


Baidu AI interface and Golang: implementing emotional analysis and making applications more intelligent
Introduction:
In recent years, with the rapid development of artificial intelligence, emotional analysis As one of the important applications of natural language processing, it is widely used in social media monitoring, public opinion analysis, emotion recognition and other fields. Baidu AI interface provides powerful sentiment analysis capabilities. Combined with the efficient performance of Golang language, it can achieve fast and accurate sentiment analysis and add intelligent functions to applications. This article will introduce how to use Baidu AI interface and Golang language to implement sentiment analysis, and give code examples.
1. Overview of Baidu AI interface
Baidu AI interface is a series of artificial intelligence capabilities provided by Baidu Intelligent Cloud, including sentiment analysis, speech recognition, image recognition, etc. This article will focus on the use of sentiment analysis interfaces.
Baidu sentiment analysis interface is a technology that analyzes text content to determine its emotional tendency. It can make positive, negative and neutral emotional judgments on texts and give corresponding emotional probabilities.
2. Characteristics of Golang language
Golang is a modern and efficient programming language with strong concurrency performance, static type checking, garbage collection and other characteristics. It is suitable for developing high-performance applications.
3. Use Baidu AI interface to implement sentiment analysis
- Building development environment
First, you need to install the Golang language environment and apply for an API Key on Baidu Smart Cloud. Use To call the sentiment analysis interface. - Introduce necessary libraries
In Golang, you can use thenet/http
library to perform HTTP request operations. This library needs to be introduced in the code.
import ( "net/http" "io/ioutil" "encoding/json" )
- Send a request and parse the returned results
Use the HTTP POST method to send a request to the Baidu AI interface, and pass the text that needs sentiment analysis as a parameter. Parse the returned results.
func SentimentAnalysis(text string) (string, error) { url := "https://aip.baidubce.com/rpc/2.0/nlp/v1/sentiment_classify" // 拼接请求参数 data := map[string]interface{}{ "text": text, } jsonStr, _ := json.Marshal(data) req, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonStr)) if err != nil { return "", err } req.Header.Set("Content-Type", "application/json") req.Header.Set("Charset", "UTF-8") // 设置API Key q := req.URL.Query() q.Add("access_token", "YOUR_API_KEY") req.URL.RawQuery = q.Encode() client := http.Client{} resp, err := client.Do(req) if err != nil { return "", err } defer resp.Body.Close() body, _ := ioutil.ReadAll(resp.Body) type Result struct { Item struct { PositiveProb float64 `json:"positive_prob"` NegativeProb float64 `json:"negative_prob"` Confidence float64 `json:"confidence"` } `json:"items"` } var result Result err = json.Unmarshal(body, &result) if err != nil { return "", err } // 根据情感概率判断情感倾向 if result.Item.PositiveProb > result.Item.NegativeProb { return "positive", nil } else if result.Item.PositiveProb < result.Item.NegativeProb { return "negative", nil } else { return "neutral", nil } }
4. Sample code and running results
The following is a sample code that implements the sentiment analysis function in the application.
func main() { text := "这家餐馆的服务非常好,菜品也很美味。" result, err := SentimentAnalysis(text) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Sentiment Analysis Result:", result) } }
Running results:
Sentiment Analysis Result: positive
5. Summary
This article introduces how to use Baidu AI interface and Golang language to implement sentiment analysis, and gives code examples. In this way, we can take advantage of the powerful functions provided by Baidu AI interface to add intelligent sentiment analysis capabilities to applications. I hope this article helps you understand and apply sentiment analysis. If you have other needs or more questions, please consult the documentation of Baidu AI interface for in-depth study.
The above is the detailed content of Baidu AI interface and Golang: implement sentiment analysis and make applications more intelligent. For more information, please follow other related articles on the PHP Chinese website!
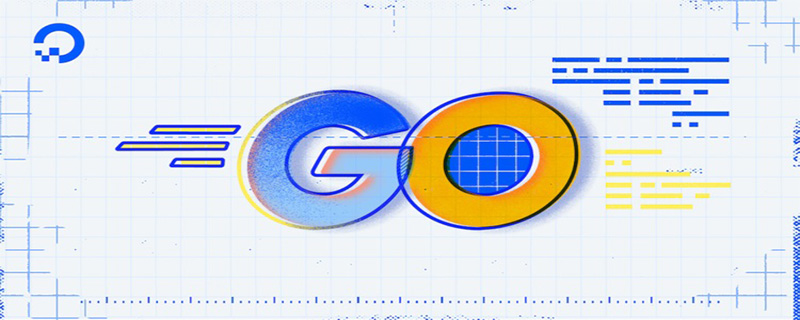
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
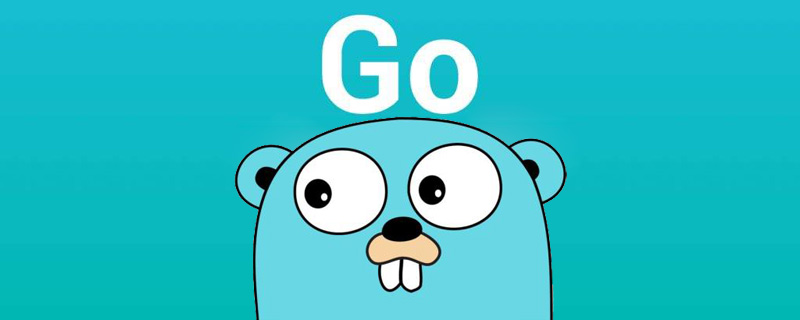
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
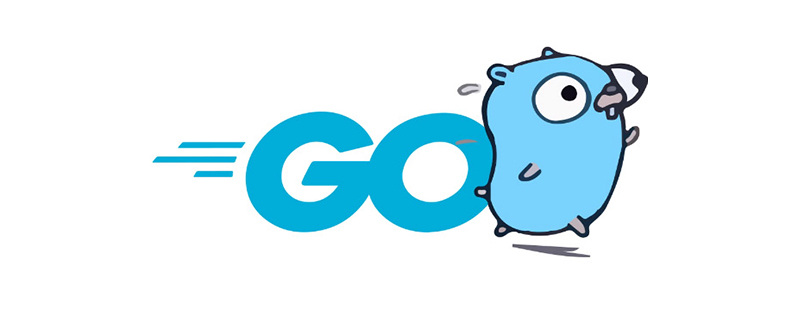
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
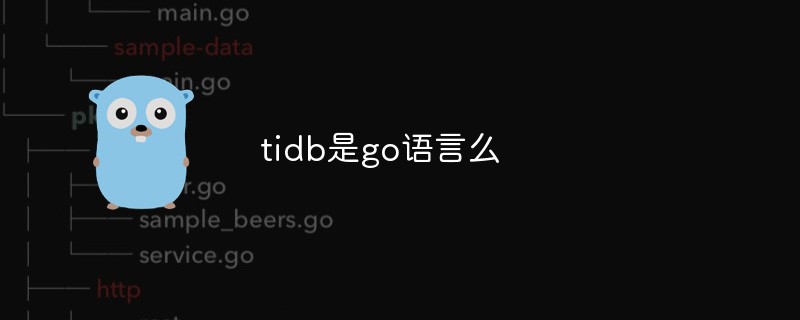
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
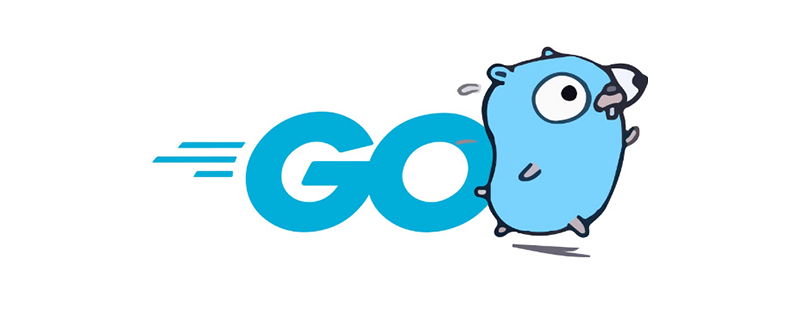
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
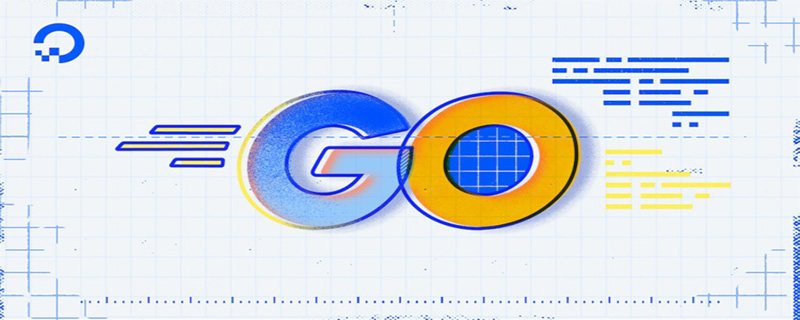
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
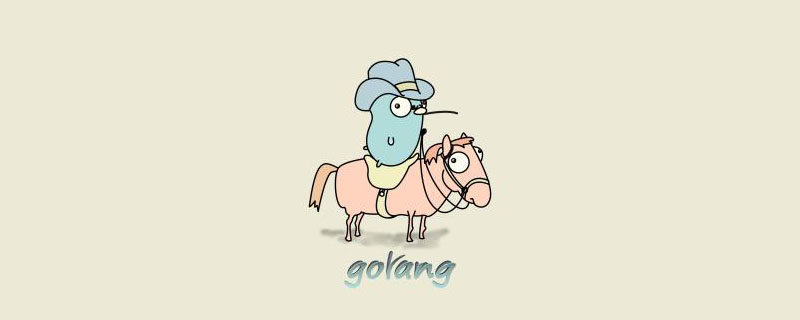
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
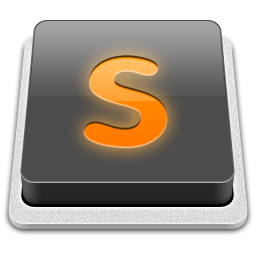
SublimeText3 Mac version
God-level code editing software (SublimeText3)
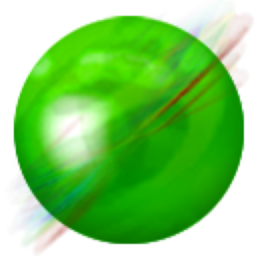
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
