The mouse in the maze problem is one of the well-known backtracking problems. Here we will see that the problem remains almost unchanged. Suppose an NxN maze M is given. The starting point is the upper left corner M[0, 0], and the end point is the lower right corner M[N – 1, N – 1]. A mouse is placed at the starting point. Our goal is to find a path from the starting point to the end point that allows the mouse to reach its destination. Here mice can jump (variant). Now there are some restrictions
- The mouse can move right or down.
- A 0 in a cell in the maze means that the cell is blocked.
- Non-zero cells represent valid paths.
- The number in a cell indicates the maximum number of jumps the rat can make from that cell. ul>
Algorithm
ratInMaze
begin if destination is reached, then print the solution matrix else 1. Place the current cell inside the solution matrix as 1 2. Move forward or jump (check max jump value) and recursively check if move leads to solution or not. 3. If the move taken from the step 2 is not correct, then move down, and check it leads to the solution or not 4. If none of the solutions in step 2 and 3 are correct, then make the current cell 0. end if end
Example
#include <iostream> #define N 4 using namespace std; void dispSolution(int sol[N][N]) { for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) cout << sol[i][j] << " "; cout << endl; } } bool isSafe(int maze[N][N], int x, int y) { //check whether x,y is valid or not // when (x, y) is outside of the maze, then return false if (x >= 0 && x < N && y >= 0 && y < N && maze[x][y] != 0) return true; return false; } bool ratMazeSolve(int maze[N][N], int x, int y, int sol[N][N]) { if (x == N - 1 && y == N - 1) { //if destination is found, return true sol[x][y] = 1; return true; } if (isSafe(maze, x, y)) { sol[x][y] = 1; //mark 1 into solution matrix for (int i = 1; i <= maze[x][y] && i < N; i++) { if (ratMazeSolve(maze, x + i, y, sol)) //move right return true; if (ratMazeSolve(maze, x, y + i, sol)) //move down return true; } sol[x][y] = 0; //if the solution is not valid, then make it 0 return false; } return false; } bool solveMaze(int maze[N][N]) { int sol[N][N] = { { 0, 0, 0, 0 }, { 0, 0, 0, 0 }, { 0, 0, 0, 0 }, { 0, 0, 0, 0 } }; if (!ratMazeSolve(maze, 0, 0, sol)) { cout << "Solution doesn't exist"; return false; } dispSolution(sol); return true; } main() { int maze[N][N] = { { 2, 1, 0, 0 }, { 3, 0, 0, 1 }, { 0, 1, 0, 1 }, { 0, 0, 0, 1 } }; solveMaze(maze); }
Output
1 0 0 0 1 0 0 1 0 0 0 1 0 0 0 1
The above is the detailed content of Can a mouse in a maze make multiple steps or jumps?. For more information, please follow other related articles on the PHP Chinese website!
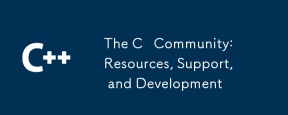
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
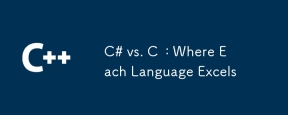
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
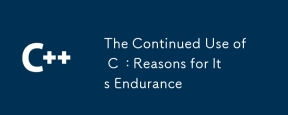
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
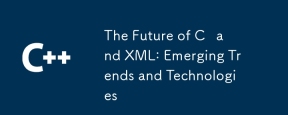
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
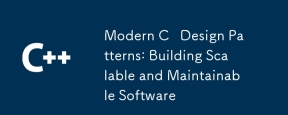
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
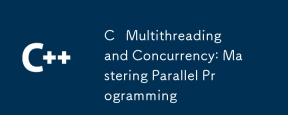
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
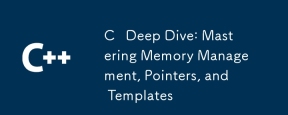
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
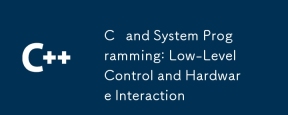
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
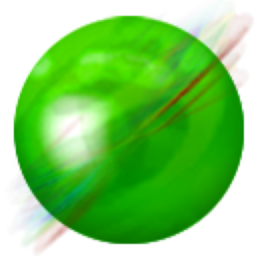
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
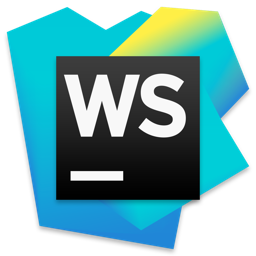
WebStorm Mac version
Useful JavaScript development tools
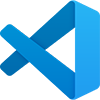
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft