


In C, we have a built-in reverse() function for reversing a substring to check if a string can become smaller lexicographically. Dictionary ordering is the process of sorting the characters of a word into dictionary order.
Let us take a string example to check whether the lexicographic order is smaller.
We will compare these two words to check for the lexicographically smaller word and take two strings, 'apple' and 'army'. The first letter of both strings begins with the letters ‘a’. When we examine the second character of the two letters, alphabetically, ‘p’ comes before ‘r’. Therefore, lexicographically, apple is smaller than army.
In the string "tutorialspoint", reverse the substring "oria" to get "airo", the substring Smaller in lexicographic order. Then write the final string as "tutairolspoint".
-
In the string "tutorix", reverse the substring "tori" to get "irot", because the first The starting character of the substring is 't', and the second substring is 'i', so 'i' is located at # in the alphabet before ##'t', so 'irot' is lexicographically smaller than 'tori'. The final string is written "tuirotx"
"acwz".
grammar
reverse( str_name.begin(), str_name.end() )
illustrateThe reverse function is part of the C standard library. It accepts two parameters
"str_name.begin()" and "str_name.end()".
str_name is a user-suggested string name.
-
begin() and end() are predefined built-in functions used under the reverse function. The job of the begin function is to return an iterator pointing to the first character of the input string. The end function returns an iterator that points to a position before the last character of the input string.
algorithm
First, we will use the three necessary header files, namely iostream, string and include
- We will start in the main function where we declare a string variable named
'str' and store the string 'tutorialspoint' in it. We will then declare the boolean variable 'isReverse' as 'false' to indicate that the given string has not been reversed and is still in its original form.
- We will then create two nested for loops to check every possible substring of
'str'. The substring is then stored in a temporary string named 'temp'.
- Afterwards, we call the
'reverse()' function to reverse the substring stored in the 'temp' variable between index 'i' and String 'j'.
- Later create an if statement to check if the reversed string is lexicographically less than the variables
'temp' and 'str' are compared.
- The compiler will compare the variables temp and str. If they are both equal, then the variable
'isReverse' will be set to true and it will break the if statement.
- Now, we check the value of isReverse and if it is true, print the if conditional statement, otherwise print the else conditional statement.
- exit the program.
In this program we will see how to make a string lexicographically smaller by reversing any substring.
#include <iostream> #include <string> #include <algorithm> using namespace std; int main() { string str = "tutorialspoint"; // User can change this to test other strings such as acwz, groffer, etc bool isReverse = false; // it is used to indicate whether or not a substring has been found in the original string “str”. // use the loop through all possible substrings of str for (int i = 0; i < str.length(); i++) { for (int j = i+1; j < str.length(); j++) { string temp = str; // create new temporary variable to store the value of str. // reverse the substring of i and j reverse ( temp.begin() + i, temp.begin() + j + 1 ); // reverse function follow the header file name as <algorithm> if (temp < str) { // Check whether the reversed string is lexicographically smaller isReverse = true; break; } } } if ( isReverse ) { cout << "Yes, this is lexicographically smaller by reversing a substring." << endl; } else { cout << "No, this is not lexicographically smaller by reversing a substring." << endl; } return 0; }Output
If we enter the value "tutorialspoint" we will get the following results -
Yes, this is lexicographically smaller by reversing a substring.If we enter the value "acwz" we will get the following results:
No, this is not lexicographically smaller by reversing a substring.in conclusion
We saw how to use string variables to calculate the smaller value in a dictionary by reversing any substring. Then we set the string variable to a temporary variable. We then use the predefined function "reverse()" to calculate the dictionary words in their reverse form. Next, we check the lexicographically smaller one by comparing the variables temp and str and get the result.
The above is the detailed content of Checks whether a string can be made lexicographically smaller by reversing any substring. For more information, please follow other related articles on the PHP Chinese website!
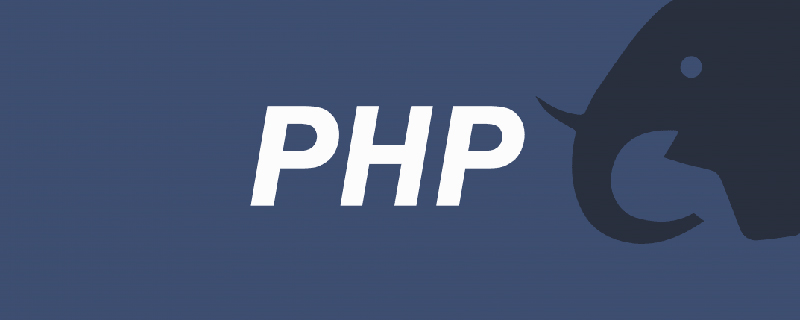
php将16进制字符串转为数字的方法:1、使用hexdec()函数,语法“hexdec(十六进制字符串)”;2、使用base_convert()函数,语法“bindec(十六进制字符串, 16, 10)”。
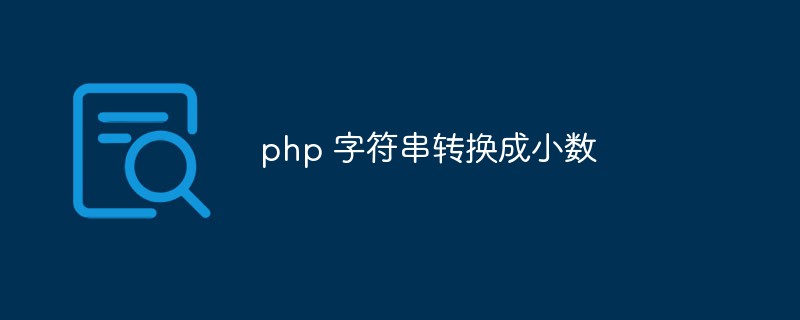
PHP 是一门功能强大的编程语言,广泛应用于 Web 开发领域。其中一个非常常见的情况是需要将字符串转换为小数。这在进行数据处理的时候非常有用。在本文中,我们将介绍如何在 PHP 中将字符串转换为小数。
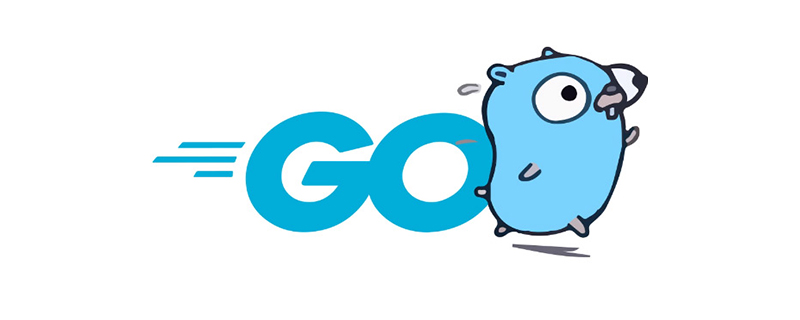
检测变量是否为字符串的方法:1、利用“%T”格式化标识,语法“fmt.Printf("variable count=%v is of type %T \n", count, count)”;2、利用reflect.TypeOf(),语法“reflect.TypeOf(变量)”;3、利用reflect.ValueOf().Kind()检测;4、使用类型断言,可以对类型进行分组。
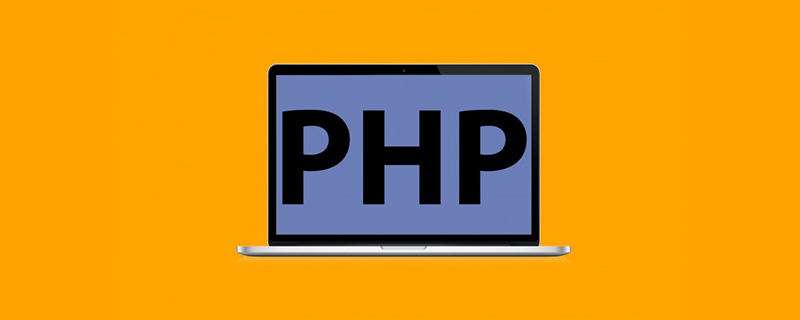
php字符串长度不一致的解决办法:1、通过mb_detect_encoding()函数查看字符串的编码方式;2、通过mb_strlen函数查看具体字符长度;3、使用正则表达式“preg_match_all('/[\x{4e00}-\x{9fff}]+/u', $str1, $matches);”剔除非中文字符即可。
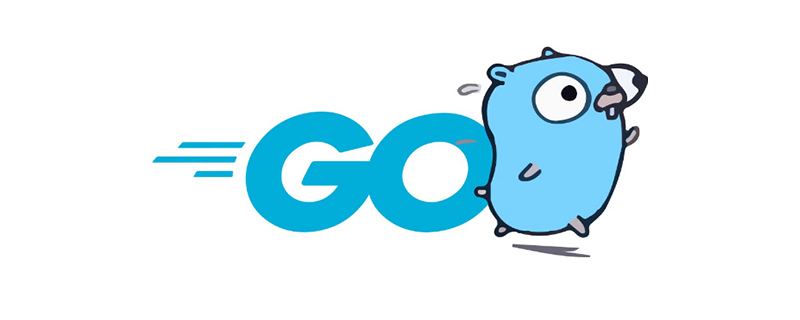
删除方法:1、使用TrimSpace()函数去除字符串左右两边的空格,语法“strings.TrimSpace(str)”;2、使用Trim()函数去除字符串左右两边的空格,语法“strings.Trim(str, " ")”;3、使用Replace()函数去除字符串的全部空格,语法“strings.Replace(str, " ", "", -1)”。
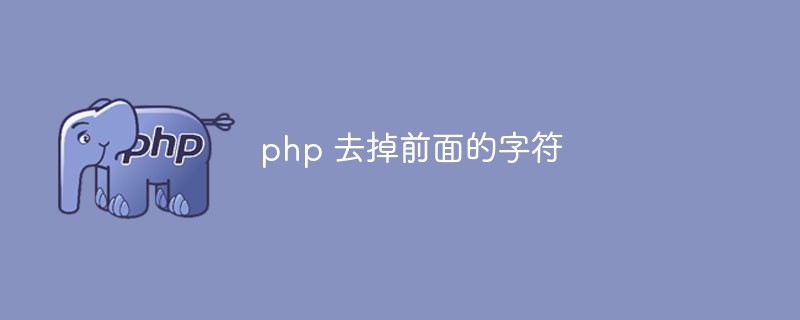
在开发PHP应用程序时,有时我们需要去掉字符串前面的某些特定字符或者字符串。在这种情况下,我们需要使用一些PHP函数来实现这一目标。本文将介绍一些PHP函数,帮助您轻松地去掉字符串前面的字符或字符串。
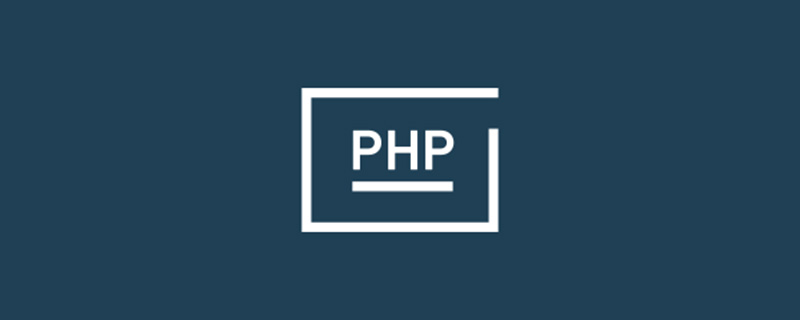
转换方法:1、在转换变量前加上用括号括起来的目标类型“(bool)”或“(boolean)”;2、用boolval()函数,语法“boolval(字符串)”;3、用settype()函数,语法“settype(变量,"boolean")”。
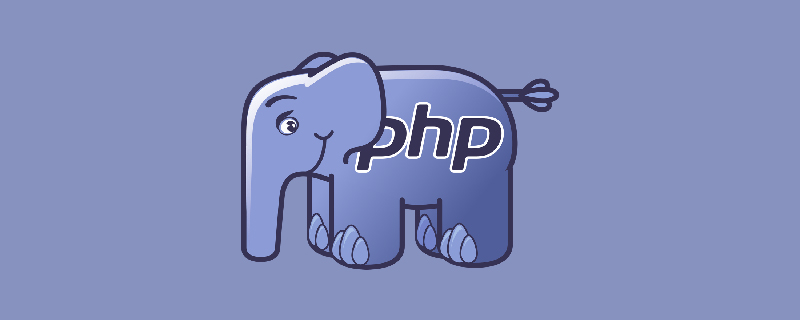
php字符串部分乱码的解决办法:1、使用“mb_substr(strip_tags($str),0,-1,'UTF-8');”截取字符串;2、使用“iconv("UTF-8","GB2312//IGNORE",$data)”转换字符集即可。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
