In the given problem, we need to print all the divisors of a given integer n.
Input: 15 Output: 1 3 5 15 Explanation Divisors of 15 are: 1,3, 5, 15 Input: 30 Output: 1 2 3 5 15 30
In the given problem, we can apply the method used in the sieve of Eratosthenes to find all divisors of n.
Methods to find the solution
In the given method, we will apply the concept of Sieve of Eratosthenes and find the divisors of n.
Example
#include <bits/stdc++.h> #define MOD 1000000007 using namespace std; vector<int> divisors[100001]; // our vector containing number with all of its divisors void findsieve(int max) { // filling data in vector divisors till 10e5 for(int i = 1; i <= max; i++) { for(int j = i; j <= max; j += i) divisors[j].push_back(i); } } void __print(int n){ // the function to print divisors for(auto x : divisors[n]) cout << x << " "; cout << "\n"; } int main() { findsieve(100000); // we hardcode the sieve and divisors till 10e5 int n = 6; // the given n __print(n); n = 30; // new n __print(n); return 0; }
Output
1 2 3 6 1 2 3 5 6 10 15 30
Explanation of the above code
In this method we follow the same concept as Sieve of Eratosthenes . We find the divisor of every number up to 105. We don't need to find the divisor when we receive q queries, so this greatly reduces our time complexity when asking q queries. Therefore, our complexity becomes O(Q*N), where Q is the number of queries we process and N is the number of divisors of n.
Conclusion
In this article, we solved the problem of query printing all divisors of n, where we applied the Sieve of Eratosthenes principle. We also learned a C program to solve this problem and a complete method to solve this problem (Normal). We can write the same program in other languages such as C, java, python and other languages. We hope this article was helpful to you.
The above is the detailed content of Query to print out all factors of n using C++. For more information, please follow other related articles on the PHP Chinese website!
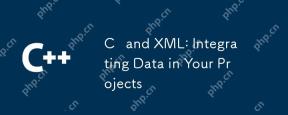
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
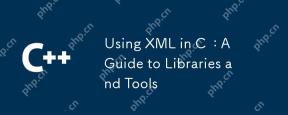
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
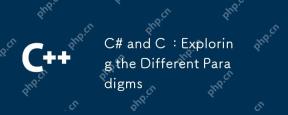
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
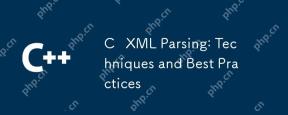
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
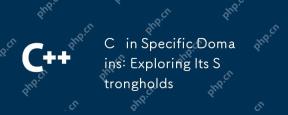
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
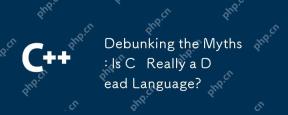
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
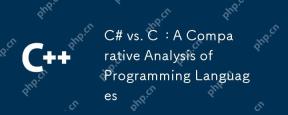
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
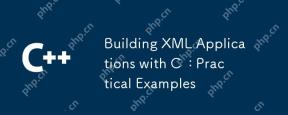
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
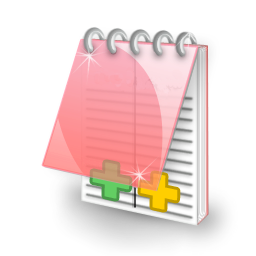
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
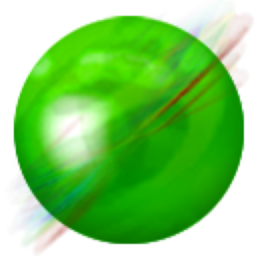
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
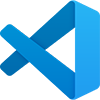
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
