Modern JavaScript libraries are quite a behemoth – just look at jQuery. When you're creating mobile apps, or even just targeting modern browsers, cleaner, more flexible libraries become a tastier proposition.
Today, we will introduce one such library called Zepto.
Problems with mixing desktop libraries and mobile devices
The rise of mobile devices has been overlooked by most people.
You see, the Internet and the technology that supports it have grown by leaps and bounds in the past few years. We moved from static websites to web applications to dynamic web applications and then to today’s real-time, hyper-responsive Thingamabob applications. One thing that most people haven't noticed is the rise of mobile devices.
Think about it: Many of us use smartphones and use them to browse on a regular basis. Even at home, a significant portion of my circle has adopted tablet devices for casual surfing and emailing. While this influx of devices is a good thing from an exposure perspective, there are some caveats.
As developers, we should not think of these devices as display-limited the way consumers do, but rather need to think of them from a resource and bandwidth perspective. Not all devices have Quad Gigabit Super CPUs or come with large amounts of memory. We don't even start with bandwidth. A large portion of the browsing population is still stuck on these hellish excuses for mobile internet connectivity.
I think you already understand what I mean. Big monolithic libraries like jQuery or Prototype definitely have their place, but for this mobile age, I think there's a place for something more flexible. Many developers seem to agree with me.
All the code that makes the library work cross-browser adds up
Another big problem I didn't mention is that contemporary libraries do a lot of cross-browser stuff. In fact, one of the initial appeals of jQuery was how it abstracted away many of the cross-browser quirks that front-end developers had to deal with. Even now, jQuery does a lot of heavy lifting under the hood to ensure that no issues arise across different browsers.
But if you are a developer who just wants to cater to contemporary devices, then dare I say, do you really need all this? The simplest answer is no. By removing unnecessary code, you can:
- Performance can be improved since the browser needs to parse fewer lines of code
- Reduce file size, which helps on mobile devices with limited bandwidth.
Do you think this issue has been exaggerated? Here's a random chunk of code from the jQuery source code:
isPlainObject: function( obj ) { // Must be an Object. // Because of IE, we also have to check the presence of the constructor property. // Make sure that DOM nodes and window objects don't pass through, as well if ( !obj || jQuery.type(obj) !== "object" || obj.nodeType || jQuery.isWindow( obj ) ) { return false; } ....
Or something more esoteric:
// Perform a simple check to determine if the browser is capable of // converting a NodeList to an array using builtin methods. // Also verifies that the returned array holds DOM nodes // (which is not the case in the Blackberry browser) try { Array.prototype.slice.call( document.documentElement.childNodes, 0 )[0].nodeType; // Provide a fallback method if it does not work } catch( e ) { // The intended fallback } ....
This may seem rather trivial, but remember, this often adds up. If you only want to target modern browsers (whether desktop or mobile), then there's no need to do all these extra checks and hacks. By having fewer browsers than you want, you get a win-win in bandwidth and performance!
What’s the deal with Zepto?
I hear you saying "Enough accumulation! Already told us about the damn library!". So let's get started.
Zepto, as the title suggests, is a mobile JavaScript framework that corrects both of the above problems. Its codebase is very small, weighing around 8kb.
It's so clean by mostly removing cross-browser content. When it was created, the main focus was to support Webkit only. The mobile version of Webkit to be precise. Now, it's been extended to work with desktop browsers - but only on modern browsers. In this IE6, no more fumbling to get things working!
Zepto’s API is compatible with jQuery. If you use jQuery, you already know how to use Zepto.
Another area where Zepto manages to be small is in how it avoids feature bloat. The core library doesn't seem to contain any extraneous functionality. Even AJAX and animation functionality are available as separate modules if required. This is an absolute godsend for users who primarily use the library for DOM traversal and manipulation.
Oh, did I mention Zepto’s main party piece? Zepto's API is compatible with jQuery. If you use jQuery, you already know how to use Zepto.
Are Zepto and jQuery interchangeable?
Yes and no. Depends is a more appropriate answer.
Yes, because Zepto's core API mimics jQuery to a large extent. To make it easy to use and significantly shorten the learning curve, Zepto emulates jQuery's API. Most commonly used methods (such as DOM operations) have almost identical names and have the same parameters in the same order. To an engineer, the method signature is the same.
Let’s look at a small example:
$('#element').html("Hey! Are you on the GW2 beta?");
看起来很眼熟吗?它应该。这与您使用 jQuery 来更改元素的 HTML 的代码完全相同。正如我所提到的,这不仅限于此方法。大多数 DOM 操作都是以与您的实用程序相同的方式构建的,例如 AJAX。
另一方面,API 并非 100% 匹配。 Zepto 放弃了 jQuery 中存在的一些可能破坏代码的方法。同样重要的是,由于 Zepto 是 jQuery 的子集,因此您可能会错过内置的特定功能—— Deferred
就是一个很好的例子。您根本无法将 jQuery 替换为 Zepto 并期望一切正常。
对我来说,最大的障碍是从 jQuery 复制的方法,但具有不同的签名和功能集。当你认为自己使用了正确的方法但事实并非如此时,你会感到有点沮丧。克隆方法复制事件处理程序的能力就是一个很好的例子。如果不查看源代码,我真的不会发现这一点。
探索核心 API
如果您以前使用过 jQuery,那么下面的所有内容都应该是一场小憩。
闲聊已经够多了,现在让我们深入研究一些代码。与许多现代库一样,DOM 遍历和操作是每个人都希望完善的核心功能。由于 API 和整体功能与 jQuery 非常相似,我认为您可以放心地假设一切都是一流的。
让我们看一下一些常见的 DOM 相关功能。
修改容器的 HTML 内容
这是 DOM 操作的基础:读取或更改元素的 HTML 内容。使用 Zepto,就像在容器上调用 html
方法一样简单,并在需要时传入新的 HTML。
例如,这会获取元素的 HTML 并将其存储在变量中。
var containerText = $('#element').html();
或者如果您想将其更改为其他内容:
$('#element').html("Hola there!");
很简单,对吧?
将元素添加到容器中
与 jQuery 一样,Zepto 使用 append
和 prepend
方法。并且调用也保持不变。
$('#element').append("<p>This is the appended element.</p>"); // or $('#element').prepend("<p>This is the appended element.</p>");
活动
事件是任何现代应用程序的支柱,Zepto 为您提供了一系列易于使用的方法来完成您的工作。大部分工作是通过 on
方法完成的。
$('#element').on('click', function(e){ // Your code here });
易于阅读且易于解析。如果您感觉老派并且想使用 bind、delegate
或 live
方法,请不要这样做。就像 jQuery 一样,它们在这里已被弃用。
AJAX
任何现代低级库都需要提供一个易于使用的 AJAX 包装器,而 Zepto 不会让您失望。这是一个超级简单的 AJAX 请求的示例。
$.ajax({ type: 'POST', url: '/project', data: { name: 'Super Volcano Lair' }, dataType: 'json', success: function(data){ // Do some nice stuff here }, error: function(xhr, type){ alert('Y U NO WORK?') } });
事情可能看起来有点复杂,但我们正在做的事情可以归结为:
- 创建 AJAX 对象并向其传递选项。
- 在选项中,指定我们想要执行 POST 请求。我想默认是 GET。
- 指定要发布到的 URL。
- 指定需要发送到服务器的数据。正如你所看到的,我正在疯狂地大笑,并试图创建我自己的超级恶棍巢穴。
- 指定请求成功或失败时将触发的方法。这样,无论发生什么情况,我们都可以更新 UI。
与 jQuery 一样,有单独的方法用于 GET 或 POST 请求,或者仅加载一些 Web 内容。
动画
如果没有一些动画,世界将会变成什么样子? Zepto 公开了全能的 animate
方法,该方法应该可以处理大多数您的动画需求。
$('#element').animate({ opacity: 0.50, top: '30px', color: '#656565' }, 0.5)
我们基本上是选择要动画的元素,调用 animate
方法并指定要动画的属性以及完成动画所需的时间。 Zepto 会完成剩下的工作。
或者,如果您只需要显示和隐藏一个元素,则切换应该可以正常工作。
我想您明白了——Zepto 的 DOM、动画和事件 API 在很大程度上模拟了 jQuery。众所周知,jQuery 在这些方面非常擅长。如果您以前使用过 jQuery,那么您在这里应该不会遇到太多麻烦。
了解触摸事件和其他细节
Zepto 确实为您提供了一些可以在应用程序中利用的触摸特定事件。其中包括:
- 滑动——处理典型的滑动动作。不同方向也有单独的事件,例如 swipeLeft。
- tap -- 响应通用点击操作而触发。
- doubleTap——显然,这可以处理双击。
- longTap -- 当元素被点击超过 750 毫秒时触发。不过,改变这种延迟似乎并不容易。
这是一个简单的示例,取自 Zepto 的文档。
<ul id=items> <li>List item 1 <span class=delete>DELETE</span></li> <li>List item 2 <span class=delete>DELETE</span></li> </ul> <script> // show delete buttons on swipe $('#items li').swipe(function(){ $('.delete').hide() $('.delete', this).show() }) // delete row on tapping delete button $('.delete').tap(function(){ $(this).parent('li').remove() }) </script>
当列表项被扫描时,所有其他列表元素的删除按钮都会隐藏,只显示当前的删除按钮。点击删除按钮会删除该按钮的父 li 项目,从而从 DOM 中删除。
This should be very similar to how you normally handle events, except that you bind the handler to a different event and that's it.
Summarize
This is perfect for me considering what I'm developing for and for; but as always, your mileage may vary.
Well, that’s pretty much what Zepto is all about. Essentially, it’s a stripped-down, free version of jQuery that can be used on mobile devices. Over time, it has evolved into a streamlined library that no longer supports outdated browsers.
This is perfect for me considering what I'm developing for and for; but as always, your mileage may vary. You might get stuck using jQuery plugins that require significant modifications to make them work under Zepto, or just feel more confident with jQuery.
Either way, you really need to try Zepto out and see how it fits into your workflow before you cancel. I did it and I loved it!
Okay, that’s all I have for today. Let me know your thoughts in the comments below and thank you so much for reading!
The above is the detailed content of Zepto.js: Uncovering the essential elements. For more information, please follow other related articles on the PHP Chinese website!
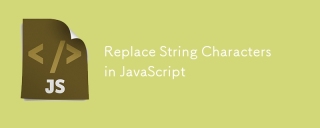
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
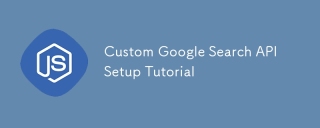
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
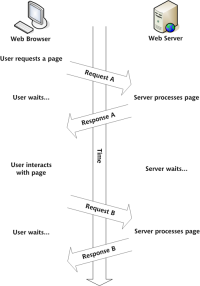
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
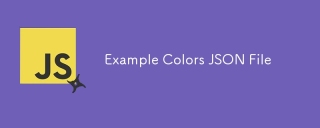
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
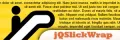
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
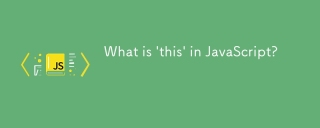
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
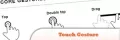
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
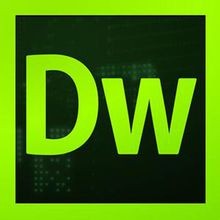
Dreamweaver CS6
Visual web development tools
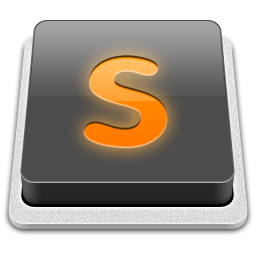
SublimeText3 Mac version
God-level code editing software (SublimeText3)
