Encrypt a string by repeating the i-th character i times
Introduction
C A string is a fixed sequence of alphanumeric characters. It is a continuous stream of characters, which can be numbers, characters or even special symbols. Every string has a certain length. Access character positions start from 0.
Strings can contain unique characters or repeated characters concatenated together. They can perform various operations and series operations.
In this article, we will develop a code that takes a string as input and displays the encrypted string where the first character is repeated 1 time and the second character is repeated 2 times. Repeat this process until the length of the string is reached. Let us see the following example to understand this topic better -
Example
Example 1 - str - "g@m$"
Output - g@@mmm$$$$
For example, the example string below also contains special characters that are repeated based on the character's position in the string.
In this article, we will create a solution to count the number of times a character at a specific position should be repeated. The extracted characters are then appended to the resulting output string until the count is exhausted.
grammar
str.length()
length()
The size of a string can be captured through the length() method, which is used to return the alphanumeric characters and special symbols contained in the string
algorithm
Accepts input string str as input
A counter, cnt is used to store the number of times each character should be repeated. Its initial value is 0.
The length of the string is calculated using the length() method and stored in a variable named len
Extract the character at the i-th position each time.
The counter cnt is calculated by increasing position i by 1.
Execute a decreasing loop initialized with the counter value, appending the extracted characters to the output string res
The counter value is decremented each time
After performing the required number of iterations on the character, the pointer will move to the next character
Example
The following C code snippet is used to create an encrypted string based on the given input example string -
//including the required libraries #include <bits/stdc++.h> using namespace std; // Function to return the encrypted string string encrypt(string str) { //counter to store the number of times a character is repeated int cnt = 0; //storing the encrypted string string res = ""; //computing the length of the string int len = str.length(); for(int i =0 ; i<len ; ) { //getting the count of the number of times the character will be repeated cnt = i + 1; //repeating the current character while (cnt){ //extracting the character at ith index char ch = str[i]; //adding the character to output string res += ch; //decrementing the count cnt--; } i++; } cout << "Encrypted string "<< res; } int main() { //taking a input string string str = "heyy"; cout << "Input string "<< str <<"\n";; //creating an encrypted string encrypt(str); return 0; }
Output
Input string heyy Encrypted string heeyyyyyyy
in conclusion
C The character position in the string starts from the 0th index by default. A string is a dynamic-length storage structure in which characters can be appended easily any number of times. In C, string concatenation can be easily performed using the operator. Each time a character is added, the length of the string increases by 1.
The above is the detailed content of Encrypt a string by repeating the i-th character i times. For more information, please follow other related articles on the PHP Chinese website!
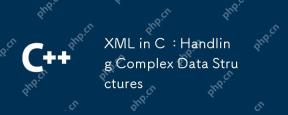
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
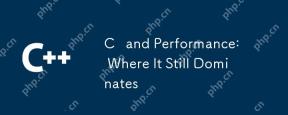
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
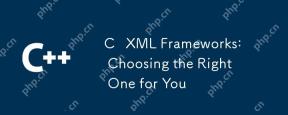
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
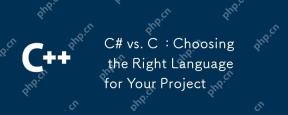
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
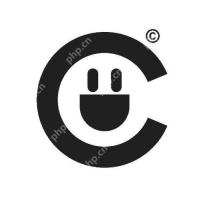
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
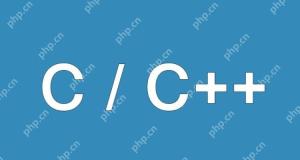
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
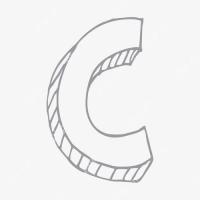
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
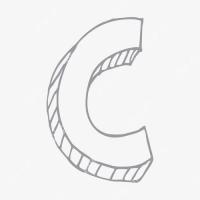
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
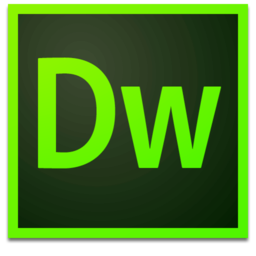
Dreamweaver Mac version
Visual web development tools
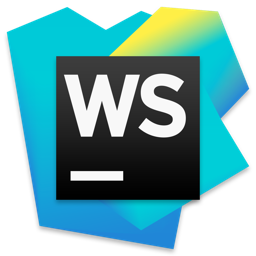
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
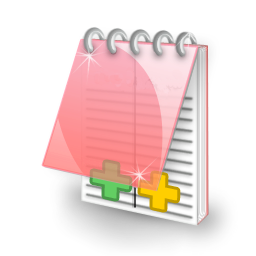
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
