We can create a Polygon object by creating an instance of fabric.Polygon. A polygon object can be characterized as any closed shape consisting of a set of connected straight line segments. Since it is one of the basic elements of FabricJS, we can also easily customize it by applying properties such as angle, opacity, etc. We use the rotating event to demonstrate how to make a polygonal object react to rotation through controls.
grammar
polygon.on(“rotating”, callbackFunction);
Example 1: Show how an object responds to rotation events
Let's look at a code example of how to make a polygon object react to rotation events. In this case, whenever we click on the polygon object and rotate it via the middle rotation control, we will see the recorded output. This is because the rotation event fires continuously as the object rotates.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2 id="Displaying-how-the-object-reacts-to-the-rotating-event">Displaying how the object reacts to the rotating event</h2> <p>You can rotate the object to see the callback function fired</p> <canvas id="canvas"></canvas> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiate a polygon instance var polygon = new fabric.Polygon( [ { x: 500, y: 20 }, { x: 550, y: 60 }, { x: 550, y: 200 }, { x: 350, y: 200 }, { x: 350, y: 60 }, { x: 500, y: 20 }, ], { fill: "red", stroke: "blue", strokeWidth: 2, objectCaching: false, } ); // Adding it to the canvas canvas.add(polygon); // Using the rotating event polygon.on("rotating", () => { canvas.renderAll(); console.log("The polygon object is rotating"); }); </script> </body> </html>
Example 2: Changing fill color when rotation occurs
Let's look at a code example to see how to change the fill color when a rotate event occurs. We can use the middle rotation (mtr) control to rotate objects on the canvas. Here, when we rotate the polygon object using the mtr control, the fill color changes to "green".
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2 id="Changing-the-fill-colour-when-rotate-happens">Changing the fill colour when rotate happens</h2> <p> You can see that the fill colour changes when the polygon is rotated </p> <canvas id="canvas"></canvas> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiate a polygon instance var polygon = new fabric.Polygon( [ { x: 500, y: 20 }, { x: 550, y: 60 }, { x: 550, y: 200 }, { x: 350, y: 200 }, { x: 350, y: 60 }, { x: 500, y: 20 }, ], { fill: "red", stroke: "blue", strokeWidth: 2, objectCaching: false, top: 50, left: 30, scaleX: 0.5, scaleY: 0.5 } ); // Adding it to the canvas canvas.add(polygon); // Using the rotating event polygon.on("rotating", () => { polygon.set("fill", "green") canvas.renderAll(); }); </script> </body> </html>
in conclusion
In this tutorial, we use two simple examples to demonstrate how to make a polygon object react to rotation events using FabricJS.
The above is the detailed content of How to make a polygon object react to rotation events using FabricJS?. For more information, please follow other related articles on the PHP Chinese website!
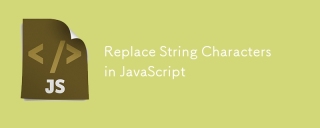
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
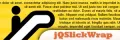
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
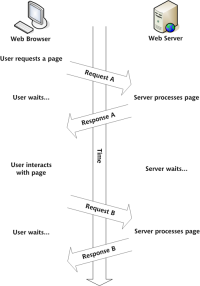
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
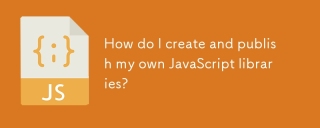
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
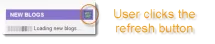
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
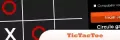
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
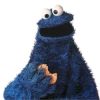
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session
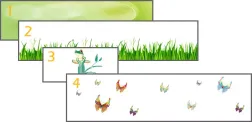
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
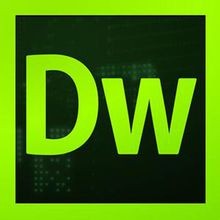
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
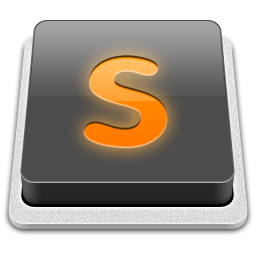
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
