


Design a queue data structure to obtain the minimum or maximum value in O(1) time
C has a deque header file that handles properties of stacks and queues. In data structures, solving the problem of O(1) time complexity requires constant time. By using a deque in this program, we get the advantages of using both a stack and a queue.
In this article, we will solve the queue data structure to get the minimum or maximum value of a number in O(1) time.
grammar
deque<data_type> name_of_queue;
parameter
deque - This is known as a deque, which orders a set of items or numbers equivalent to a queue.
data_type - The data type used, such as int, float, etc.
name_of_queue - Any name given to the queue, such as ab, cd, etc.
front()
front() is a predefined function in C STL, which directly refers to the first index position of the queue.
back()
back() is a predefined function in C STL, which directly refers to the last index position of the queue.
push_back()
push_back() is also a predefined function for inserting elements from behind.
algorithm
We will use the header files 'iostream' and 'deque' to start the program.
-
We insert into the deque to handle the maximum or minimum value of the number.
"deque
dq" - By using this we can enable properties of stacks and queues
Starting from the for loop, we insert elements in the range 10 to 15. Then use a method called 'push_back[i ]' which accepts 'i' as a parameter and uses a for loop to push the array elements.
We then create two variables using the predefined functions front() and back() to find the minimum and maximum values of a number. front() looks for the first index to represent the smallest number, while back() looks for the last index to represent the largest number.
Now we are initializing the for loop to iterate over the index number length and use that length to classify the comparison of the smallest and largest elements as 'dq[i]'. So this will find the minimum and maximum number.
-
Finally, we print the output of minimum and maximum length with the help of 'min_element' and 'max_element' variables.
李>
Example
In this program, we will solve the queue data structure to get the minimum and maximum values in O(1) time.
#include <iostream> #include <deque> using namespace std; int main() { deque<int> dq; // double ended queue // insert elements into the deque using a loop for(int i = 10; i <= 15; i++) { dq.push_back(i); } // find the minimum and maximum elements int min_element = dq.front(); int max_element = dq.back(); for(int i = 1; i < dq.size(); i++) { if(dq[i] < min_element) { min_element = dq[i]; } if(dq[i] > max_element) { max_element = dq[i]; } } //Print the minimum and maximum elements cout << "Minimum element: " << min_element << endl; cout << "Maximum element: " << max_element << endl; return 0; }
Output
Minimum element: 10 Maximum element: 15
in conclusion
We explored the concept of queue data structures to find the smallest or largest element. We saw how front() and back() can be used to find the minimum and maximum value of an element, and also how to add pushback to the end of an indexed element. By using a deque we can handle the problem in O(1) time complexity.
The above is the detailed content of Design a queue data structure to obtain the minimum or maximum value in O(1) time. For more information, please follow other related articles on the PHP Chinese website!
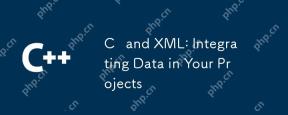
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
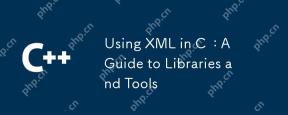
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
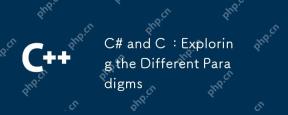
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
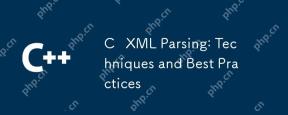
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
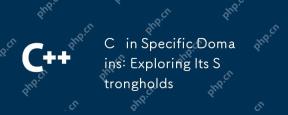
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
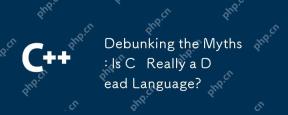
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
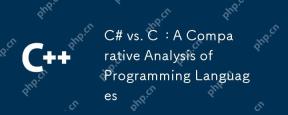
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
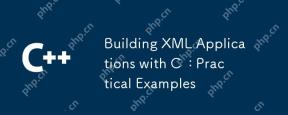
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
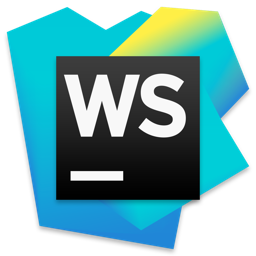
WebStorm Mac version
Useful JavaScript development tools
