How to use PHP to implement online chat function
Introduction:
With the development of the Internet, online chat function has become a must-have for many websites and applications characteristic. PHP, as a programming language widely used in web development, can also be used to implement online chat functions. This article will introduce how to use PHP to implement a simple online chat function, and provide code samples for readers' reference.
1. Preparation work
Before we start writing code, we need to do some preparation work. First, we need a web server and a database to store chat records. We chose to use Apache as the web server and MySQL as the database. In addition, we also need to install the PHP operating environment.
2. Create a database
We first need to create a database to store chat records. Use the MySQL command line tool or graphical interface to create a database named "chat" and a table named "messages", which is used to store information such as the content, sender and sending time of chat messages.
CREATE DATABASE chat;
USE chat;
CREATE TABLE messages (
id INT AUTO_INCREMENT PRIMARY KEY,
message TEXT,
sender VARCHAR(256),
timestamp DATETIME
);
3. Writing the front-end page
We need to create a front-end page to display chat messages and process user input. The following is a simple HTML page example:
<!DOCTYPE html> <html> <head> <title>在线聊天</title> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> </head> <body> <div id="chatbox"></div> <input type="text" id="message" placeholder="输入消息"> <button onclick="sendMessage()">发送</button> <script> // 向服务器发送消息 function sendMessage() { var message = $('#message').val(); $.post('send_message.php', {message: message}, function(data) { $('#message').val(''); }); } // 定时从服务器获取最新的聊天消息并显示在聊天框中 setInterval(function() { $.get('get_messages.php', function(data) { $('#chatbox').html(data); }); }, 1000); </script> </body> </html>
4. Writing back-end code
We use PHP to process user input and obtain the latest chat messages. The following is an example:
send_message.php:
<?php // 获取用户发送的消息 $message = $_POST['message']; // 将消息存储到数据库中 $servername = "localhost"; $username = "root"; $password = "password"; $dbname = "chat"; $conn = new mysqli($servername, $username, $password, $dbname); $sql = "INSERT INTO messages (message, sender, timestamp) VALUES ('$message', '用户', NOW())"; $conn->query($sql); $conn->close(); ?>
get_messages.php:
<?php $servername = "localhost"; $username = "root"; $password = "password"; $dbname = "chat"; $conn = new mysqli($servername, $username, $password, $dbname); $sql = "SELECT * FROM messages ORDER BY timestamp DESC"; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo $row['sender'] . ': ' . $row['message'] . '<br>'; } } else { echo "暂无聊天记录"; } $conn->close(); ?>
5. Run the program
Save the above code as the corresponding file (such as index.php, send_message.php and get_messages.php), place the front-end page file in the root directory of the web server, and then use the browser to open the page to start using the online chat function.
Conclusion:
The online chat function written in PHP can achieve real-time chat with other users, and this function can be used in various websites and applications. Readers can use the code examples provided in this article to master how to use PHP to implement online chat functions, and further expand and optimize according to actual needs.
The above is the detailed content of How to use PHP to implement online chat function. For more information, please follow other related articles on the PHP Chinese website!
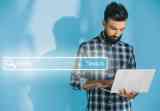
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
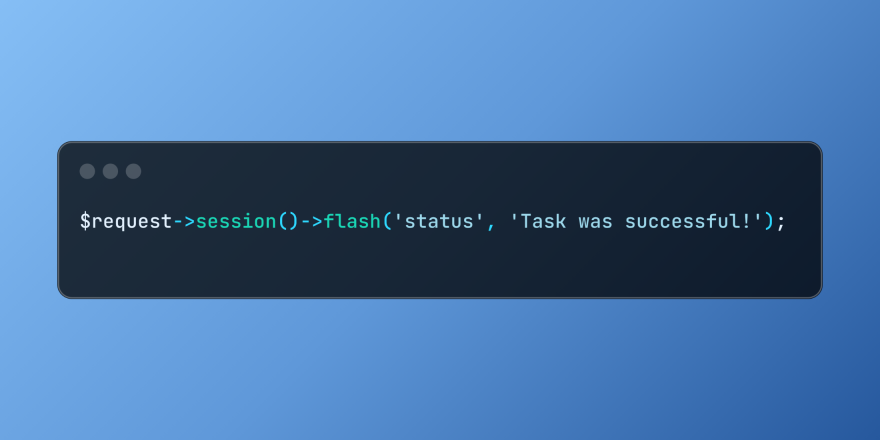
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
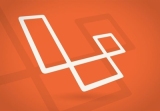
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
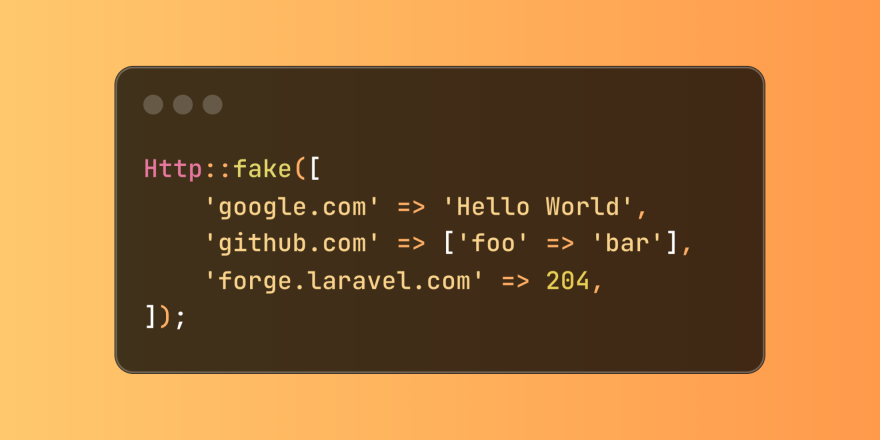
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
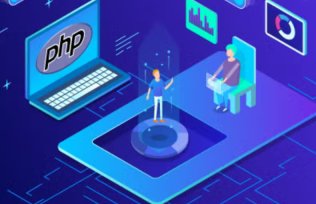
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
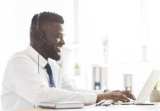
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
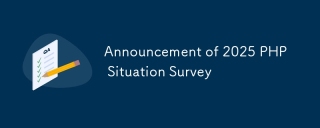
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
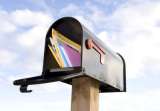
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
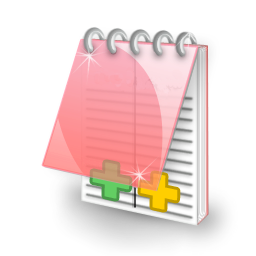
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
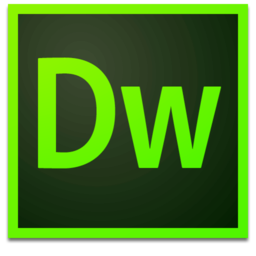
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
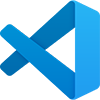
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
