How to solve C++ runtime error: 'pointer is uninitialized'?
How to solve C runtime error: 'pointer is uninitialized'?
In C programming, various runtime errors are often encountered. One of the common errors is 'pointer is uninitialized', which is the error that the pointer is not initialized. This article will describe the cause and solution of this error, and provide corresponding code examples.
In C, a pointer is a variable that stores a memory address. When we use a pointer, we need to ensure that it points to a valid memory address, otherwise undefined behavior will occur. If a pointer is not allocated or initialized before being used, the 'pointer is uninitialized' error will occur.
There are many reasons why the pointer is not initialized. Here are a few common situations:
-
Declaring the pointer but not allocating memory for it:
int* ptr;
-
The object pointed to by the pointer was destroyed before declaration:
int* ptr; { int value = 10; ptr = &value; } // 代码块结束时,value对象被销毁
-
The pointer was copied or assigned to another pointer, and the other pointer was not initialized:
int* ptr1; int* ptr2 = ptr1;
The solution to this problem is to ensure that the pointer is initialized or points to a valid memory address before use. The following are several common solutions:
-
Use the new keyword to allocate memory for the pointer:
int* ptr = new int; *ptr = 10;
-
Initialize the pointer to null:
int* ptr = nullptr;
-
If an object has been declared before declaring the pointer, you can point the pointer to the object:
int value = 10; int* ptr = &value;
- Be careful to avoid copying uninitialized pointers to other objects. pointer.
Here is a complete example showing how to solve 'pointer is uninitialized' error in C:
#include <iostream> int main() { int* ptr = nullptr; // 初始化指针为null ptr = new int; // 为指针分配内存 *ptr = 10; // 写入int型对象的值 std::cout << *ptr << std::endl; // 输出10 delete ptr; // 释放内存 return 0; }
In this example, we first initialize the pointer ptr to nullptr , and then use the new keyword to allocate memory for it. Next, we write a value at the memory address pointed to by the pointer and print the result. Finally, we use the delete keyword to free the previously allocated memory.
To summarize, there are many ways to solve the 'pointer is uninitialized' error in C. The most common is to use new to allocate memory for the pointer or initialize the pointer to null. During the programming process, be sure to initialize pointers correctly to avoid undefined behavior.
The above is the detailed content of How to solve C++ runtime error: 'pointer is uninitialized'?. For more information, please follow other related articles on the PHP Chinese website!
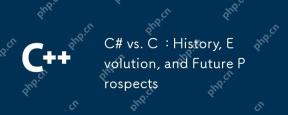
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
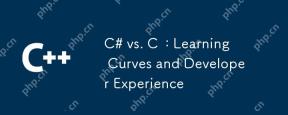
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
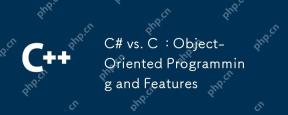
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
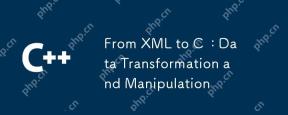
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
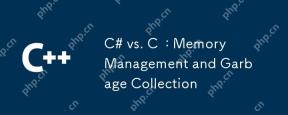
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
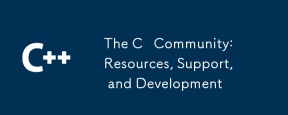
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
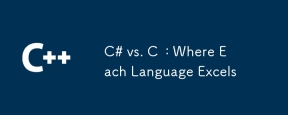
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
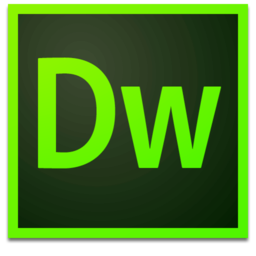
Dreamweaver Mac version
Visual web development tools
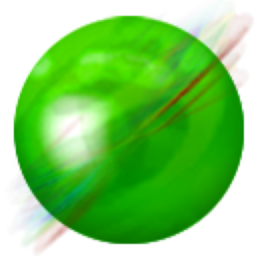
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
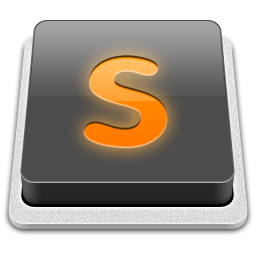
SublimeText3 Mac version
God-level code editing software (SublimeText3)