How to avoid common mistakes in PHP development
How to avoid common mistakes in PHP development
Introduction:
In PHP development, we often encounter some common mistakes. These errors can lead to abnormal program functionality, poor performance, or even security vulnerabilities. To avoid these mistakes, this article will cover some common mistakes and how to avoid them in PHP development. This article will give some code examples to explain better.
1. Prevent SQL injection attacks
SQL injection attacks are a common form of network attack, which cause database leaks or directly attack the database by injecting malicious code into SQL queries. Here are some best practices to prevent SQL injection attacks:
1.1 Use parameterized queries or prepared statements:
$mysqli = new mysqli("localhost", "username", "password", "database"); $stmt = $mysqli->prepare("SELECT * FROM users WHERE username = ?"); $stmt->bind_param("s", $username); $stmt->execute();
1.2 Use secure filtering functions:
$username = mysqli_real_escape_string($conn, $_POST['username']); $password = hash('sha256', $_POST['password']);
2. Prevent cross-site scripting attacks (XSS)
Cross-site scripting attacks are attacks that obtain sensitive information or perform malicious operations by inserting malicious scripts into web applications. Here are some best practices to prevent XSS attacks:
2.1 Filter and validate user input:
$username = htmlspecialchars($_POST['username'], ENT_QUOTES, 'UTF-8'); $email = filter_var($_POST['email'], FILTER_VALIDATE_EMAIL);
2.2 Use secure output functions:
echo htmlentities($output, ENT_QUOTES, 'UTF-8');
3. Avoid errors Error handling
In PHP development, error handling is very important. Improper error handling may lead to the leakage of sensitive information or cause program anomalies. The following are some correct error handling methods:
3.1 Use error reporting settings:
ini_set('display_errors', 0); error_reporting(E_ALL);
3.2 Use custom error handling functions:
function customError($errno, $errstr, $errfile, $errline) { // 处理错误的代码 } set_error_handler("customError");
4. Pay attention to password security
Password security is an important issue that any web application must pay attention to. Here are some best practices for password security:
4.1 Use secure hashing algorithms:
$password = password_hash($_POST['password'], PASSWORD_DEFAULT);
4.2 Set password policy requirements:
$minimum_password_length = 8; $require_uppercase = true; $require_lowercase = true; $require_numbers = true;
5. Use the latest PHP version
Using the latest PHP version ensures that you are using the latest features, bug fixes, and security patches. At the same time, updating the PHP version can also improve performance and stability.
Conclusion:
In PHP development, it is very important to avoid common mistakes. These errors can be effectively avoided by adopting some best practices, such as preventing SQL injection attacks, preventing XSS attacks, handling errors correctly, paying attention to password security, and updating PHP versions. I hope the code examples and practical suggestions in this article can help you better avoid common mistakes in PHP development.
The above is the detailed content of How to avoid common mistakes in PHP development. For more information, please follow other related articles on the PHP Chinese website!
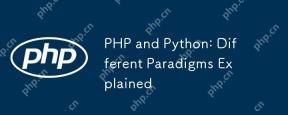
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
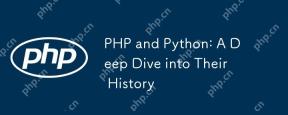
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
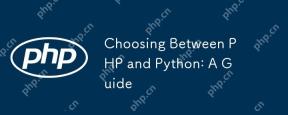
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
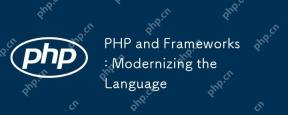
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
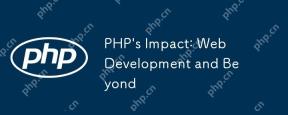
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
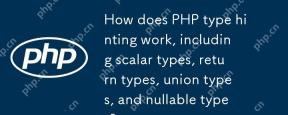
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
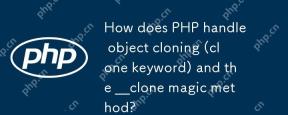
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
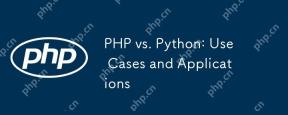
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
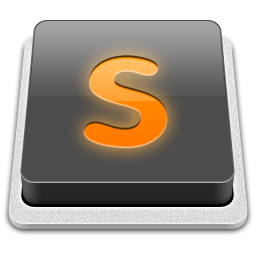
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor