


Checks if a string contains consecutive letters and each letter appears only once
Introduction
In C, a string is a series of characters, which can be different or repeated. Consecutive characters are characters that appear at the same time, and the difference between them is 1. For example, the characters a and b are consecutive because they appear together. However, the characters m and o have a difference of 2 in their positions, making them not consecutive.
In this article, we will develop a code that will take a string as input and display true when all characters in the string are contiguous. Let us see the following example to understand this topic better
Sample Example
Example 1 - str - "pqsr"
Output - Yes
In this article, we will develop a code to extract the current and the previous character from the string. It is then further checked if the characters differ by position non-equivalent to 1, then the boolean false value is returned.
Syntax
The translation ofsort()
is:sort()
sort(str.begin(), str.end())
The sort() method in C is used to arrange the characters in a string in increasing order from the beginning to the end.
parameter
str - The input string
end - the last character
occurring in the stringbegin-The first character appearing in the string
length()
is translated as:length()
The length() method in C is used to compute the number of characters in the string.
str.length()
parameter
str - The input string
algorithm
Accepts an input string, str as input.
The input string is sorted using the sort() method.
An iteration of the string is performed, using the for loop i.
The length of the string is computed using the length() method and stored in len variable.
Perform for loop iteration on the string, i is the iteration performed.
Extract the characters at the ith, ch and i-1th, ch1 positions each time.
If the difference between these two characters is not equal to 1, then a boolean false value is returned
If all the corresponding characters satisfy the required condition, then the boolean value - true is returned.
This value is returned as a Boolean flag and stored in the variable res. If its value is true, a string containing consecutive characters is printed.
Example
The following C code snippet is used to input a sample string and calculate whether all characters appearing in the string are consecutive.
//including the required libraries #include <bits/stdc++.h> using namespace std; //function to check of characters consecutive bool validateString(string str) { //length of the string int len = str.length(); // sorting the given string sort(str.begin(), str.end()); // Iterate for every index and // check for the condition for (int i = 1; i < len; i++) { //extract characters at the required pos char ch = str[i]; char ch1 = str[i-1]; if (ch-ch1 != 1) //in case characters are not consecutive return false; } //if condition holds return true; } //calling the main method int main() { // 1st example string str = "mpon"; cout << "Input String : " <<str << " \n"; bool res = validateString(str); if (res) cout << "Yes, the string contains only consecutive characters\n"; else cout << "No, the string doesn't contain only consecutive characters.\n"; return 0; }
Output
Input String − mpon Yes, the string contains only consecutive characters
Conclusion
Characters that appear continuously in a string are letters that appear at the same time. This can be achieved by sorting the string from start to end. Characters in consecutive positions can be easily compared and checked how many positions differ between them. This can be used to capture information about whether a string is continuous.
The above is the detailed content of Checks if a string contains consecutive letters and each letter appears only once. For more information, please follow other related articles on the PHP Chinese website!
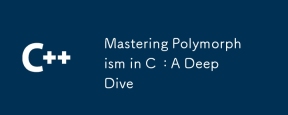
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
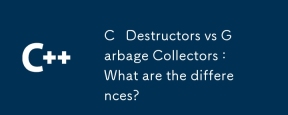
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
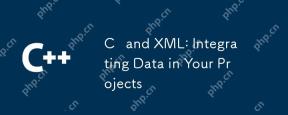
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
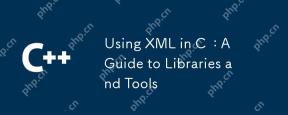
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
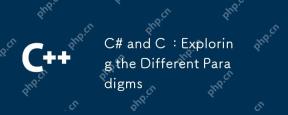
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
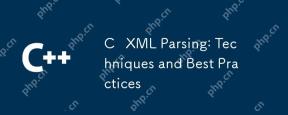
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
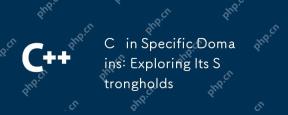
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
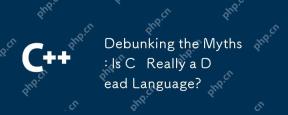
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
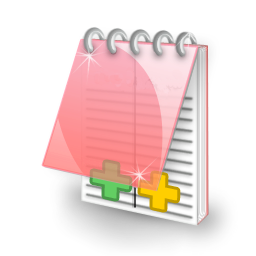
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
