


Building a full-stack application: Detailed explanation of Vue3+Django4 project development
Building a full-stack application: Detailed explanation of Vue3 Django4 project development
1. Introduction
With the rapid development of the Internet, full-stack development has received more and more attention. Full-stack developers can be responsible for both front-end and back-end development work, thereby improving development efficiency and the overall quality of the project. This article will introduce in detail how to build a full-stack application, and use Vue3 and Django4 as the development framework to explain.
2. Technical Overview
Before building a full-stack application, we need to understand some key technical concepts. Vue3 is a simple, flexible and efficient JavaScript framework that can be used to build modern web applications. Django4 is a powerful and easy-to-use Python web framework for quickly developing secure and reliable web applications.
3. Build the front-end
- Environment preparation
First, you need to install Node.js and npm package manager. After the installation is complete, use the following command to check the version:
node -v npm -v
- Create Vue project
Enter the following command on the command line to create a new Vue project:
vue create my-vue-app
Select some basic settings according to the prompts, such as project name, project configuration, etc.
- Install Vue Router and Vuex
Use the following command to install Vue Router and Vuex in the Vue project:
cd my-vue-app npm install vue-router vuex
- Create component
In src Create a folder named components under the folder, then create a file named HelloWorld.vue in it, and add the following code:
<template> <div> <h1 id="msg">{{ msg }}</h1> </div> </template> <script> export default { data() { return { msg: "Hello, World!" }; } }; </script> <style scoped> h1 { color: blue; } </style>
- Configure routing
In src Create a folder named router under the folder, then create a file named index.js in it, and add the following code:
import { createRouter, createWebHistory } from "vue-router"; import HelloWorld from "../components/HelloWorld.vue"; const routes = [ { path: "/", name: "HelloWorld", component: HelloWorld } ]; const router = createRouter({ history: createWebHistory(), routes }); export default router;
- Configuration status management
In Create a folder named store under the src folder, then create a file named index.js in it, and add the following code:
import { createStore } from "vuex"; export default createStore({ state() { return { count: 0 }; }, mutations: { increment(state) { state.count++; } } });
- Introduce components into the main application , routing and status management
Add the following code in the main.js file under the src folder:
import { createApp } from "vue"; import App from "./App.vue"; import router from "./router"; import store from "./store"; createApp(App) .use(router) .use(store) .mount("#app");
4. Build the backend
- Environment preparation
First, you need to install Python and the pip package manager. After the installation is complete, use the following command to check the version:
python -V pip -V
- Create a Django project
Enter the following instructions on the command line to create a new Django project:
django-admin startproject mydjangoapp
- Create Django application
Enter the command line in the project root directory and enter the following instructions to create an application named mydjangoapp:
cd mydjangoapp ./manage.py startapp myapp
- Configuration database
Configure database connection parameters in the settings.py file:
DATABASES = { "default": { "ENGINE": "django.db.backends.sqlite3", "NAME": BASE_DIR / "db.sqlite3", } }
- Write API views
Add the following code in the views.py file under the myapp folder:
from django.http import JsonResponse def hello_world(request): return JsonResponse({"message": "Hello, World!"})
- Configure routing
Add the following code in the urls.py file under the mydjangoapp folder:
from django.urls import path from myapp.views import hello_world urlpatterns = [ path("api/hello", hello_world), ]
- Start the Django development server
Run the following command in the project root directory to start the Django development server:
./manage.py runserver
5. Front-end and back-end joint debugging
- Create proxy configuration
At the root of the Vue project Create a file named vue.config.js in the directory and add the following code:
module.exports = { devServer: { proxy: { "/api": { target: "http://localhost:8000", ws: true, changeOrigin: true } } } };
- Call API
Add the following code in the HelloWorld.vue component:
<template> <div> <h1 id="msg">{{ msg }}</h1> <h2 id="Count-count">Count: {{ count }}</h2> <button @click="increment">Increment</button> </div> </template> <script> export default { data() { return { msg: "", count: 0 }; }, created() { fetch("/api/hello") .then(response => response.json()) .then(data => { this.msg = data.message; }); }, methods: { increment() { this.$store.commit("increment"); } }, computed: { count() { return this.$store.state.count; } } }; </script>
6. Run the project
Enter the Vue project root directory in the command line, and run the following instructions to start the front-end development server:
npm run serve
Enter the Django project in another command line window In the root directory, run the following command to start the back-end development server:
./manage.py runserver
Now, open the browser and visit http://localhost:8080, you will see a message containing "Hello, World!" and "Count: 0" page. Click the "Increment" button and "Count" will automatically increase by 1.
7. Summary
Through the detailed introduction of this article, we have learned how to build a full-stack application, using Vue3 as the front-end framework and Django4 as the back-end framework, and demonstrated the joint debugging of the front and rear ends through code examples. process. Full-stack development is of great significance to improving development efficiency and project quality. I hope this article will be helpful to you. I wish you greater success on the road to full-stack development!
The above is the detailed content of Building a full-stack application: Detailed explanation of Vue3+Django4 project development. For more information, please follow other related articles on the PHP Chinese website!

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
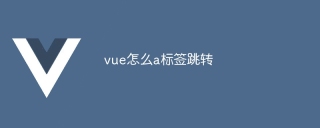
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
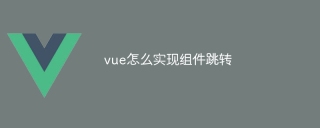
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
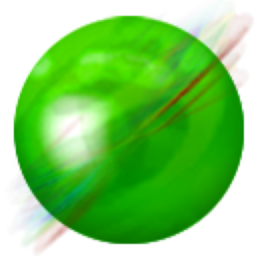
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
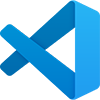
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
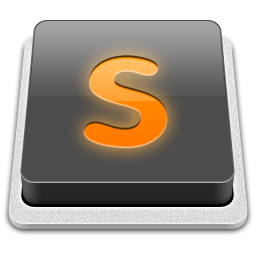
SublimeText3 Mac version
God-level code editing software (SublimeText3)
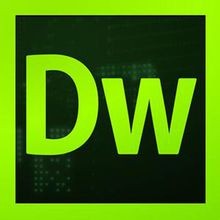
Dreamweaver CS6
Visual web development tools