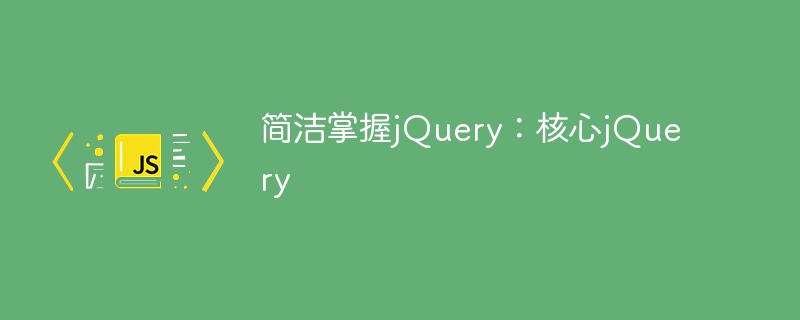
Basic concepts behind jQuery
While there are some conceptual variations in the jQuery API (such as functions such as $.ajax
), the central concept behind jQuery is "find something, do something". More specifically, select DOM elements from an HTML document and then use jQuery methods to perform certain operations on them. This is the big picture concept.
To understand this concept deeply, consider the following code.
<!DOCTYPE html>
<html lang="en">
<body>
<!-- jQuery will change this -->
<a href=""></a>
<!-- to this <a href="https://www.jquery.com">jQuery</a> -->
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script>
<script>
jQuery('a').text('jQuery').attr('href', 'https://www.php.cn/link/51ef70624ca791283ec434a52da0d4e2');
</script>
</body>
</html>
Please note that in this HTML document we are using jQuery to select DOM elements (<a></a>
). After selecting something, we then perform some actions on the selection by calling the jQuery methods text()
, attr()
, and appendTo()
.
The
text
method is called on the wrapped <a></a>
element and sets the element's display text to "jQuery". attr
Called to set the href
attribute to the jQuery site.
Understanding the basic concept of "find something, do something" is critical to progress as a jQuery developer.
Concept, behind the concept, behind jQuery
While selecting something and doing something are the core concepts behind jQuery, I wanted to expand this concept to include creating something. Therefore, the concepts behind jQuery can be expanded to include first creating something new, selecting it, and then doing something with it. We can call it the concept behind jQuery, the concept behind the concept.
What I want to point out is that you don't just select what's already in the DOM. Another important point about grok is that jQuery can be used to create new DOM elements and then perform certain operations on those elements.
In the code example below, we create a new <a></a>
element that is not in the DOM. Once created, it is selected and acted upon.
<!DOCTYPE html>
<html lang="en">
<body>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script>
<script>
jQuery('<a>jQuery</a>').attr('href', 'https://www.php.cn/link/51ef70624ca791283ec434a52da0d4e2').appendTo('body');
</script>
</body>
</html>
The key concept to grasp here is that we are dynamically creating the <a></a>
element and then manipulating it as if it were already in the DOM.
jQuery requires HTML to run in standards mode or near standards mode
There is a known issue with jQuery methods not working properly when the browser renders the HTML page in weird mode. Make sure that when you use jQuery, the browser interprets HTML in standards mode or almost standards mode by using a valid document type.
To ensure correct functionality, the code examples in this book use the HTML 5 document type.
<!DOCTYPE html>
Waiting for DOM to be ready
When the DOM is loaded and available for manipulation, jQuery triggers a custom event named ready
. Code that manipulates the DOM can be run in the handler of this event. This is a common pattern in jQuery usage.
The following examples show three coding examples of this custom event in use.
<!DOCTYPE html>
<script>
// Standard
jQuery(document).ready(function () { alert('DOM is ready!'); });
// Shortcut, but same thing as above
jQuery(function () { alert('No really, the DOM is ready!'); });
// Shortcut with fail-safe usage of $. Keep in mind that a reference
// to the jQuery function is passed into the anonymous function.
jQuery(function ($) {
alert('Seriously its ready!');
// Use $() without fear of conflicts
});
</script>
Remember that you can attach as many ready()
events to the document as you want. You are not limited to just one. They are executed in the order they are added.
Execute jQuery code when the browser window is fully loaded
Normally, we don't want to wait for the window.onload
event. This is the point of using a custom event like ready()
which will execute code before the window loads and after the DOM is ready to be traversed and manipulated.
However, sometimes we do want to wait. While the custom ready()
event is great for executing code after the DOM is available, we can also use jQuery to execute code after the entire web page has fully loaded, including all resources.
This can be done by attaching a load event handler to the window
object. jQuery provides the load()
event method that can be used to call a function after the window is fully loaded. Below, I've provided an example usage of the load()
event method.
<!DOCTYPE html>
<script>
// Pass window to the jQuery function and attach
// event handler using the load() method shortcut
jQuery(window).load(function(){ alert('The page and all its assets are loaded!'); });
</script>
Include all CSS files before including jQuery
Starting with jQuery 1.3, the library no longer guarantees that all CSS files are loaded before the custom ready()
event is fired. Due to this change in jQuery 1.3, you should always include all CSS files before any jQuery code. This will ensure that the browser has parsed the CSS before redirecting to JavaScript contained later in the HTML document. Of course, images referenced via CSS may or may not be downloaded when the browser parses the JavaScript.
Use the hosted version of jQuery
When embedding jQuery into a web page, most people choose to download the source code and link to it from a personal domain/host. However, there are other options that require someone else to host the jQuery code for you.
Google hosts multiple versions of the jQuery source code for anyone to use. This is actually very convenient. In the code example below, I use the <script></script>
element to include a minified version of jQuery hosted by Google.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script>
Google 还托管了多个以前版本的 jQuery 源代码,并且对于每个版本,都提供了缩小版和非缩小版变体。我建议在开发过程中使用非缩小变体,因为在处理非缩小代码时调试错误总是更容易。
使用 Google 托管版本的 jQuery 的一个好处是它可靠、快速并且可以缓存。
不使用 Ready() 解析 DOM 时执行 jQuery 代码
并不完全需要自定义 ready()
事件。如果您的 JavaScript 代码不影响 DOM,您可以将其包含在 HTML 文档中的任何位置。这意味着如果您的 JavaScript 代码不依赖于完整的 DOM,您可以完全避免 ready()
事件。
现在大多数 JavaScript,尤其是 jQuery 代码,都会涉及到操作 DOM。这意味着 DOM 必须由浏览器完全解析才能进行操作。这就是为什么开发人员几年来一直陷在 window.onload
过山车上的原因。
为了避免对 DOM 操作的代码使用 ready()
事件,您只需将代码放在 HTML 文档中的
The above is the detailed content of Mastering jQuery Simplistically: Core jQuery. For more information, please follow other related articles on the PHP Chinese website!