Vue3+TS+Vite development skills: how to manage user rights
Vue3 TS Vite development tips: How to manage user rights
Introduction:
In modern web applications, user rights management is a crucial Function. By determining the user's role and permissions, we can restrict the user's access to different features and pages. With the combination of Vue3, TypeScript and Vite, we can manage user rights more efficiently. This article will introduce some practical tips and code examples to help you implement user rights management in Vue3 TS Vite.
- Define roles and permissions
Before performing user permission management, we first need to define different roles and permissions. The role can be admin (administrator), user (ordinary user), etc., and the permissions can be create (create), update (update) or delete (delete), etc. The following is a simple example of role and permission definition:
// roles.ts export enum Role { Admin = 'admin', User = 'user', } // permissions.ts export enum Permission { Create = 'create', Update = 'update', Delete = 'delete', }
- Create permission directive
In order to more conveniently display or hide certain elements in the Vue component, we can create a custom Define directives to check user permissions. The following is an example of a permission directive:
// directives/permission.ts import { Directive, DirectiveBinding } from 'vue' import { Role, Permission } from '@/constants/roles' const permissionDirective: Directive = (el: HTMLElement, binding: DirectiveBinding) => { const { value } = binding const userRole = 'admin' // 这里假设用户角色为admin,实际开发中需要根据实际情况获取 // 在这里检查用户权限和角色,决定是否展示元素 if (value) { const [requiredRole, requiredPermission] = value.split('.') if ( (requiredRole && requiredRole !== userRole) || (requiredPermission && !hasPermission(userRole, requiredPermission)) ) { el.style.display = 'none' } } } const hasPermission = (role: Role, permission: Permission): boolean => { // 在这里根据角色和权限检查用户是否有权限 // 实际开发中,可以从后端接口获取用户角色和权限,并做相应的校验 return true } export default permissionDirective
- Register permission directive
Register the permission directive in the application's entry file. The following is an example:
// main.ts import { createApp } from 'vue' import App from './App.vue' import permissionDirective from '@/directives/permission' const app = createApp(App) app.directive('permission', permissionDirective) app.mount('#app')
- Using permission directives
In Vue components, we can use permission directives to control the display or hiding of elements. Here is an example:
<template> <div> <h1 id="仅管理员可见">仅管理员可见</h1> <h1 id="仅普通用户可见">仅普通用户可见</h1> </div> </template>
In the above example, the first
tag will only be displayed if the user role is admin and has create permissions. Similarly, the second tag will only be displayed if the user role is user and has the update permission. - Dynamic Routing Permission Management
In actual projects, it is often necessary to dynamically generate routes based on user permissions. We can check the user's permissions in the route navigation guard and dynamically add, delete or redirect routes based on the permissions. The following is an example of using Vue Router for dynamic routing permission management:
// router.ts
import { createRouter, createWebHistory } from 'vue-router'
import { Role, Permission } from '@/constants/roles'
import { hasPermission } from '@/utils/permission'
const routes = [
{
path: '/admin',
name: 'admin',
component: () => import('@/views/Admin.vue')
meta: {
requiresAuth: true,
requiredRoles: [Role.Admin],
},
},
// ...
]
const router = createRouter({
history: createWebHistory(),
routes,
})
router.beforeEach((to, from, next) => {
const isLoggedIn = true // 假设用户已登录,实际开发中需要根据实际情况获取
if (to.meta.requiresAuth && !isLoggedIn) {
next('/login')
} else {
const userRole = 'admin' // 这里假设用户角色为admin,实际开发中需要根据实际情况获取
if (to.meta.requiredRoles && !hasPermission(userRole, to.meta.requiredRoles)) {
next('/error')
} else {
next()
}
}
})
export default router
- Dynamic Routing Permission Management
In actual projects, it is often necessary to dynamically generate routes based on user permissions. We can check the user's permissions in the route navigation guard and dynamically add, delete or redirect routes based on the permissions. The following is an example of using Vue Router for dynamic routing permission management:
// router.ts import { createRouter, createWebHistory } from 'vue-router' import { Role, Permission } from '@/constants/roles' import { hasPermission } from '@/utils/permission' const routes = [ { path: '/admin', name: 'admin', component: () => import('@/views/Admin.vue') meta: { requiresAuth: true, requiredRoles: [Role.Admin], }, }, // ... ] const router = createRouter({ history: createWebHistory(), routes, }) router.beforeEach((to, from, next) => { const isLoggedIn = true // 假设用户已登录,实际开发中需要根据实际情况获取 if (to.meta.requiresAuth && !isLoggedIn) { next('/login') } else { const userRole = 'admin' // 这里假设用户角色为admin,实际开发中需要根据实际情况获取 if (to.meta.requiredRoles && !hasPermission(userRole, to.meta.requiredRoles)) { next('/error') } else { next() } } }) export default router
In the above example, we first check whether the user is logged in. We then get the user roles and match them against the "requiredRoles" of the route. If the user role does not meet the requirements, redirect to an error page. Otherwise, we continue loading the requested route.
Conclusion:
With the powerful capabilities of Vue3, TypeScript and Vite, we can manage user rights more efficiently. By defining roles and permissions, creating permission directives, and using dynamic routing permission management, we can easily implement user permission control. The above examples hope to help you implement user rights management in your Vue3 TS Vite project.
The above is the detailed content of Vue3+TS+Vite development skills: how to manage user rights. For more information, please follow other related articles on the PHP Chinese website!

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
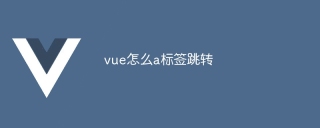
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
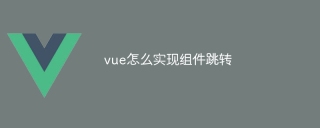
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.
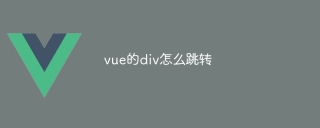
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
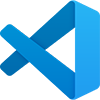
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
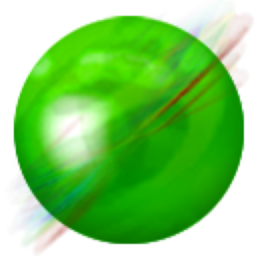
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
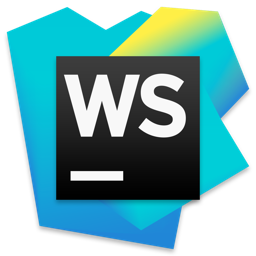
WebStorm Mac version
Useful JavaScript development tools