How to use C++ to implement efficient algorithms and data processing?
How to use C to achieve efficient algorithms and data processing?
C is a powerful and widely used programming language that can be used to implement various complex algorithms and efficient data processing. In this article, we will explore some ways to improve the efficiency of C programs and how to implement efficient algorithms and data processing.
- Use appropriate data structures
Choosing appropriate data structures is crucial for efficient algorithms and data processing. C provides a variety of built-in data structures, such as arrays, vectors, linked lists, queues, stacks, etc. Choosing the most appropriate data structure according to actual needs can greatly improve the efficiency of the program.
For example, if you need to insert and delete data frequently, you can choose a linked list instead of an array. If you need to access and modify data efficiently, you can choose vectors or arrays.
The following is a sample code implemented using vectors to implement an algorithm for quickly searching for specified elements:
#include <iostream> #include <vector> int main() { std::vector<int> nums = {1, 2, 3, 4, 5}; int target = 3; bool found = false; for (int num : nums) { if (num == target) { found = true; break; } } if (found) { std::cout << "找到了目标元素" << std::endl; } else { std::cout << "未找到目标元素" << std::endl; } return 0; }
- Use an appropriate algorithm
When implementing the algorithm, choose the appropriate The algorithm can greatly improve the efficiency of the program. The C standard library provides many efficient algorithms, such as sorting, searching, merging, etc. Proper use of these algorithms can greatly reduce the workload of writing code while improving program performance.
For example, if you need to sort an array, you can directly use the sort function in the standard library instead of implementing the sorting algorithm yourself. The following is a sample code for sorting using the sort function:
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> nums = {4, 2, 1, 3, 5}; std::sort(nums.begin(), nums.end()); for (int num : nums) { std::cout << num << " "; } std::cout << std::endl; return 0; }
- Avoid unnecessary data copying
Data copying is a very time-consuming operation, especially when processing large amounts of data. To be obvious. In order to improve the efficiency of the program, unnecessary data copying should be avoided as much as possible.
A common situation is function parameter passing. If a function needs to modify the parameters passed in, it can declare the parameters as references or pointers to avoid data copying. If the function does not need to modify the parameters passed in, the parameters can be declared as constant references to avoid data copying and modification.
The following is a sample code using reference passing:
#include <iostream> #include <vector> void modifyVector(std::vector<int>& nums) { nums.push_back(10); } int main() { std::vector<int> nums = {1, 2, 3, 4, 5}; modifyVector(nums); for (int num : nums) { std::cout << num << " "; } std::cout << std::endl; return 0; }
By declaring the parameters as references, the incoming vector can be modified directly in the function, avoiding unnecessary data copying.
- Use bit operations as much as possible
Bit operations are a very efficient operation that can process multiple data in one calculation. In C, bit operations can be used to optimize code efficiency.
For example, use bit operations to determine whether an integer is even:
#include <iostream> bool isEven(int num) { return (num & 1) == 0; } int main() { int num1 = 4; int num2 = 5; std::cout << num1 << (isEven(num1) ? "是偶数" : "不是偶数") << std::endl; std::cout << num2 << (isEven(num2) ? "是偶数" : "不是偶数") << std::endl; return 0; }
By using bitwise AND operations to compare with 1, you can determine whether an integer is even, avoiding the need for Performance cost of using remainder operation.
In summary, by choosing appropriate data structures and algorithms, avoiding unnecessary data copying, and using bit operations and other methods, efficient algorithms and data processing can be achieved in C. Reasonable application of these methods can improve the efficiency of the program and make the program run faster and more stably.
The above is the detailed content of How to use C++ to implement efficient algorithms and data processing?. For more information, please follow other related articles on the PHP Chinese website!
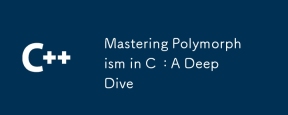
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
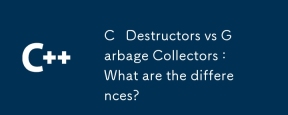
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
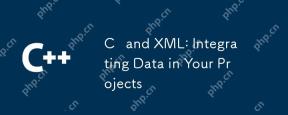
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
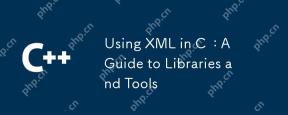
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
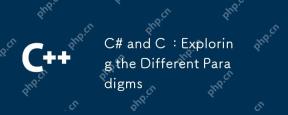
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
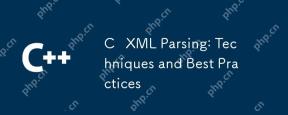
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
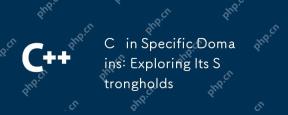
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
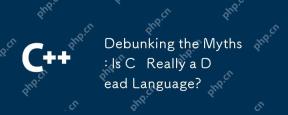
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
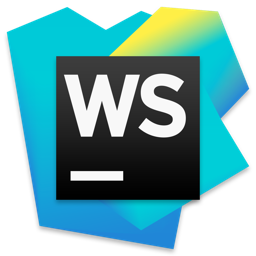
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
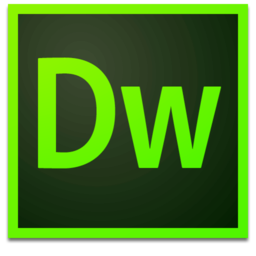
Dreamweaver Mac version
Visual web development tools
