


Analysis of the underlying development principles of PHP8 new features and their application examples
Analysis of the underlying development principles of PHP8 new features and their application examples
Abstract: PHP is a widely used server-side scripting language that runs on Web servers. PHP 8 is the latest version and introduces many exciting new features and improvements. This article will explore the underlying development principles of PHP8 and provide some practical application examples.
- JIT compiler
Just-In-Time (just-in-time compilation) is an important feature in PHP 8. JIT is a dynamic compilation technology that can compile hot code into local machine code while the program is running. This compilation method improves the execution speed of PHP scripts, especially in loops and intensive calculations.
Here is a sample code using the JIT compiler:
// 开启JIT编译器 opcache_compile_file('path/to/file.php'); // 调用被编译的函数 myFunction();
- New type system
PHP 8 introduces a new type system, including static Types and strong typing. Static typing enables type errors to be detected during the compilation phase, improving the reliability and maintainability of the code. Strong typing means that only variables of the same type can be operated on, reducing bugs caused by type errors.
The following is a sample code using the new type system:
// 声明变量类型 int $num = 5; string $name = "John"; // 类型检查和转换 if (is_int($num)) { $result = $num * 2; } // 类型错误的示例 if ($num + $name) { // 这里会产生一个错误 }
- Improvements in property definition
PHP 8 has improved property definition and increased access Modifiers and type declarations. This makes property access more secure and reliable.
The following is a sample code using the improved property definition:
class Person { public string $name; protected int $age; private array $languages; public function __construct(string $name, int $age, array $languages) { $this->name = $name; $this->age = $age; $this->languages = $languages; } public function getName(): string { return $this->name; } public function getAge(): int { return $this->age; } public function getLanguages(): array { return $this->languages; } } // 创建Person对象 $person = new Person("John", 30, ["English", "Spanish"]); // 访问属性 echo $person->getName(); // 输出 "John" echo $person->getAge(); // 输出 30 echo implode(", ", $person->getLanguages()); // 输出 "English, Spanish"
- Other improvements
In addition to the above features, PHP 8 also introduces some other improvements , including improvements to error and exception handling, improvements to anonymous functions, and improvements to native data structures.
Here is sample code with some other improvements:
// 错误和异常处理 try { // 一些可能会抛出异常的代码 } catch (Exception $e) { // 异常处理逻辑 } // 匿名函数的改进 $func = fn($value) => $value * 2; // 对原生数据结构的改进 $array = [1, 2, 3, 4, 5]; // 使用新的array_map函数 $newArray = array_map(fn($value) => $value * 2, $array);
Conclusion:
PHP 8’s new features and improvements bring many benefits to developers, including faster Execution speed, more reliable code, and more powerful language features. Understanding and applying these features can improve development efficiency and code quality. The above are some analysis and practical application examples of the underlying development principles of PHP 8. I hope it will be helpful to readers.
The above is the detailed content of Analysis of the underlying development principles of PHP8 new features and their application examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
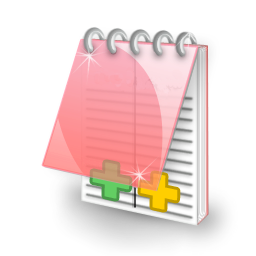
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use
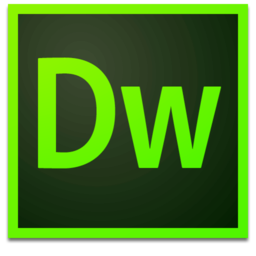
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
