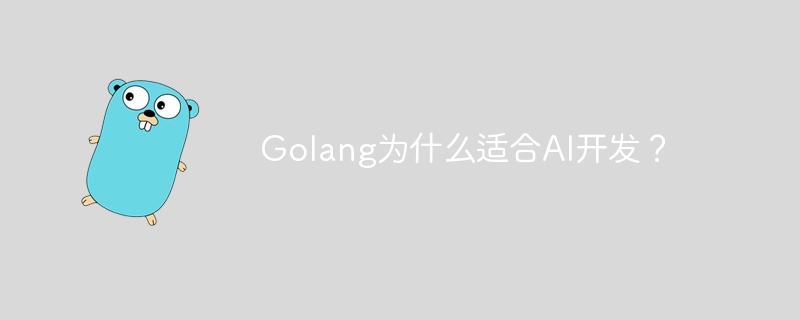
Why is Golang suitable for AI development?
With the rapid development of artificial intelligence (AI) technology, more and more developers and researchers have begun to pay attention to the potential of using the Golang programming language in the field of AI. Golang (also known as Go) is an open source programming language developed by Google. It is loved by developers for its high performance, high concurrency and simplicity and ease of use. This article will explore why Golang is suitable for AI development and provide some sample code to demonstrate Golang's advantages in the AI field.
- High performance and concurrency:
Golang is an ideal language for writing high-performance applications. Its concurrency model is implemented using goroutine and channel, and has lightweight and efficient concurrent processing capabilities. This makes Golang ideal for handling massively parallel tasks, such as during AI training and inference. The following is a simple sample code that illustrates how to use goroutine and channel to process tasks concurrently:
package main
import (
"fmt"
"time"
)
func worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Printf("Worker %d started job %d
", id, j)
time.Sleep(time.Second)
fmt.Printf("Worker %d finished job %d
", id, j)
results <- j * 2
}
}
func main() {
jobs := make(chan int, 5)
results := make(chan int, 5)
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
for j := 1; j <= 5; j++ {
jobs <- j
}
close(jobs)
for a := 1; a <= 5; a++ {
<-results
}
}
In this example, we create 3 goroutines as workers and use channels to deliver tasks and results. Each worker processes the received tasks in parallel and sends the processed results to the result channel.
- Rich standard library and third-party libraries:
Golang has a rich standard library and active development community, which allows developers to easily use various AI-related functions and algorithms. For example, Golang's standard library contains powerful numerical calculation libraries, such as math and math/rand, which can be used for the implementation of AI algorithms. In addition, Golang has many third-party libraries that can be used for the development of AI tasks such as machine learning, natural language processing, and image processing, such as Gorgonia, Golearn, and GoCV. These libraries provide many already implemented and optimized algorithms and tools, greatly reducing the workload of AI development.
- Cross-platform and easy deployment:
Golang’s compiler can convert source code into machine code, so that Golang applications can run in different operating systems and architectures. This is especially important for AI development, because AI systems often need to run on different platforms, such as cloud servers, mobile devices, or embedded systems. The deployment of Golang is also very convenient. You only need to copy the compiled executable file to the target machine without worrying about dependencies and environment configuration.
Although Golang has not yet become as popular as Python or R in the AI field, its excellent performance, concurrency capabilities and rich library support make it a potential candidate. With the development and support of Golang in the AI community, I believe more and more developers will choose to use Golang for AI development.
Reference link:
- https://tour.golang.org/concurrency/1
- https://github.com/golang/go/wiki /Projects#machine-learning
The above is the detailed content of Why is Golang suitable for AI development?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn