A square pyramid number refers to the sum of the squares of natural numbers. Natural numbers include all numbers from 1 to infinity. For example, the first 4 square pyramid numbers are 1, 5, 14, and 30.
To understand better, consider the following fact: If we take the square pyramid of numbers at the beginning and stack the number balls in descending order, they will form a pyramid.
Problem Statement
Given a number Sum. If Sum is the sum of the squares of the first n natural numbers, return n, otherwise return false.
Example 1
is translated as:Example 1
Input = 30 Output = 4
Explanation = 30 is the sum of the squares of the first 4 natural numbers.
1*1 + 2*2 + 3*3 +4*4 = 30.
Therefore, the output should be 4.
Example 2
Input = 54 Output = -1
Explanation = There is no sum of squares of any n natural numbers equal to 54. Therefore, the output should be -1.
Solution to problem statement
There are two solutions to this problem.
Method 1: Violent solution
The brute force cracking method starts from n = 1. Create a variable 'total' that adds the square of the next natural number to the previous value of total. Returns n if total is equal to Sum, otherwise returns false if total is greater than Sum.
pseudocode
start n =1 While (total < sum ): Total += n*n n=n+1 if(total == sum) : Return n Else: Return false end
Example
Below is a C program to check whether a given number is the sum of squares of natural numbers.
#include <iostream> using namespace std; // This function returns n if the sum of squares of first // n natural numbers is equal to the given sum // Else it returns -1 int square_pyramidal_number(int sum) { // initialize total int total = 0; // Return -1 if Sum is <= 0 if(sum <= 0){ return -1; } // Adding squares of the numbers starting from 1 int n = 0; while ( total < sum){ n++; total += n * n; } // If total becomes equal to sum return n if (total == sum) return n; return -1; } int main(){ int S = 30; int n = square_pyramidal_number(S); cout<< "Number of Square Pyramidal Numbers whose sum is 30: "<< endl; (n) ? cout << n : cout << "-1"; return 0; }
Output
Number of Square Pyramidal Numbers whose sum is 30: 4
Time complexity - O(sum), where sum is the given input.
Space complexity - O(1): no extra space used.
Method 2 using Newton-Raphson method
Another method is the Newton-Raphson method. Newton-Raphson method Used to find the roots of a given function f(x) and an initial guess of the roots.
sum of squares of first n natural numbers = n * (n + 1) * (2*n + 1) / 6, n * (n + 1) * (2*n + 1) / 6 = sum or k * (k + 1) * (2*k + 1) – 6*sum = 0
So n is the root of this cubic equation and can be calculated using the Newton-Raphson method which involves starting from an initial guess value x0 and using the following formula to find the next value x i.e. from the previous value xn Get xn 1.
$$\mathrm{x_{1}=x_{0}-\frac{f(x_{0})}{f^{'}(x_{0})}}$$
pseudocode
Start calculate func(x) and derivativeFunction(x) for given initial x Compute h: h = func(x) / derivFunc(x) While h is greater than allowed error ε h = func(x) / derivFunc(x) x = x – h If (x is an integer): return x Else: return -1; end
Example
The following is a C program for checking whether a given number is the sum of the squares of natural numbers.
#include<bits/stdc++.h> #define EPSILON 0.001 using namespace std; // According to Newton Raphson Method The function is // k * (k + 1) * (2*k + 1) – 6*sum or 2*k*k*k + 3*k*k + k - 6*sum double func(double k, int sum){ return 2*k*k*k + 3*k*k + k - 6*sum; } // Derivative of the above function is 6*k*k + 6*k + 1 double derivativeFunction(double k){ return 6*k*k + 6*k + 1; } // Function to check if double is an integer or not bool isInteger(double N){ int X = N; double temp2 = N - X; if (temp2*10 >=1 ) { return false; } return true; } // Function that finds the root of k * (k + 1) * (2*k + 1) – 6*sum int newtonRaphson(double k, int sum){ double h = func(k, sum) / derivativeFunction(k); while (abs(h) >= EPSILON){ h = func(k, sum)/derivativeFunction(k); // k(i+1) = k(i) - f(k) / f'(k) k = k - h; } if (isInteger(k)) { return (int)k; } else { return -1; } } // Driver program int main(){ double x0 = 1; // Initial values assumed int sum = 91; int n = newtonRaphson(x0,sum); cout<< "Number of Square Pyramidal Numbers whose sum is 91: "<< endl; (n) ? cout << n : cout << "-1"; return 0; }
Output
Number of Square Pyramidal Numbers whose sum is 91: 6
Time Complexity - O((log n) F(n)) where F(n) is the cost of calculating f(x)/f'(x), with n-digit precision.
Space complexity - O(1): no extra space used.
in conclusion
This article solves the problem of finding the square pyramid number of a given sum. We introduce two methods: one is a brute force method and the other is an efficient method. Both methods provide C programs.
The above is the detailed content of Square pyramid numbers (sum of squares). For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
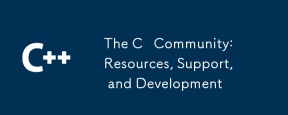
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
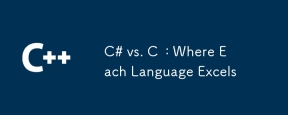
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
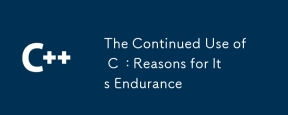
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
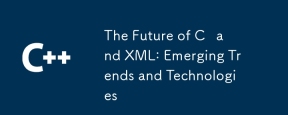
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
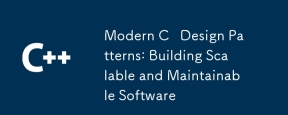
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
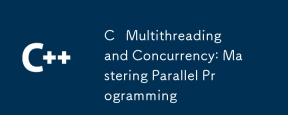
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
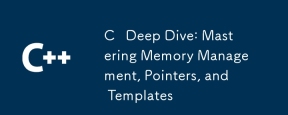
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
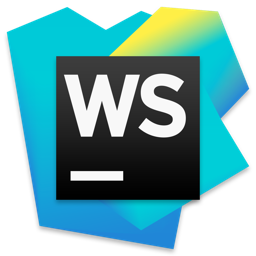
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.