


How to use forced inheritance to proxy final classes in Java to increase code scalability?
How to use forced inheritance to proxy final classes in Java to increase the scalability of the code?
In traditional object-oriented programming, we often use inheritance to achieve code reuse and expansion. However, there is a special class in the Java language, the final class, which prohibits other classes from inheriting it. This is very useful for situations where you need to limit the behavior of a class or protect the implementation details of a class, but it also brings certain challenges to the scalability of the code.
In this case, we can use forced inheritance proxies to bypass the restrictions of final classes, thereby increasing the scalability of the code. Forced proxy inheritance is a design pattern that implements proxy access to a final class by including a final class object in another class and wrapping the object's methods in a new class. This way we can extend the functionality of a final class without violating its limitations.
Below we use an example to illustrate how to use forced inheritance agents in Java to increase the scalability of the code.
Suppose we have a final class called TextUtil, which provides some text processing methods, but we want to extend its functionality, such as adding a new method for counting the number of words in the text. We can achieve this through the following steps:
Step 1: Create a new class called TextUtilProxy and define a TextUtil object in it.
public class TextUtilProxy { private TextUtil textUtil = new TextUtil(); // 新方法:统计文本中单词的数量 public int countWords(String text) { String[] words = textUtil.splitText(text); // 调用TextUtil对象的方法 return words.length; } // 其他方法与TextUtil类相同,通过代理调用TextUtil对象的对应方法 public String capitalize(String text) { return textUtil.capitalize(text); } public String reverse(String text) { return textUtil.reverse(text); } // ... }
Step 2: Call the method of TextUtil object through TextUtilProxy class.
public class Main { public static void main(String[] args) { TextUtilProxy textUtilProxy = new TextUtilProxy(); String text = "Hello, world!"; System.out.println("Capitalized text: " + textUtilProxy.capitalize(text)); System.out.println("Reversed text: " + textUtilProxy.reverse(text)); System.out.println("Word count: " + textUtilProxy.countWords(text)); } }
Through the above code example, we successfully bypassed the limitations of the final class TextUtil by using the forced inheritance proxy and added a new method to count the number of words in the text. In this way, we not only retain the encapsulation characteristics of the final class, but also increase the scalability of the code.
It should be noted that forced inheritance of proxies is not a solution suitable for all situations. When using forced inheritance proxies, we need to carefully consider the complexity of the design, the performance overhead, and the possible potential problems. In actual development, we need to judge whether to use forced inheritance agents based on specific circumstances.
To sum up, by using the forced inheritance proxy, we can bypass the restrictions of the final class in Java and expand its functions, thereby increasing the scalability of the code. However, we need to think carefully when using it and ensure that it is suitable for the current scenario.
The above is the detailed content of How to use forced inheritance to proxy final classes in Java to increase code scalability?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
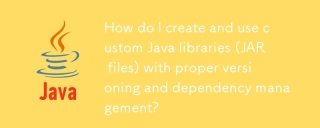
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
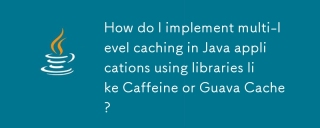
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
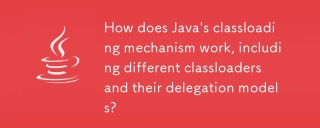
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
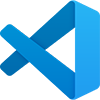
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
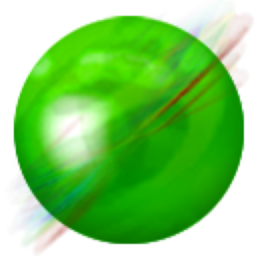
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
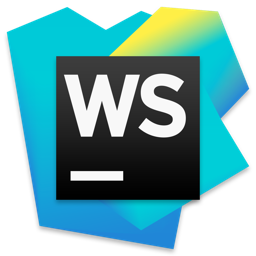
WebStorm Mac version
Useful JavaScript development tools