 4………….
th The second move - change the elements at positions 2, 4, 6, 8... ……..
Count the number of 1’s in the last array.
We understand through examples.
Arr[]={ 0,0,0,0 } N=4
Output
Number of 1s in the array after N moves − 2
Explanation - Array after subsequent movement-
Move 1: { 1,1,1,1 } Move 2: { 1,0,1,0 } Move 3: { 1,0,0,3 } Move 4: { 1,0,0,1 } Number of ones in the final array is 2.
Input
Arr[]={ 0,0,0,0,0,0} N=6
Output
Number of 1s in the array after N moves − 2
Explanation - Array after subsequent movement-
Move 1: { 1,1,1,1,1,1,1 } Move 2: { 1,0,1,0,1,0,1 } Move 3: { 1,0,0,1,0,0,1 } Move 4: { 1,0,0,0,1,0,0 } Move 5: { 1,0,0,0,0,1,0 } Move 4: { 1,0,0,0,0,0,1 } Number of ones in the final array is 2.
The method used in the following program is as follows
We use an integer array Arr[] initialized with 0 and an integer N.
Function Onecount takes an Arr[] and its size N as input and returns no. The number in the final array after N moves.
The for loop starts from 1 and goes to the end of the array.
Each i represents step i.
Nested for loop starts at index 0 and goes to the end of the array.
For each i-th move, if index j is a multiple of i (j%i==0), replace 0 at that position with 1.
Continue this process for each i until the end of the array.
- Note - Indexing starts at i=1,j=1, but array indexing goes from 0 to N-1. So arr[j1] will be converted every time.
Finally traverse the entire array again, counting no. It contains 1 and is stored in the count.
- Returns the count of the desired results. Example
- Live Demonstration
#include <stdio.h> int Onecount(int arr[], int N){ for (int i = 1; i <= N; i++) { for (int j = i; j <= N; j++) { // If j is divisible by i if (j % i == 0) { if (arr[j - 1] == 0) arr[j - 1] = 1; // Convert 0 to 1 else arr[j - 1] = 0; // Convert 1 to 0 } } } int count = 0; for (int i = 0; i < N; i++) if (arr[i] == 1) count++; // count number of 1's return count; } int main(){ int size = 6; int Arr[6] = { 0 }; printf("Number of 1s in the array after N moves: %d", Onecount(Arr, size)); return 0; }
Output
If we run the above code, it will generate the following output-
Number of 1s in the array after N moves: 2
The above is the detailed content of In C language, count the number of 1's in the array after N moves. For more information, please follow other related articles on the PHP Chinese website!
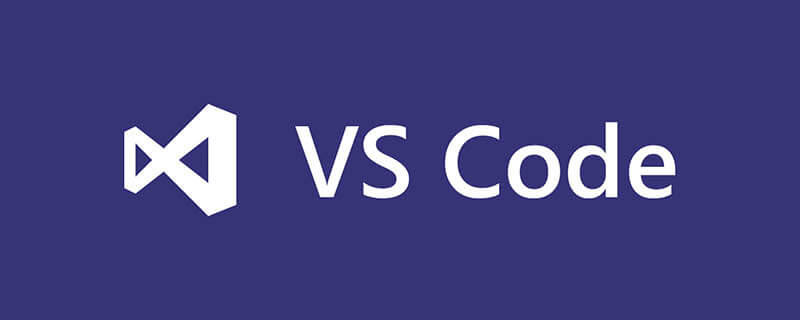
VScode中怎么配置C语言环境?下面本篇文章给大家介绍一下VScode配置C语言环境的方法(超详细),希望对大家有所帮助!
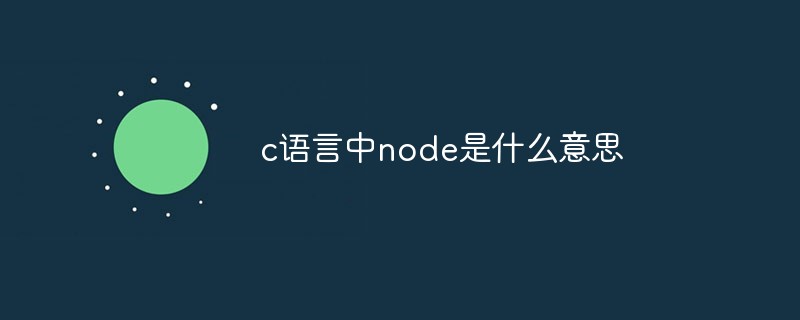
在C语言中,node是用于定义链表结点的名称,通常在数据结构中用作结点的类型名,语法为“struct Node{...};”;结构和类在定义出名称以后,直接用该名称就可以定义对象,C语言中还存在“Node * a”和“Node* &a”。

c语言将数字转换成字符串的方法:1、ascii码操作,在原数字的基础上加“0x30”,语法“数字+0x30”,会存储数字对应的字符ascii码;2、使用itoa(),可以把整型数转换成字符串,语法“itoa(number1,string,数字);”;3、使用sprintf(),可以能够根据指定的需求,格式化内容,存储至指针指向的字符串。
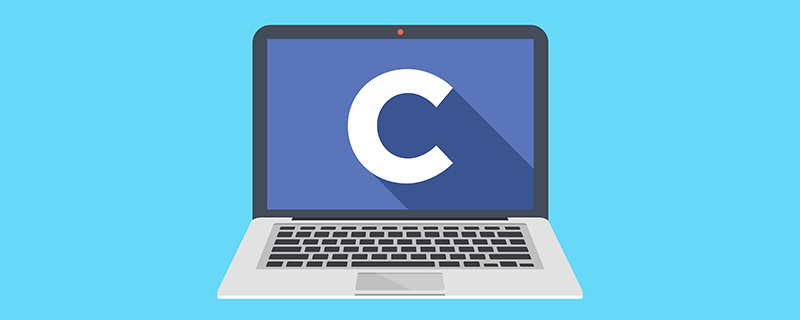
在c语言中,没有开根号运算符,开根号使用的是内置函数“sqrt()”,使用语法“sqrt(数值x)”;例如“sqrt(4)”,就是对4进行平方根运算,结果为2。sqrt()是c语言内置的开根号运算函数,其运算结果是函数变量的算术平方根;该函数既不能运算负数值,也不能输出虚数结果。

C语言数组初始化的三种方式:1、在定义时直接赋值,语法“数据类型 arrayName[index] = {值};”;2、利用for循环初始化,语法“for (int i=0;i<3;i++) {arr[i] = i;}”;3、使用memset()函数初始化,语法“memset(arr, 0, sizeof(int) * 3)”。
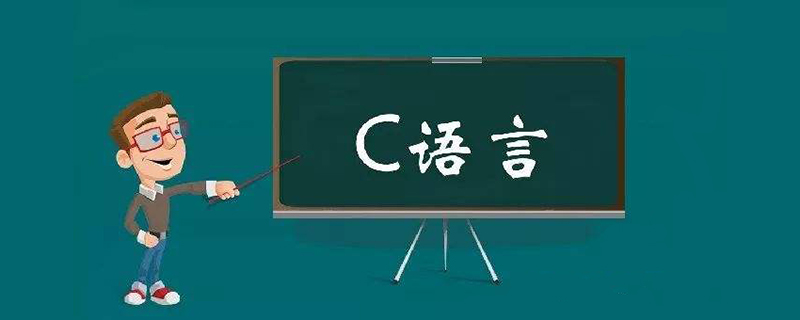
c语言合法标识符的要求是:1、标识符只能由字母(A~Z, a~z)、数字(0~9)和下划线(_)组成;2、第一个字符必须是字母或下划线,不能是数字;3、标识符中的大小写字母是有区别的,代表不同含义;4、标识符不能是关键字。
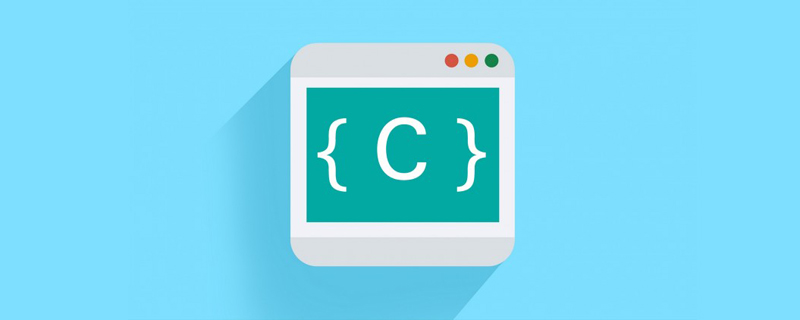
c语言编译后生成“.OBJ”的二进制文件(目标文件)。在C语言中,源程序(.c文件)经过编译程序编译之后,会生成一个后缀为“.OBJ”的二进制文件(称为目标文件);最后还要由称为“连接程序”(Link)的软件,把此“.OBJ”文件与c语言提供的各种库函数连接在一起,生成一个后缀“.EXE”的可执行文件。
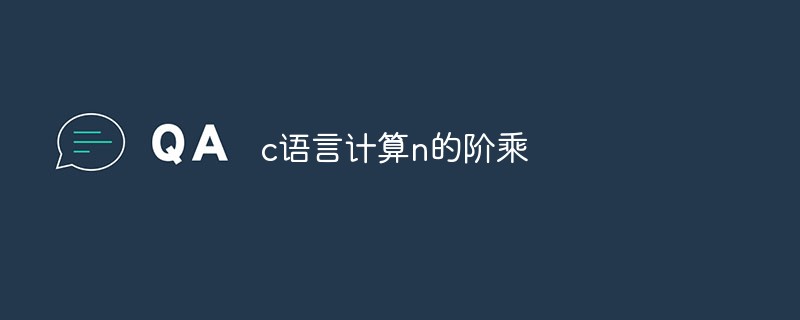
c语言计算n的阶乘的方法:1、通过for循环计算阶乘,代码如“for (i = 1; i <= n; i++){fact *= i;}”;2、通过while循环计算阶乘,代码如“while (i <= n){fact *= i;i++;}”;3、通过递归方式计算阶乘,代码如“ int Fact(int n){int res = n;if (n > 1)res...”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
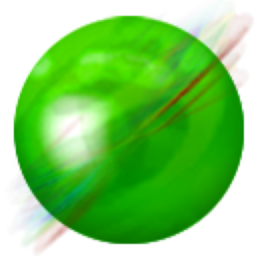
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
