Introduction
OpenCV (Open Source Computer Vision Library) is an open source computer vision and machine learning software library. It contains more than 2500 optimization algorithms and is widely used in real-time applications.
Java provides bindings to OpenCV through the JavaCV library, allowing Java developers to leverage the capabilities of OpenCV in their applications. One such application is capturing images from webcams.
prerequisites
To continue, you need the following -
OpenCV is already installed on your system.
JavaCV, a wrapper for OpenCV in Java.
Capturing snapshots using OpenCV
To capture a snapshot, we need to create an instance of the VideoCapture class, which represents the video capture device. We can then use the read method to capture frames from the video source.
Example
Let’s see an example -
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.videoio.VideoCapture; import org.opencv.imgcodecs.Imgcodecs; public class Main { static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); } public static void main(String[] args) { // Create a VideoCapture object VideoCapture camera = new VideoCapture(0); // Check if camera opened successfully if(!camera.isOpened()){ System.out.println("Error: Camera not accessible"); return; } // Capture a new frame Mat frame = new Mat(); camera.read(frame); // Save the frame as an image Imgcodecs.imwrite("snapshot.png", frame); // Release the camera camera.release(); } }
illustrate
In the above code, we first load the OpenCV library.
Then we create a VideoCapture object for the default camera (index 0). If you have multiple cameras and want to use a different one, you can specify its index when you create the VideoCapture object.
We use the isOpened method to check whether the camera is successfully opened. If the camera is inaccessible, we print an error message and return from the main method.
Next, we use the read method to capture a frame from the camera and store it in the Mat object. Mat (short for Matrix) is the main image structure in OpenCV used to store pixel data.
We use the imwrite method in the Imgcodecs class to save the captured frame as an image and name it "snapshot.png".
Finally, we use the release method to release the camera to release resources.
When you run this program, it will capture a single frame from the webcam and save it as "snapshot.png" in the project directory.
in conclusion
Capturing images from a webcam in Java using OpenCV is a simple process that opens up numerous opportunities for more complex applications such as face recognition, motion detection, etc. Understanding how to use the OpenCV library in Java provides a solid foundation for delving into computer vision and image processing.
The above is the detailed content of Taking snapshot from system camera using OpenCV in Java. For more information, please follow other related articles on the PHP Chinese website!
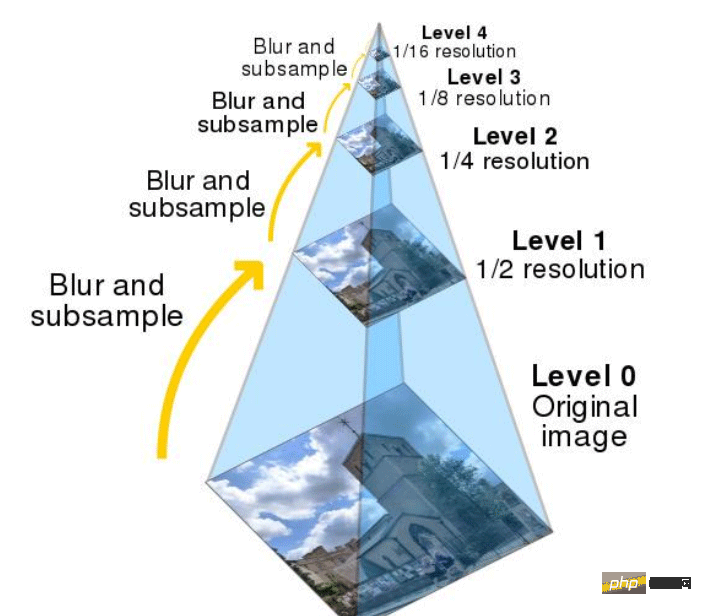
1.图像金字塔理论基础图像金字塔是图像多尺度表达的一种,是一种以多分辨率来解释图像的有效但概念简单的结构。一幅图像的金字塔是一系列以金字塔形状排列的分辨率逐步降低,且来源于同一张原始图的图像集合。其通过梯次向下采样获得,直到达到某个终止条件才停止采样。我们将一层一层的图像比喻成金字塔,层级越高,则图像越小,分辨率越低。那我们为什么要做图像金字塔呢?这就是因为改变像素大小有时候并不会改变它的特征,比方说给你看1000万像素的图片,你能知道里面有个人,给你看十万像素的,你也能知道里面有个人,但是对计
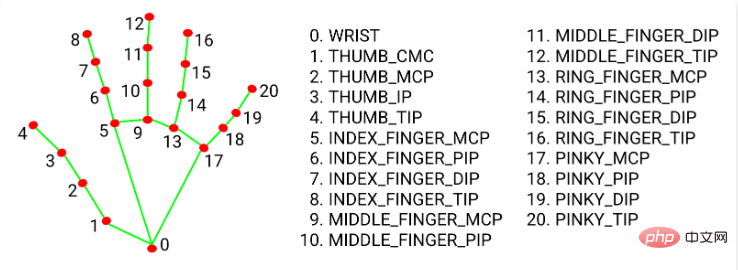
一、项目效果二、核心流程1、openCV读取视频流、在每一帧图片上画一个矩形。2、使用mediapipe获取手指关键点坐标。3、根据手指坐标位置和矩形的坐标位置,判断手指点是否在矩形上,如果在则矩形跟随手指移动。三、代码流程环境准备:python:3.8.8opencv:4.2.0.32mediapipe:0.8.10.1注:1、opencv版本过高或过低可能出现一些如摄像头打不开、闪退等问题,python版本影响opencv可选择的版本。2、pipinstallmediapipe后可能导致op
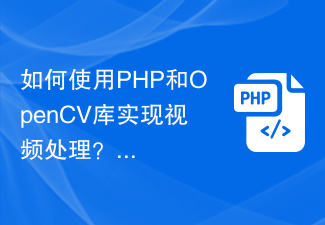
如何使用PHP和OpenCV库实现视频处理?摘要:在现代科技应用中,视频处理已经成为一项重要的技术。本文将介绍如何使用PHP编程语言结合OpenCV库来实现一些基本的视频处理功能,并附上相应的代码示例。关键词:PHP、OpenCV、视频处理、代码示例引言:随着互联网的发展和智能手机的普及,视频内容已经成为人们生活中不可或缺的一部分。然而,要想实现视频的编辑和
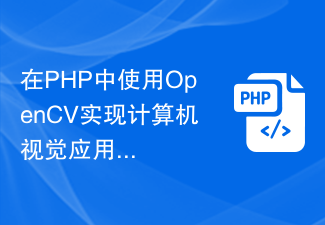
计算机视觉(ComputerVision)是人工智能领域的重要分支之一,它可以使计算机能够自动地感知和理解图像、视频等视觉信号,实现人机交互以及自动化控制等应用场景。OpenCV(OpenSourceComputerVisionLibrary)是一个流行的开源计算机视觉库,在计算机视觉、机器学习、深度学习等领域都有广泛的应用。本文将介绍在PHP中使
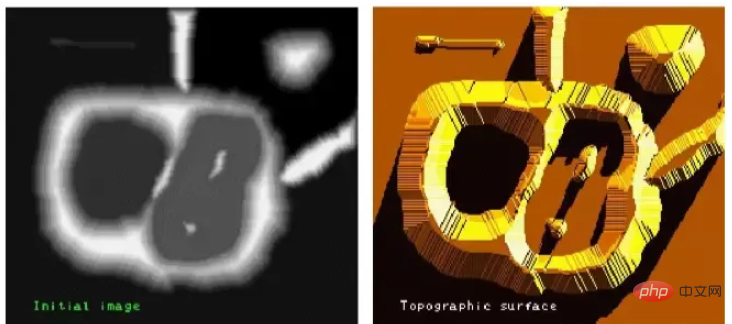
图像分割与提取图像中将前景对象作为目标图像分割或者提取出来。对背景本身并无兴趣分水岭算法及GrabCut算法对图像进行分割及提取。用分水岭算法实现图像分割与提取分水岭算法将图像形象地比喻为地理学上的地形表面,实现图像分割,该算法非常有效。算法原理任何一幅灰度图像,都可以被看作是地理学上的地形表面,灰度值高的区域可以被看成是山峰,灰度值低的区域可以被看成是山谷。左图是原始图像,右图是其对应的“地形表面”。该过程将图像分成两个不同的集合:集水盆地和分水岭线。我们构建的堤坝就是分水岭线,也即对原始图像
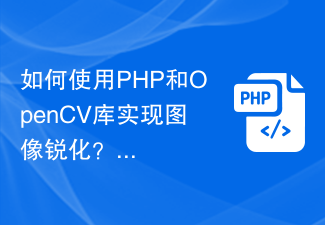
如何使用PHP和OpenCV库实现图像锐化?概述:图像锐化是一种常见的图像处理技术,用于提高图像的清晰度和边缘的强度。在本文中,我们将介绍如何使用PHP和OpenCV库来实现图像锐化。OpenCV是一款功能强大的开源计算机视觉库,它提供了丰富的图像处理功能。我们将使用OpenCV的PHP扩展来实现图像锐化算法。步骤1:安装OpenCV和PHP扩展首先,我们需
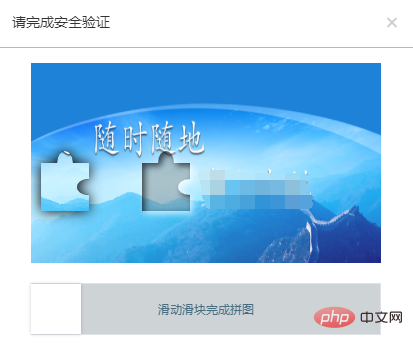
1、滑块验证思路被测对象的滑块对象长这个样子。相对而言是比较简单的一种形式,需要将左侧的拼图通过下方的滑块进行拖动,嵌入到右侧空槽中,即完成验证。要自动化完成这个验证过程,关键点就在于确定滑块滑动的距离。根据上面的分析,验证的关键点在于确定滑块滑动的距离。但是看似简单的一个需求,完成起来却并不简单。如果使用自然逻辑来分析这个过程,可以拆解如下:1.定位到左侧拼图所在的位置,由于拼图的形状和大小固定,那么其实只需要定位其左边边界离背景图片的左侧距离。(实际在本例中,拼图的起始位置也是固定的,节省了
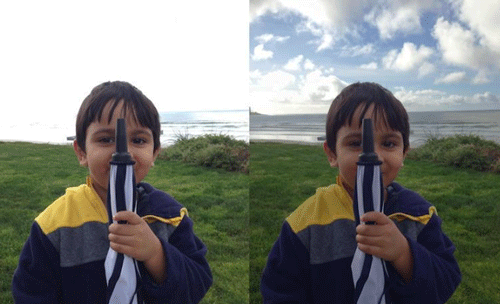
1背景1.1什么是高动态范围(HDR)成像?大多数数码相机和显示器将彩色图像捕获或显示为24位矩阵。每个颜色通道有8位,一共三个通道,因此每个通道的像素值在0到255之间。换句话说,普通相机或显示器具有有限的动态范围。然而,我们周围的世界颜色有一个非常大的变化范围。当灯关闭时,车库会变黑;太阳照射下,车库看起来变得非常明亮。即使不考虑这些极端情况,在日常情况下,8位也几乎不足以捕捉场景。因此,相机会尝试估计光线并自动设置曝光,以使图像中最有用的部分具有良好的动态颜色范围,而太暗和太亮的部分分别被


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
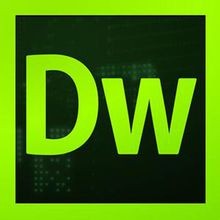
Dreamweaver CS6
Visual web development tools
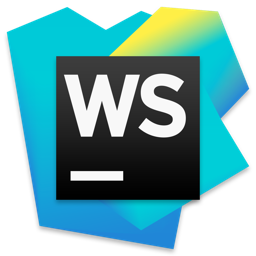
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor
