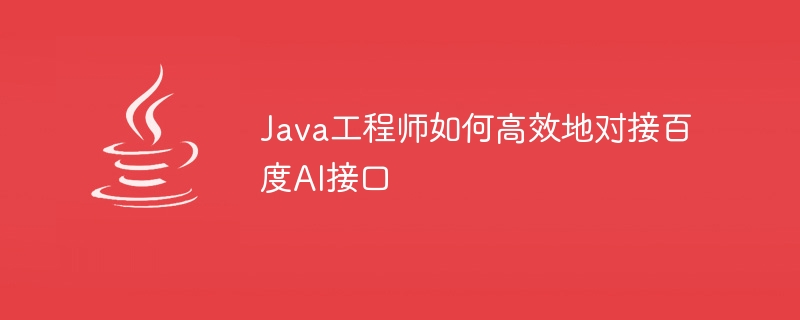
How Java engineers can efficiently connect to Baidu AI interface
- Introduction
Nowadays, artificial intelligence plays an important role in various industries. Baidu, as one of China's leading Internet companies, has launched a series of AI interfaces, including speech recognition, image recognition, natural language processing, etc. For Java engineers, how to efficiently connect to Baidu AI interface will make our applications more intelligent. This article will introduce in detail how to use Baidu AI interface and give corresponding code examples.
- Preparation
Before using the Baidu AI interface, we first need to apply for a Baidu developer account and create a new application to obtain the API Key and Secret Key we need.
- Speech Recognition API
The speech recognition API can convert audio files into text. First, we need to import the corresponding dependent libraries and use the interface to call them in Java. The following is a simple sample code:
import java.io.File;
import java.io.IOException;
import com.baidu.aip.speech.AipSpeech;
import org.json.JSONObject;
public class Sample {
// 设置APPID/AK/SK
public static final String APP_ID = "your_app_id";
public static final String API_KEY = "your_api_key";
public static final String SECRET_KEY = "your_secret_key";
public static void main(String[] args) {
// 初始化一个AipSpeech
AipSpeech client = new AipSpeech(APP_ID, API_KEY, SECRET_KEY);
// 调用API进行语音识别
JSONObject res = client.asr("test.pcm", "pcm", 16000, null);
System.out.println(res.toString(2));
}
}
- Image Recognition API
Image Recognition API can identify image tags. We also need to import the corresponding dependent libraries and use Java calls API. The following is a simple sample code:
import com.baidu.aip.imageclassify.AipImageClassify;
import org.json.JSONObject;
public class Sample {
// 设置APPID/AK/SK
public static final String APP_ID = "your_app_id";
public static final String API_KEY = "your_api_key";
public static final String SECRET_KEY = "your_secret_key";
public static void main(String[] args) {
// 初始化一个AipImageClassify
AipImageClassify client = new AipImageClassify(APP_ID, API_KEY, SECRET_KEY);
// 调用API进行图像识别
String path = "test.jpg";
JSONObject res = client.advancedGeneral(path, new HashMap<String, String>());
System.out.println(res.toString(2));
}
}
- Natural Language Processing API
The Natural Language Processing API can perform word segmentation, part-of-speech tagging, and sentiment analysis on text. The following is a simple sample code:
import com.baidu.aip.nlp.AipNlp;
import org.json.JSONObject;
public class Sample {
// 设置APPID/AK/SK
public static final String APP_ID = "your_app_id";
public static final String API_KEY = "your_api_key";
public static final String SECRET_KEY = "your_secret_key";
public static void main(String[] args) {
// 初始化一个AipNlp
AipNlp client = new AipNlp(APP_ID, API_KEY, SECRET_KEY);
// 调用API进行文本分析
String text = "今天是个好日子";
JSONObject res = client.sentimentClassify(text, new HashMap<String, Object>());
System.out.println(res.toString(2));
}
}
- Summary
Through the above sample code, we can see that Java engineers only need to import the corresponding dependent libraries and use the ones provided by Baidu Java interface can easily connect to Baidu AI interface. In this way, we can quickly build intelligent applications and improve user experience. Of course, the above example is just a simple demonstration of how to connect to Baidu AI interface. In actual application, you also need to pay attention to the format of input data, processing of returned results and other issues. I hope this article can be helpful to Java engineers when using Baidu AI interface.
The above is the detailed content of How Java engineers can efficiently connect to Baidu AI interface. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn