


Minimum number of swaps between two strings such that one string is strictly greater than the other
In this article, we will discuss an interesting string manipulation problem - "The minimum number of exchanges required between two strings such that one string is strictly larger than the other string". We'll look at the problem, detail strategies for solving it, implement it in C, and clarify concepts with a relevant example.
Understanding the problem statement
Given two strings of equal length, our goal is to determine the minimum number of character swaps required to make one string strictly larger than the other. Characters are swapped between the two strings, with each swap involving a character from both strings. Strings are compared lexicographically, where 'a'
method
The idea is to use a greedy algorithm. We start from the beginning of the string, and for each position, if the character in the first string is smaller than the corresponding character in the second string, we swap them. If they are equal, we look for the larger character in the second string to swap. If no such character is found, we continue to the next position. We repeat this process until all characters in the string have been processed.
Example
Let's implement this method in C -
#include<bits/stdc++.h> using namespace std; int minSwaps(string &s1, string &s2) { int swaps = 0; int n = s1.size(); for(int i=0; i<n; i++) { if(s1[i] < s2[i]) { swap(s1[i], s2[i]); swaps++; } else if(s1[i] == s2[i]) { for(int j=i+1; j<n; j++) { if(s2[j] > s1[i]) { swap(s1[i], s2[j]); swaps++; break; } } } } return (s1 > s2) ? swaps : -1; } int main() { string s1 = "bbca"; string s2 = "abbc"; int swaps = minSwaps(s1, s2); if(swaps != -1) cout << "Minimum swaps: " << swaps << "\n"; else cout << "Cannot make string 1 greater\n"; return 0; }
Output
Minimum swaps: 2
Test Case
Let us consider the strings "bbca" and "abbc". The following exchange will occur −
Exchange 'b' in the first string with 'a' in the second string. The strings are now "bbac" and "abbc".
Exchange "c" in the first string with "b" in the second string. The strings now are "bbcb" and "abac".
"bbcb" is lexicographically greater than "abac". Therefore, the minimum number of swaps required is 2, and the output of the program will be "Minimum number of swaps: 2".
in conclusion
In this article, we explore the problem of determining the minimum number of swaps required between two strings so that one string is lexicographically larger than the other. We discuss strategies for solving the problem, implement it in C, and explain the concept with examples. String manipulation questions like this are common in interviews and competitive programming, and understanding these concepts is very beneficial.
The above is the detailed content of Minimum number of swaps between two strings such that one string is strictly greater than the other. For more information, please follow other related articles on the PHP Chinese website!
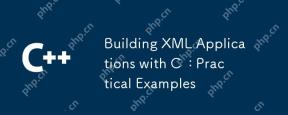
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
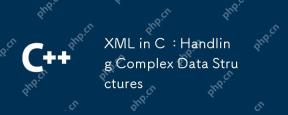
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
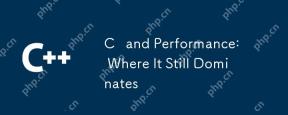
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
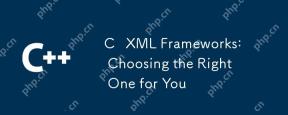
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
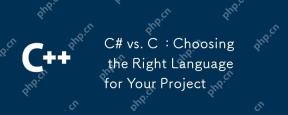
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
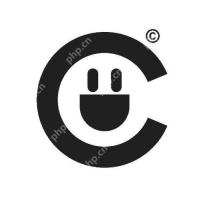
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
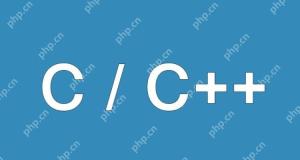
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
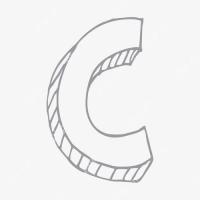
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
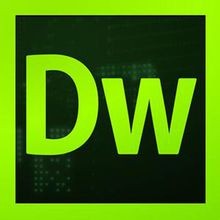
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
