


Java comparator interface for sorting Java objects. Comparator classes in Java compare different objects (Obj 01, Obj 02) by calling "java.util.comparator". In this method, objects can be compared based on the return value. The comparison can be positive, equal, or negative.
This process provides the user with multiple sorting sequences. There are many ways to compare the two methods.
public int compare class (obj 1, obj 2) - Performs a comparison between two objects.
public Boolean equals (obj) - Compares the current object with the specified object.
Java Collection Class - Provides static methods for sorting elements in a data collection. This collection element is used in TreeMap.
Let us discuss how to build a Java code using comparators to search for a user-defined object from a list via binary search.
Binary search parameters and their components
parameter
is a specific array
fromindex - the first element to search for
toindex - the last element to search for key - the value to search for Key-value pairs to be searched
Comparators
return
Returns the index of the search key that exists within the specified range.
exception
ClassCast
Illegal parameter
ArrayIndexOutOfBounds
algorithm
Step one - start.
Step 2 - Intermediate element set calculation.
Step 3 - Compare the keyword to the middle element.
Step 4 - If the values of key and mid elements are the same; then return the result.
Step 5 - Otherwise, the value of the key is greater than the middle element, then follow the right half of the collection
Step 6 - Or; if the value of key is less than the mid element then follow upper
Binary search using comparator - syntax
public static int binarySearch(primitive() p,Primitive key) public static int binarySearch(Object() o,Object key) public static int binarySearch(Object() o,Object key,Comparator c) Java Collections binarySearch(List<? extends Comparable1<? super R>> list, R key)and; Java Collections binarySearch(List<? extends R> list, R key, Comparator<? super R> c)
There are two well-known syntaxes to search for user-defined objects from lists via binary search using comparators. For the first case, the list needs to be sorted in ascending order and the procedure is called using a specific method, where the result is undefined.
On the other hand, to search for a specified object, it is important to call the method.
Following method
Method 1 to search for a user-defined object from a list by using a binary searcher and comparator
Search for user-defined objects from a list using a comparator
In these examples, we use collections, binarySearch() and comparator class operations to sort some user-defined data using the binary search operation through the comparator
Example 1: Use Collections, binarySearch() to find data from a list
import java.util.*; public class Binarysearch { public static void main(String[] args){ List<Domain> l1 = new ArrayList<Domain>(); l1.add(new Domain(100, "India")); l1.add(new Domain(200, "Bangladesh")); l1.add(new Domain(300, "Dhaka")); l1.add(new Domain(400, "Kolkata")); Comparator<Domain> c = new Comparator<Domain>() { public int compare(Domain u1, Domain u2) { return u1.getId().compareTo(u2.getId()); } }; int index = Collections.binarySearch( l1, new Domain(10, null), c); System.out.println("Found at index number zone" + index); index = Collections.binarySearch(l1, new Domain(6, null), c); System.out.println(index); } } class Domain { private int id; private String url; public Domain(int id, String url){ this.id = id; this.url = url; } public Integer getId() { return Integer.valueOf(id); } }
Output
Found at index number zone-1 -1
Example 2: Sort the list in ascending order
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class ascendingsearch { public static void main(String[] args){ List<Integer> ak = new ArrayList<integer>(); ak.add(100); ak.add(200); ak.add(30); ak.add(10); ak.add(20); int index = Collections.binarySearch(ak, 100); System.out.println(index); index = Collections.binarySearch(ak, 130); System.out.println(index); } } </integer>
Output
Note: ascendingsearch.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. -6 -6
Example 3: Sort the list in descending order and find the index number
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class binsearchdecend { public static void main(String[] args){ List<Integer> a0710 = new ArrayList<Integer>(); a0710.add(1000); a0710.add(500); a0710.add(300); a0710.add(10); a0710.add(2); int index = Collections.binarySearch( a0710, 50, Collections.reverseOrder()); System.out.println("Found at index number present " + index); } }
Output
Found at index number present -4
Example 4: Find the number of elements and values
import java.util.Scanner; public class BinarySearchExample{ public static void main(String args[]){ int counter, num, item, array[], first, last, middle; Scanner input = new Scanner(System.in); System.out.println("Enter number of elements:"); num = input.nextInt(); array = new int[num]; System.out.println("Enter " + num + " integers"); for (counter = 0; counter < num; counter++) array[counter] = input.nextInt(); System.out.println("Enter the search value:"); item = input.nextInt(); first = 0; last = num - 1; middle = (first + last)/2; while( first <= last ){ if ( array[middle] < item ) first = middle + 1; else if ( array[middle] == item ){ System.out.println(item + " found at location " + (middle + 1) + "."); break; } else{ last = middle - 1; } middle = (first + last)/2; } if ( first > last ) System.out.println(item + " is not found.\n"); } }
Output
Enter number of elements: 7 Enter 7 integers 10 12 56 42 48 99 100 Enter the search value: 50 50 is not found.
in conclusion
In this article, we learned about the Comparable interface in Java through some sample codes and algorithms. Here we declare some user-defined classes and comparator interfaces. They serve some specific purposes and allow specific data to be processed in a Java environment.
The above is the detailed content of Java program to search user defined object from list by using binary search comparator. For more information, please follow other related articles on the PHP Chinese website!
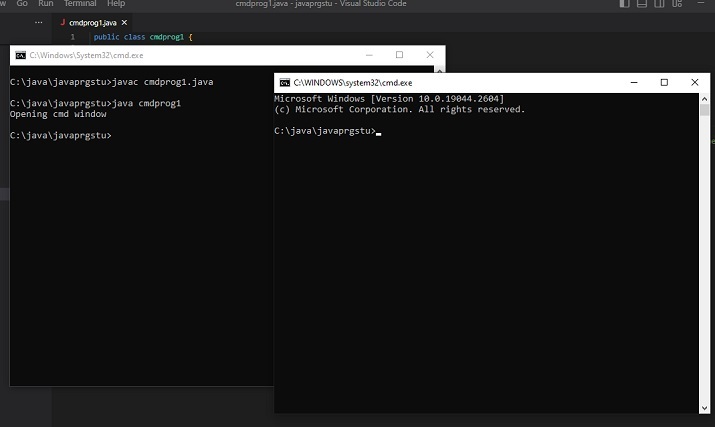
ThisarticleusesvariousapproachesforselectingthecommandsinsertedintheopenedcommandwindowthroughtheJavacode.Thecommandwindowisopenedbyusing‘cmd’.Here,themethodsofdoingthesamearespecifiedusingJavacode.TheCommandwindowisfirstopenedusingtheJavaprogram.Iti
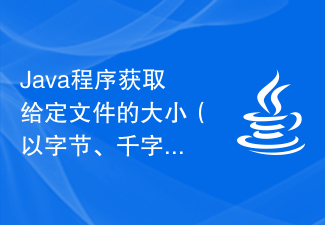
文件的大小是特定文件在特定存储设备(例如硬盘驱动器)上占用的存储空间量。文件的大小以字节为单位来衡量。在本节中,我们将讨论如何实现一个java程序来获取给定文件的大小(以字节、千字节和兆字节为单位)。字节是数字信息的最小单位。一个字节等于八位。1千字节(KB)=1,024字节1兆字节(MB)=1,024KB千兆字节(GB)=1,024MB和1太字节(TB)=1,024GB。文件的大小通常取决于文件的类型及其包含的数据量。以文本文档为例,文件的大小可能只有几千字节,而高分辨率图像或视频文件的大小可
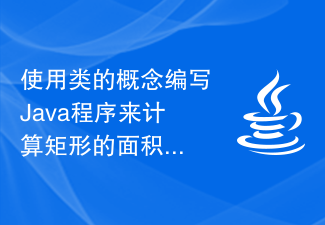
Java语言是当今世界上最常用的面向对象编程语言之一。类的概念是面向对象语言中最重要的特性之一。一个类就像一个对象的蓝图。例如,当我们想要建造一座房子时,我们首先创建一份房子的蓝图,换句话说,我们创建一个显示我们将如何建造房子的计划。根据这个计划,我们可以建造许多房子。同样地,使用类,我们可以创建许多对象。类是创建许多对象的蓝图,其中对象是真实世界的实体,如汽车、自行车、笔等。一个类具有所有对象的特征,而对象具有这些特征的值。在本文中,我们将使用类的概念编写一个Java程序,以找到矩形的周长和面
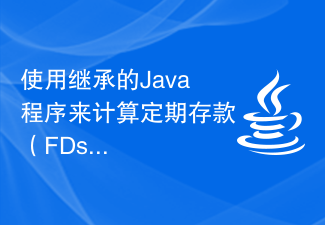
继承是一个概念,它允许我们从一个类访问另一个类的属性和行为。被继承方法和成员变量的类被称为超类或父类,而继承这些方法和成员变量的类被称为子类或子类。在Java中,我们使用“extends”关键字来继承一个类。在本文中,我们将讨论使用继承来计算定期存款和定期存款的利息的Java程序。首先,在您的本地机器IDE中创建这四个Java文件-Acnt.java−这个文件将包含一个抽象类‘Acnt’,用于存储账户详情,如利率和金额。它还将具有一个带有参数‘amnt’的抽象方法‘calcIntrst’,用于计
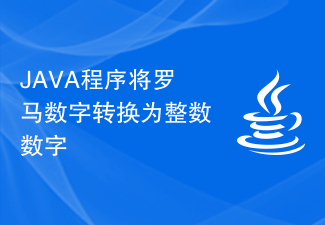
罗马数字-基于古罗马系统,使用符号来表示数字。这些数字称为罗马数字。符号为I、V、X、L、C、D和M,分别代表1、5、10、50、100、500和1,000。整数-整数就是由正值、负值和零值组成的整数。分数不是整数。这里我们根据整数值设置符号值。每当输入罗马数字作为输入时,我们都会将其划分为单位,然后计算适当的罗马数字。I-1II–2III–3IV–4V–5VI–6...X–10XI–11..XV-15在本文中,我们将了解如何在Java中将罗马数字转换为整数。向您展示一些实例-实例1InputR
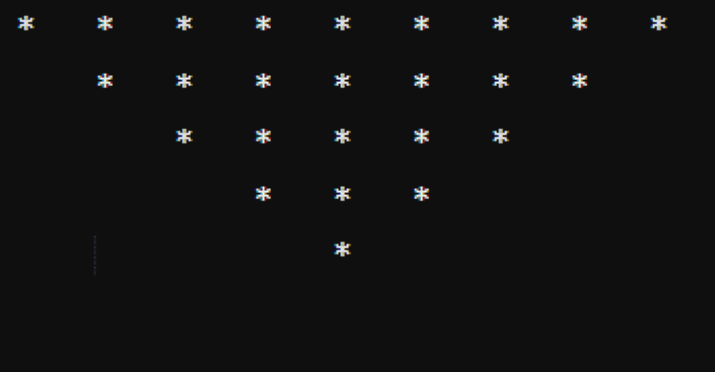
如果有人想在Java编程语言方面打下坚实的基础。然后,有必要了解循环的工作原理。此外,解决金字塔模式问题是增强Java基础知识的最佳方法,因为它包括for和while循环的广泛使用。本文旨在提供一些Java程序,借助Java中可用的不同类型的循环来打印金字塔图案。创建金字塔图案的Java程序我们将通过Java程序打印以下金字塔图案-倒星金字塔星金字塔数字金字塔让我们一一讨论。模式1:倒星金字塔方法声明并初始化一个指定行数的整数“n”。接下来,将空间的初始计数定义为0,将星形的初始计数定义为“n+
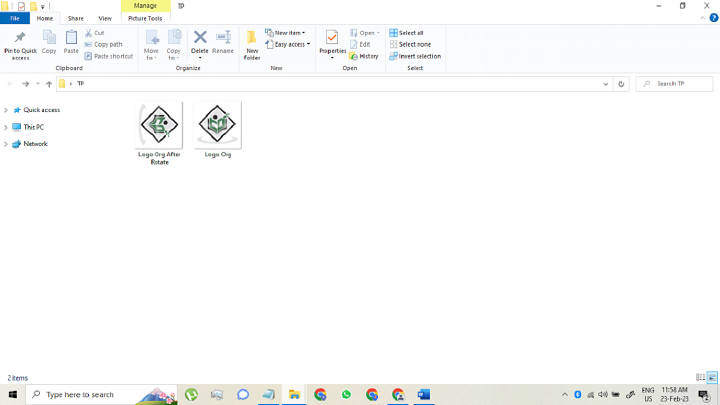
一个图像文件可以顺时针或逆时针旋转。要旋转图像,需要下载一个随机的图像文件并将其保存在系统的任何文件夹中。此外,需要一个.pdf文件,在打开下载的图像文件后,可以在该特定的.pdf文件中旋转一些角度。对于90度的旋转,新图像的锚点可以帮助我们使用Java中的平移变换执行旋转操作。锚点是任何特定图像的中心。AlgorithmtoRotateanImagebyUsingJavaThe"AffineTransformOp"classisthesimplestwaytorotatea
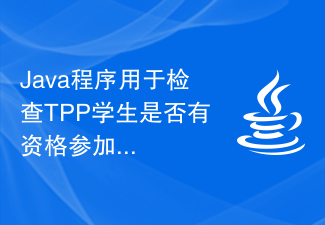
请考虑下表了解不同公司的资格标准-CGPA的中文翻译为:绩点平均成绩符合条件的公司大于或等于8谷歌、微软、亚马逊、戴尔、英特尔、Wipro大于或等于7教程点、accenture、Infosys、Emicon、Rellins大于或等于6rtCamp、Cybertech、Skybags、Killer、Raymond大于或等于5Patronics、鞋子、NoBrokers让我们进入java程序来检查tpp学生参加面试的资格。方法1:使用ifelseif条件通常,当我们必须检查多个条件时,我们会使用


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
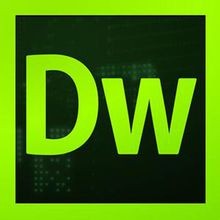
Dreamweaver CS6
Visual web development tools
