Introduction to disjoint set data structure or union-find algorithm
The disjoint set information structure, also known as the union search algorithm, is probably a fundamental concept in computer science that provides an effective method for solving problems related to allocation and networking . It is particularly valuable for solving problems involving sets of components and determining their connections. In this article, we will look at language constructs, algorithms, and two unique ways to implement disjoint set information structures in C. We will also provide fully executable code examples illustrating these methods.
grammar
Before we delve into the algorithm, let’s familiarize ourselves with the syntax used in the following code examples -
// Create a disjoint set DisjointSet ds(n); // Perform union operation ds.unionSets(a, b); // Find the representative of a set ds.findSet(x);
algorithm
Utilizing disjoint data structures can be very useful when dealing with multiple disjoint collections. Each individual grouping is assigned a specific representative to characterize it. The starting point involves each component forming its own isolated set, which corresponds to its respective representative (which also happens to be itself). The two main operations performed on disjoint sets are union and search.
Joint operation
Find the representatives of the two sets to be merged.
If the representatives are different, make one representative point to the other, effectively merging the sets.
If the representatives are the same, the sets have been merged and no further action is required.
Find operation
Given an element, find the representative of the set it belongs to.
Follow the parent pointer until it reaches the representative node.
Return the delegate as the result.
Method 1: Rank-based merging and path compression
An effective way to implement disjoint set data structures is to use rank-wise union and path compression techniques.
In this method, each collection has an associated rank, which is initially set to 0.
When performing a union operation between two sets, the higher-ranked set is given priority and the lower-ranked set is merged. If two sets have similar ranks, one must arbitrarily choose which set contains whom. In either case, once merged into a new set, its rank increases by 1. Additionally, to speed up lookup operations and reduce time complexity, path compression helps flatten the tree structure during these operations.
The Chinese translation ofExample
is:Example
#include <iostream> #include <vector> class DisjointSet { std::vector<int> parent; std::vector<int> rank; public: DisjointSet(int n) { parent.resize(n); rank.resize(n, 0); for (int i = 0; i < n; ++i) parent[i] = i; } int findSet(int x) { if (parent[x] != x) parent[x] = findSet(parent[x]); return parent[x]; } void unionSets(int x, int y) { int xRoot = findSet(x); int yRoot = findSet(y); if (xRoot == yRoot) return; if (rank[xRoot] < rank[yRoot]) parent[xRoot] = yRoot; else if (rank[xRoot] > rank[yRoot]) parent[yRoot] = xRoot; else { parent[yRoot] = xRoot; rank[xRoot]++; } } }; int main() { // Example usage of DisjointSet int n = 5; // Number of elements DisjointSet ds(n); ds.unionSets(0, 1); ds.unionSets(2, 3); ds.unionSets(3, 4); std::cout << ds.findSet(0) << std::endl; std::cout << ds.findSet(2) << std::endl; return 0; }
Output
0 2
Method 2: Size-based merging with size and path compression
Another way to deal with disjoint set data structures is to use merge-by-size and path compression techniques.
In this method, each collection has an associated size, initially set to 1.
In a union operation, smaller sets are merged into larger sets.
The size of the result set will be updated accordingly.
Apply path compression during the seek operation to flatten the tree structure, similar to the previous method.
Example
is:Example
#include <iostream> #include <vector> class DisjointSet { std::vector<int> parent; std::vector<int> size; public: DisjointSet(int n) { parent.resize(n); size.resize(n, 1); for (int i = 0; i < n; ++i) parent[i] = i; } int findSet(int x) { if (parent[x] != x) parent[x] = findSet(parent[x]); return parent[x]; } void unionSets(int x, int y) { int xRoot = findSet(x); int yRoot = findSet(y); if (xRoot == yRoot) return; if (size[xRoot] < size[yRoot]) { parent[xRoot] = yRoot; size[yRoot] += size[xRoot]; } else { parent[yRoot] = xRoot; size[xRoot] += size[yRoot]; } } }; int main() { // Example usage of DisjointSet int n = 5; // Number of elements DisjointSet ds(n); ds.unionSets(0, 1); ds.unionSets(2, 3); ds.unionSets(3, 4); std::cout << ds.findSet(0) << std::endl; std::cout << ds.findSet(2) << std::endl; return 0; }
Output
0 2
in conclusion
The disjoint set data structure or union search algorithm is a powerful tool for solving problems involving sets and connectivity. This article extensively studies C's disjoint set data structure syntax and its algorithms. To extend our understanding, we provide readers with two unique approaches - ranking-based union combined with path compression, and size-based union via size plus path compression. By understanding and implementing these methods, you can effectively solve a variety of problems that require tracking disjoint sets.
The above is the detailed content of Introduction to disjoint set data structure or union-find algorithm. For more information, please follow other related articles on the PHP Chinese website!
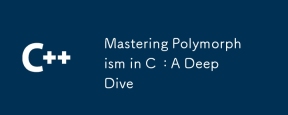
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
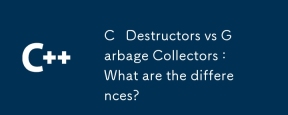
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
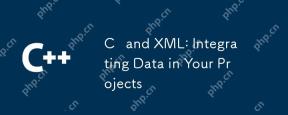
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
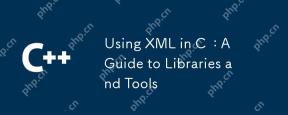
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
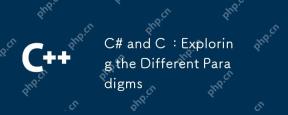
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
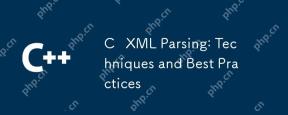
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
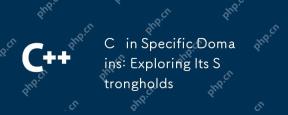
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
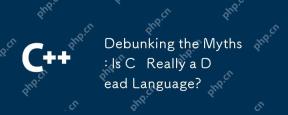
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
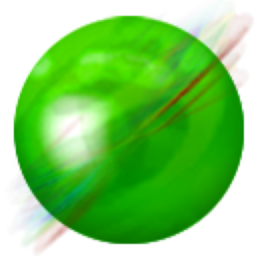
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
