In JavaScript, a function is considered "curried" if it accepts one or more arguments and returns a new function that takes the remaining arguments. Currying is a powerful technique that can be used to create new functions from existing functions, or "currying" functions of depth n.
Why do we need to cancel function references?
There are several reasons why you might want to uncurry a certain function. For example, you might want -
Use curried functions in contexts that require uncurried functions
Convert a curried function to a non-curried form to make it easier to read or debug
Optimize the performance of curried functions
How to cancel function reference?
There are two waysCurrying functions -
The first method is to use the "Function.prototype.apply" method.
The second method is to use the "Function.prototype.call" method.
Use the "Function.prototype.apply" method
"Function.prototype.apply" method can be used to store functions of depth n. To do this, you need to simply pass the "this" value and the parameter array to the "apply" method. For example -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <p>uncurry up to depth 2 using Function apply() method</p> <div id="result"></div> <script> function add(a, b) { return a + b; } function curriedAdd(a) { return function(b) { return a + b; } } // Uncurry the "curriedAdd" function up to depth 2: document.getElementById("result").innerHTML = curriedAdd.apply(null, [1]).apply(null, [2]); // 3 </script> </body> </html>
Use the "Function.prototype.call" method
"Function.prototype.call" method can also be used to uncurry a function to depth n. The "call" method is similar to the "apply" method, but you pass the "this" value and arguments to the "call" i> method as separate arguments , rather than as an array. For example -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <p> uncurry up to depth 2 using Function call() method</p> <div id="result"></div> <script> function add(a, b) { return a + b; } function curriedAdd(a) { return function(b) { return parseInt(a) + parseInt(b); } } // Uncurry the "curriedAdd" function up to depth 2: document.getElementById("result").innerHTML = curriedAdd.call(null, [1]).call(null, [2]); // 3 </script> </body> </html>
In the above example, we used the "call" method to curry the "curriedAdd" function. As you can see, the "call" method is slightly more verbose than the "apply" method, but it has the same effect.
What are the benefits of using the "Function.prototype.apply" method?
There are several benefits of using the "Function.prototype.apply" method to dereference a function -
The "apply" method is faster than the "call" method.
The "apply" method is more widely supported than the "call" method.
The "apply" method is more concise than the "call" method.
In summary, currying is a powerful technique that can be used to create new functions from existing functions, or to "curry" functions of depth n.
The above is the detailed content of How to recurse a function to depth n in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
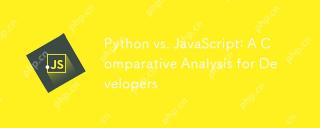
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
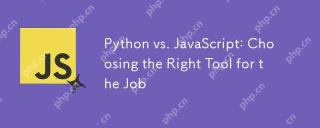
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
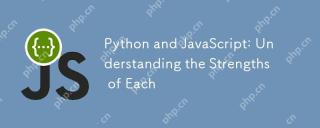
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
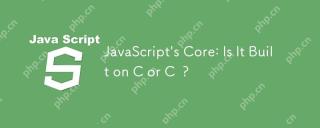
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
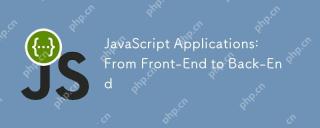
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
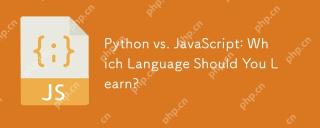
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
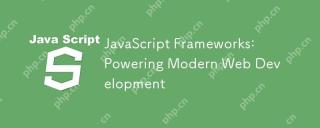
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
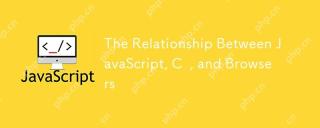
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
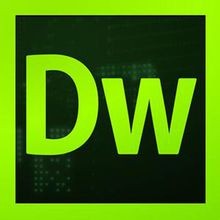
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
