C++ program to calculate the sum of diagonals of a matrix
The utilization of 2-dimensional arrays or matrices is extremely advantageous for several applications. Matrix rows and columns are used to hold numbers. We can define 2D Multidimensional arrays are used to represent matrices in C. In this article we will look at how to implement use C to calculate the diagonal sum of a given square matrix.
The matrices have two diagonals, the main diagonal and the secondary diagonal (sometimes referred to as major and minor diagonals). The major diagonal starts from the top-left corner (index [0, 0]) to the bottom-right corner (index [n-1, n-1]) where n is the order of the Square matrix. The main diagonal starts from the upper right corner (index [n-1, 0]) and ends at the lower left corner corner (index [0, n-1]). Let us see the algorithm to find the sum of the elements along with these two diagonals.
The Chinese translation ofMatrix Diagonal Sum
is:The sum of the matrix diagonals
$$\begin{bmatrix} 8 & 5& 3\newline 6 & 7& 1\newline 2 & 4& 9\ \end{bmatrix},$$
Sum of all elements in major diagonal: (8 + 7 + 9) = 24 Sum of all elements in minor diagonal: (3 + 7 + 2) = 12
In the previous example, one 3 x 3 matrix was used. We have scanned the diagonals individually and calculated the sum. Let us see the algorithm and implementation for a clear view.
Algorithm
- Read matrix M as input
- Consider M with n rows and n columns
- sum_major := 0
- sum_minor := 0
- For the range of i from 0 to n-1, execute
- for j range from 0 to n - 1, do
- if i and j are the same, then
- sum_major := sum_major M[ i ][ j ]
- end if
- if (i j) is same as (N - 1), then
- sum_minor := sum_minor M[ i ][ j ]
- end if
- if i and j are the same, then
- end for
- end for
- return sum
Example
#include <iostream> #include <cmath> #define N 7 using namespace std; float solve( int M[ N ][ N ] ){ int sum_major = 0; int sum_minor = 0; for ( int i = 0; i < N; i++ ) { for ( int j = 0; j < N; j++ ) { if( i == j ) { sum_major = sum_major + M[ i ][ j ]; } if( (i + j) == N - 1) { sum_minor = sum_minor + M[ i ][ j ]; } } } cout << "The sum of major diagonal: " << sum_major << endl; cout << "The sum of minor diagonal: " << sum_minor << endl; } int main(){ int mat1[ N ][ N ] = { {5, 8, 74, 21, 69, 78, 25}, {48, 2, 98, 6, 63, 52, 3}, {85, 12, 10, 6, 9, 47, 21}, {6, 12, 18, 32, 5, 10, 32}, {8, 45, 74, 69, 1, 14, 56}, {7, 69, 17, 25, 89, 23, 47}, {98, 23, 15, 20, 63, 21, 56}, }; cout << "For the first matrix: " << endl; solve( mat1 ); int mat2[ N ][ N ] = { {6, 8, 35, 21, 87, 8, 26}, {99, 2, 36, 326, 25, 24, 56}, {15, 215, 3, 157, 8, 41, 23}, {96, 115, 17, 5, 3, 10, 18}, {56, 4, 78, 5, 10, 22, 58}, {85, 41, 29, 65, 47, 36, 78}, {12, 23, 87, 45, 69, 96, 12} }; cout << "\nFor the second matrix: " << endl; solve( mat2 ); }
Output
For the first matrix: The sum of major diagonal: 129 The sum of minor diagonal: 359 For the second matrix: The sum of major diagonal: 74 The sum of minor diagonal: 194
Conclusion
In this article, we have seen how to calculate the diagonal sums of a given square matrix. The main diagonal runs from the upper left corner to the lower right corner, while the secondary diagonal runs from the lower left corner to the upper right corner. The diagonal line starts from the upper right corner to the lower left corner. To find the sum of these diagonal elements, we loop through all elements. When both row and column index values Same, it represents the main diagonal element when the sum of the two indices is Same as the order n-1 of the matrix, it will be added to the subdiagonal procedure takes two nested loops and we are traversing through all elements present in the 2D array. Therefore, calculating the sum of the two diagonals will take O(n2) time given matrix.
The above is the detailed content of C++ program to calculate the sum of diagonals of a matrix. For more information, please follow other related articles on the PHP Chinese website!
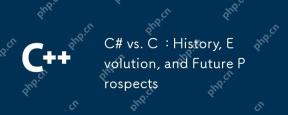
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
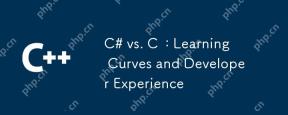
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
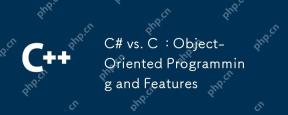
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
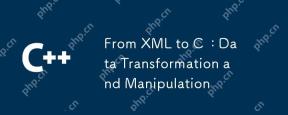
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
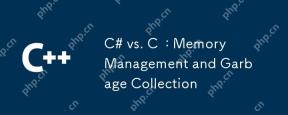
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
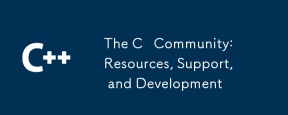
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
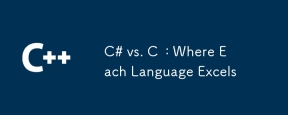
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
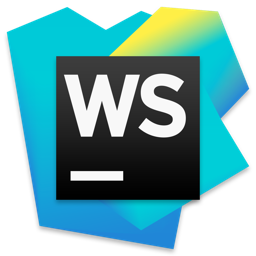
WebStorm Mac version
Useful JavaScript development tools
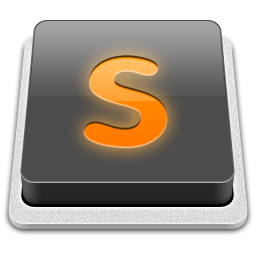
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.