


How to achieve better code abstraction through forced inheritance of proxy final classes in Java programming?
How to achieve better code abstraction by forcing inheritance of the proxy final class in Java programming?
Introduction:
In Java programming, we often face situations where we need to extend existing classes. However, sometimes the classes we encounter are declared final, that is, they cannot be inherited. So, how to achieve code abstraction and reuse in this case? This article will introduce a method of delegating final classes through forced inheritance to achieve better code abstraction while maintaining code security.
- Restrictions on final classes
In Java, the final keyword can be used to modify classes, methods, and variables. When a class is declared final, it means that the class cannot be inherited. Such a design is usually to protect the integrity and security of the class and prevent other classes from modifying or extending it.
However, sometimes we need to extend such final classes to implement more functions or adapt to different needs. In this case, we can use forced inheritance to proxy the final class method.
- How to implement forced inheritance of the agent final class
To implement the method of forced inheritance of the agent final class, we need to follow the following steps:
Step 1: Define a Interface
First, we need to define an interface that contains the functionality we need to extend the final class. The methods declared in the interface will serve as abstractions for the proxy class methods.
public interface FinalClassProxy { void doSomething(); }
Step 2: Create a proxy class (Proxy Class)
Then, we create a proxy class that implements the interface defined in step 1 and uses the final class as a member variable.
public class FinalClassProxyImpl implements FinalClassProxy { private final FinalClass finalClass; public FinalClassProxyImpl(FinalClass finalClass) { this.finalClass = finalClass; } @Override public void doSomething() { // 执行代理操作 System.out.println("执行代理操作"); // 调用final类的方法 finalClass.doSomething(); } }
Step 3: Use the proxy class
Now, we can use the proxy class to extend the final class.
public class Main { public static void main(String[] args) { FinalClass finalClass = new FinalClass(); FinalClassProxy proxy = new FinalClassProxyImpl(finalClass); proxy.doSomething(); } }
- Code example description
In the above code example, we first define an interface FinalClassProxy, which declares a method doSomething(). Next, we created a proxy class FinalClassProxyImpl, which implements the FinalClassProxy interface and uses the final class FinalClass as a member variable.
In the doSomething() method of the proxy class, we first perform some proxy operations, and then call the doSomething() method of the final class. In this way, we indirectly called the method of the final class through the proxy class and successfully extended the class.
Finally, create an instance of the final class and an instance of the proxy class in the main program, and call the method of the final class through the method of the proxy class.
- Summary
By forcing inheritance to proxy the final class method, we can extend the final class and achieve better code abstraction while maintaining code security and integrity. This method is very useful when the final class needs to be extended, and can effectively solve the problem of extending the functionality of the final class when it cannot inherit it.
The above is the detailed content of How to achieve better code abstraction through forced inheritance of proxy final classes in Java programming?. For more information, please follow other related articles on the PHP Chinese website!
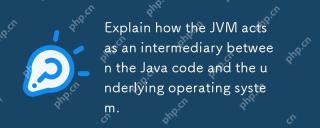
JVM works by converting Java code into machine code and managing resources. 1) Class loading: Load the .class file into memory. 2) Runtime data area: manage memory area. 3) Execution engine: interpret or compile execution bytecode. 4) Local method interface: interact with the operating system through JNI.

JVM enables Java to run across platforms. 1) JVM loads, validates and executes bytecode. 2) JVM's work includes class loading, bytecode verification, interpretation execution and memory management. 3) JVM supports advanced features such as dynamic class loading and reflection.

Java applications can run on different operating systems through the following steps: 1) Use File or Paths class to process file paths; 2) Set and obtain environment variables through System.getenv(); 3) Use Maven or Gradle to manage dependencies and test. Java's cross-platform capabilities rely on the JVM's abstraction layer, but still require manual handling of certain operating system-specific features.
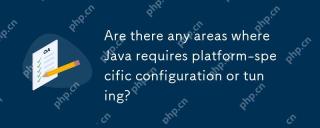
Java requires specific configuration and tuning on different platforms. 1) Adjust JVM parameters, such as -Xms and -Xmx to set the heap size. 2) Choose the appropriate garbage collection strategy, such as ParallelGC or G1GC. 3) Configure the Native library to adapt to different platforms. These measures can enable Java applications to perform best in various environments.
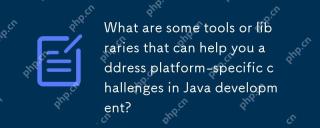
OSGi,ApacheCommonsLang,JNA,andJVMoptionsareeffectiveforhandlingplatform-specificchallengesinJava.1)OSGimanagesdependenciesandisolatescomponents.2)ApacheCommonsLangprovidesutilityfunctions.3)JNAallowscallingnativecode.4)JVMoptionstweakapplicationbehav
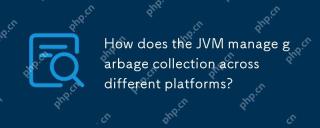
JVMmanagesgarbagecollectionacrossplatformseffectivelybyusingagenerationalapproachandadaptingtoOSandhardwaredifferences.ItemploysvariouscollectorslikeSerial,Parallel,CMS,andG1,eachsuitedfordifferentscenarios.Performancecanbetunedwithflagslike-XX:NewRa
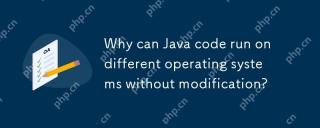
Java code can run on different operating systems without modification, because Java's "write once, run everywhere" philosophy is implemented by Java virtual machine (JVM). As the intermediary between the compiled Java bytecode and the operating system, the JVM translates the bytecode into specific machine instructions to ensure that the program can run independently on any platform with JVM installed.
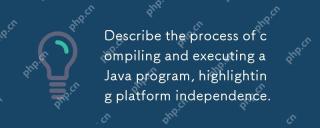
The compilation and execution of Java programs achieve platform independence through bytecode and JVM. 1) Write Java source code and compile it into bytecode. 2) Use JVM to execute bytecode on any platform to ensure the code runs across platforms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
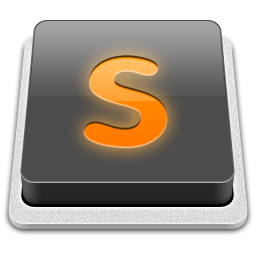
SublimeText3 Mac version
God-level code editing software (SublimeText3)
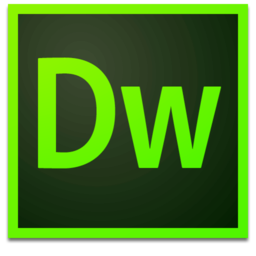
Dreamweaver Mac version
Visual web development tools
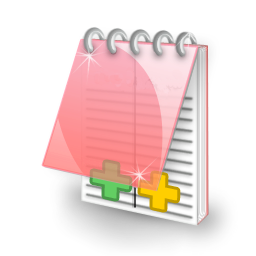
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
