By using arrays and data structures, it is possible to store homogeneous (identical) data in multiple memory locations. The key benefit of using arrays is that we can retrieve them from any position using the index parameter. This data structure becomes linear because data must be inserted and extracted step by step. We just need to put the index or position number of the element inside square brackets to retrieve it from the array. In this article, we will use an array A and another element e. We will insert e in C at the starting position of A.
Understand concepts and illustrate with examples
Given array A = [10, 14, 65, 85, 96, 12, 35, 74, 69] After inserting 23 at the end, the array will look like this: [23, 10, 14, 65, 85, 96, 12, 35, 74, 69]
In the above example, we have an array A containing nine elements. We will insert another element 23 at the beginning of array A. The resulting array contains all elements plus the leading 23. To insert an element at the beginning we have to move all elements one position to the right, then the first slot will be empty and we put the new element in that position. Let’s take a look at the algorithm for a clearer understanding.
algorithm
Take an array A and an element e
-
If array A has enough space to insert element e, then
-
For i in the range from n-1 to 0, do the following:
A[ i 1 ] = A[ i ]
End loop
A[0]=e
Increase n 1
-
End if
Return A
Example
is:Example
#include <iostream> # define Z 50 using namespace std; void displayArr(int arr[], int n){ for( int i = 0; i < n; i++ ){ cout << arr[ i ] << ", "; } cout << endl; } void insertAtBeginning( int A[], int &n, int e ){ if( n + 1 < Z ) { for( int i = n - 1; i >= 0; i-- ) { A[ i + 1 ] = A[ i ]; } A[ 0 ] = e; n = n + 1; } } int main() { int A[ Z ] = {57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14}; int n = 12; cout << "Array before insertion: "; displayArr( A, n ); cout << "Inserting 58 at the beginning:" << endl; insertAtBeginning( A, n, 58 ); cout << "Array after insertion: "; displayArr( A, n ); cout << "Inserting 225 at the beginning:" << endl; insertAtBeginning( A, n, 225 ); cout << "Array after insertion: "; displayArr( A, n ); }
Output
Array before insertion: 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Inserting 58 at the beginning: Array after insertion: 58, 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Inserting 225 at the beginning: Array after insertion: 225, 58, 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14,
Use a vector to insert an element in front
Vector is a dynamic data structure that is part of the C STL. We can get similar functionality to arrays in vectors. But in vector we can insert only at the end or behind. There is no direct way to insert at the beginning. However, we can move the element one position back as before and insert the new element at the beginning. Or we can create another one-element vector containing only the new elements and then concatenate them. Therefore, the resulting vector will contain all previous elements and the new element at the beginning. Let's look at the algorithm and C implementation.
algorithm
Take an array A and an element e
Create an empty vector B
Insert e into B
A := Connect B and A (first B and then A)
Return A
Example
is:Example
#include <iostream> #include <vector> # define Z 50 using namespace std; void displayArr( vector<int> v ){ for( int i = 0; i < v.size() ; i++ ){ cout << v[ i ] << ", "; } cout << endl; } vector<int> insertAtBeginning( vector<int> A, int e ){ vector<int> B( 1 ); B[ 0 ] = e; B.insert( B.end(), A.begin(), A.end() ); return B; } int main() { vector<int> A = {57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14}; cout << "Array before insertion: "; displayArr( A ); cout << "Inserting 58 at the beginning:" << endl; A = insertAtBeginning( A, 58 ); cout << "Array after insertion: "; displayArr( A ); cout << "Inserting 225 at the beginning:" << endl; A = insertAtBeginning( A, 225 ); cout << "Array after insertion: "; displayArr( A ); }
Output
Array before insertion: 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Inserting 58 at the beginning: Array after insertion: 58, 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Inserting 225 at the beginning: Array after insertion: 225, 58, 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14,
in conclusion
In this article, we have seen how to insert elements at the beginning of an array. Here we discuss two different solutions. The first solution uses static C arrays, while the second solution uses vectors. Vectors have no way to insert elements directly at the beginning. We can insert elements directly at the end using the push_back() method. To do this, we use a trick where we create an array of size 1 and insert the new element into it. Then concatenate it with the given array. We can achieve the same effect using lists. However, C lists have push_front() method, which can insert elements directly in front. But lists are not arrays, they are linked lists.
The above is the detailed content of C++ program to add element to beginning of array. For more information, please follow other related articles on the PHP Chinese website!
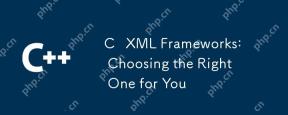
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
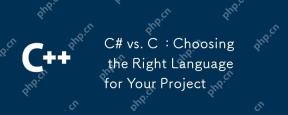
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
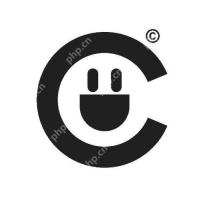
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
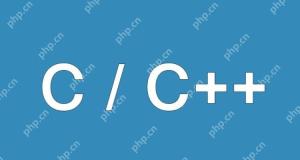
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
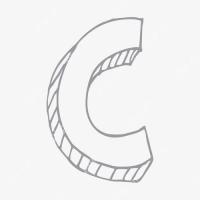
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
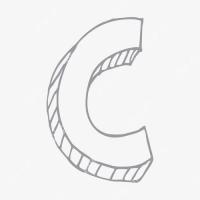
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
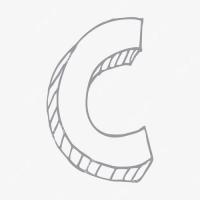
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
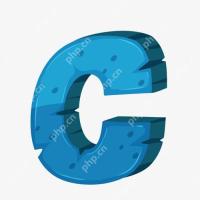
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
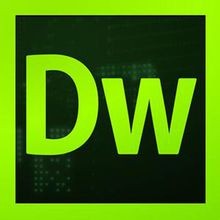
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
