Replace each node in a linked list with its surpasser count using C++
Given a linked list, we need to find an element in the given linked list that is greater than the right side of the current element. The count of these elements needs to be substituted into the value of the current node.
Let's take a linked list containing the following characters and replace each node with its surpasser count -
4 -> 6 -> 1 -> 4 -> 6 -> 8 -> 5 -> 8 -> 3
Start backward and traverse the linked list (so we don't need to worry about the current element on the left). Our data structure keeps track of the current element in sorted order. Replaces the current element in the sorted data structure with the total number of elements above it.
Through the recursive method, the linked list will be traversed backwards. Another option is PBDS. Using PBDS allows us to find elements that are strictly less than a certain key. We can add the current element and subtract it from the strictly smaller element.
PBDS does not allow duplicate elements. However, we need repeated elements to count. To make each entry unique, we will insert a pair in the PBDS (first = element, second = index). To find the total elements equal to the current element, we will use a hash map. A hash map stores the number of occurrences of each element (basic integer-to-integer mapping).
Example
The following is a C program to replace each node in a linked list with its transcendental number -
#include <iostream> #include <unordered_map> #include <ext/pb_ds/assoc_container.hpp> #include <ext/pb_ds/tree_policy.hpp> #define oset tree<pair<int, int>, null_type,less<pair<int, int>>, rb_tree_tag, tree_order_statistics_node_update> using namespace std; using namespace __gnu_pbds; class Node { public: int value; Node * next; Node (int value) { this->value = value; next = NULL; } }; void solve (Node * head, oset & os, unordered_map < int, int >&mp, int &count){ if (head == NULL) return; solve (head->next, os, mp, count); count++; os.insert ( { head->value, count} ); mp[head->value]++; int numberOfElements = count - mp[head->value] - os.order_of_key ({ head->value, -1 }); head->value = numberOfElements; } void printList (Node * head) { while (head) { cout << head->value << (head->next ? "->" : ""); head = head->next; } cout << "\n"; } int main () { Node * head = new Node (43); head->next = new Node (65); head->next->next = new Node (12); head->next->next->next = new Node (46); head->next->next->next->next = new Node (68); head->next->next->next->next->next = new Node (85); head->next->next->next->next->next->next = new Node (59); head->next->next->next->next->next->next->next = new Node (85); head->next->next->next->next->next->next->next->next = new Node (37); oset os; unordered_map < int, int >mp; int count = 0; printList (head); solve (head, os, mp, count); printList (head); return 0; }
Output
43->65->12->46->68->85->59->85->30 6->3->6->4->2->0->1->0->0
illustrate
So, for the first element, element = [65, 46, 68, 85, 59, 85], which is 6
The second element, element = [68, 85, 85] i.e. 3
And so on for all elements
in conclusion
This question requires a certain understanding of data structure and recursion. We need to lay out methods and then, based on observations and knowledge, derive data structures that meet our needs. If you liked this article, read more and stay tuned.
The above is the detailed content of Replace each node in a linked list with its surpasser count using C++. For more information, please follow other related articles on the PHP Chinese website!
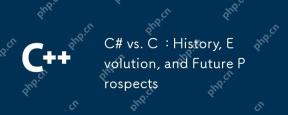
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
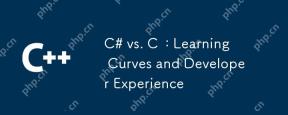
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
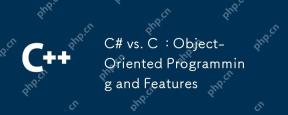
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
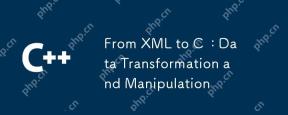
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
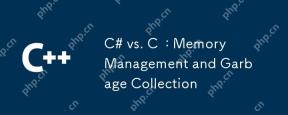
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
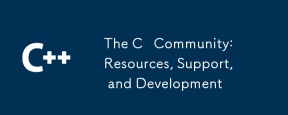
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
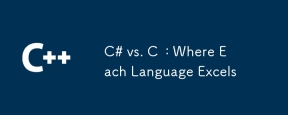
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
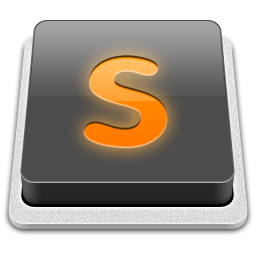
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.