


Print all balanced bracket strings formed by replacing the wildcard character '?'
Balanced brackets means that if we have a string of brackets, then each open bracket has a corresponding closing bracket, and the bracket pairs are nested correctly. The size of the string should be an even number. In this problem, we are given a bracket string containing the character '?' and our task is to form every possible balanced bracket string by replacing '?' with the appropriate bracket. In our given string, only parentheses '(' and ')' are used.
ExampleExample
Input 1: str = “()(?)?” Output 1: ()(())The Chinese translation of
Explanation
is:Explanation
Only a balanced string can be formed by replacing '?'.
Input 2: str = “??????”
Output 2: ((())) (()()) (())() ()(()) ()()()The Chinese translation of
Explanation
is:Explanation
There are two possible ways to form a balanced string.
One way is to replace indices 0, 1 and 2 with an open bracket and the other indices with a closed bracket.
The second method is to replace indices 0, 1, and 3 with an open bracket, and the other indices with a closed bracket.
The third method is to replace indexes 0, 1 and 4 with open brackets and the other indices with closed brackets.
The fourth method is to replace the positions at index 0, 2 and 3 with an open bracket and replace the positions at other indexes with a closed bracket.
The last way is to replace indexes 0, 2 and 4 with open brackets and the other indexes with closed brackets.
method
We have seen the example of the given string above, let’s move on to the next step -
We can use the backtracking method to solve this problem.
Let us discuss this method below -
First, we will initialize a function called 'create' to create all possible strings after replacing '?' with brackets, with parameters str and index = 0.
In this function,
Initialize the 'check' function to verify that the string is balanced.
−> First, we set the basic conditions. If we reach the end of the string, then we must pass the string to the "check" function to verify that the string is balanced. If it is balanced, print the string.
−>If the current character of the string is ‘?’,
First, replace it with an open bracket and call the same function to check if the end of the string is reached.
Secondly, replace it with the closing bracket and call the same function again to check if we have reached the end of the string.
Finally, we backtrack the string and assign the current character to ‘?’
−> Otherwise, if the current character of the string is a bracket, move to the next index by calling the same function.
−> In this function, we initialize the stack and then check
−> If the first character of the string is a closing bracket, return false
−> If the current bracket is closed, there are two situations: if the stack is empty, false is returned because there is no corresponding open bracket. Otherwise, pop the corresponding open bracket from the stack.
−> Finally, we check whether the stack is empty. If it is empty, it means the string is balanced and returns true. Otherwise, there are some brackets remaining, which means the string is unbalanced and returns false.
Example
is:Example
The following is the C code used for the above backtracking method to obtain all balanced strings
#include <bits/stdc++.h> using namespace std; // Function 'check' to verify whether the string is balanced or not bool check(string str){ stack<char> S; // created stack // If the first character of the string is a close bracket, then return false if (str[0] == ')') { return false; } // Traverse the string using for loop for (int i = 0; i < str.size(); i++) { // If the current character is an open bracket, then push it into the stack if (str[i] == '(') { S.push('('); } // If the current character is a close bracket else { // If the stack is empty, there is no corresponding open bracket return false if (S.empty()){ return false; } // Else pop the corresponding opening bracket from the stack else S.pop(); } } // If the stack is empty, return true if (S.empty()){ return true; } else { return false; } } // Function 'create' to create all possible bracket strings void create(string str, int i){ // If reached the end of the string if (i == str.size()) { // passed 'str' to the 'check' function to verify whether the string is balanced or not if (check(str)) { // If it is a balanced string cout<< str << endl; // print the string } return; } // If the current character of the string is '?' if (str[i] == '?') { str[i] = '('; // replace ? with ( create(str, i + 1); // continue to next character str[i] = ')'; // replace ? with ) create(str, i + 1); // continue to next character // backtrack str[i] = '?'; } // If the current character is bracketed then move to the next index else { create(str, i + 1); } } int main(){ string str = "??????"; //given string // Call the function create (str, 0); return 0; }
Output
((())) (()()) (())() ()(()) ()()()
Time complexity and space complexity
The time complexity of the above code is O(N*(2^N)) because we need to backtrack on the string.
The space complexity of the above code is O(N) because we store the brackets on the stack.
Where N is the size of the string.
in conclusion
In this tutorial, we implemented a program that prints all balanced bracket strings that can be formed by replacing the wildcard character '?'. We implemented a backtracking method. The time complexity is O(N*(2^N), and the space complexity is O(N). Where N is the size of the string.
The above is the detailed content of Print all balanced bracket strings formed by replacing the wildcard character '?'. For more information, please follow other related articles on the PHP Chinese website!
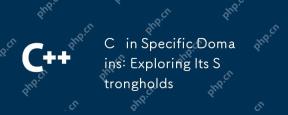
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
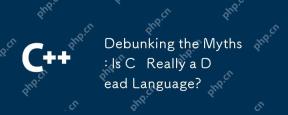
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
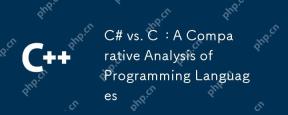
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
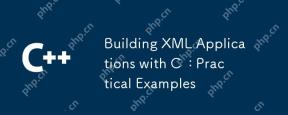
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
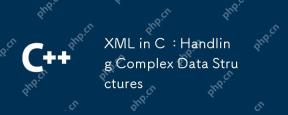
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
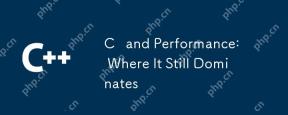
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
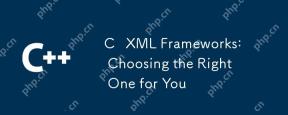
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
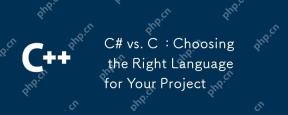
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
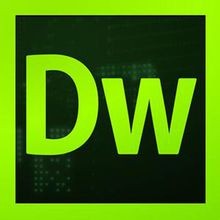
Dreamweaver CS6
Visual web development tools
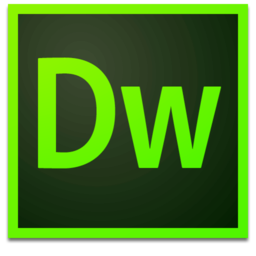
Dreamweaver Mac version
Visual web development tools
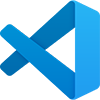
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
