As mentioned in the given question, we need to remove spaces from string using string stream. As the name suggests, string streams convert strings into streams. It works like cin in C. It is associated with a string object that has access to the string buffer in which it is stored.
string s =" a for apple, b for ball"; res = solve(s);
Using a string buffer, we will read each word one by one and concatenate it into a new string, which will be our answer.
Note - String-like streams are available in C's sstream header, so we need to include it.
Let’s look at some input/output scenarios
Assuming there are no spaces in the input of the function, the output result will be the same as the input -
Input: “Tutorialspoint” Result: “Tutorialspoint”
Assuming there are no spaces in the input of the function, the output result will be a string without spaces -
Input: “Tutorials Point” Result: “TutorialsPoint”
Assuming that the input accepted by the function only contains spaces, this method cannot provide output results -
Input: “ ” Result:
algorithm
Consider an input string with characters.
Checks if the string is empty and removes any whitespace present in the input using the stringstream keyword.
This process will be completed until the string stream pointer reaches the end of the line.
If the end of the line of the string is reached, the program terminates.
The updated string is returned to the output result.
Example
For example, we have a string such as "a represents apple, b represents ball", and we need to convert it to "aforapple,bforball".
Follow the detailed code to remove spaces from a string input to make it a stream of characters -
#include <iostream> #include <sstream> using namespace std; string solve(string s) { string answer = "", temp; stringstream ss; ss << s; while(!ss.eof()) { ss >> temp; answer+=temp; } return answer; } int main() { string s ="a for apple, b for ball"; cout << solve(s); return 0; }
Output
Aforapple,bforball
Example (using getline)
We have another way to solve the same query in C using getline.
#include <iostream> #include <sstream> using namespace std; string solve(string s) { stringstream ss(s); string temp; s = ""; while (getline(ss, temp, ' ')) { s = s + temp; } return s; } int main() { string s ="a for apple, b for ball"; cout << solve(s); return 0; }
Output
Aforapple,bforball
in conclusion
We see that using string streams, the strings are stored in a buffer and we can get the strings verbatim and concatenate them, removing spaces.
The above is the detailed content of C++ program to remove spaces from string using string stream. For more information, please follow other related articles on the PHP Chinese website!
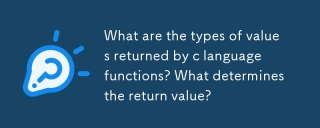
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
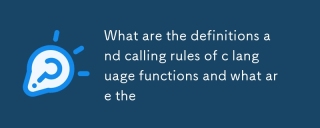
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
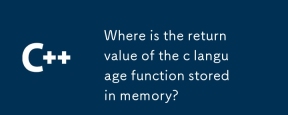
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
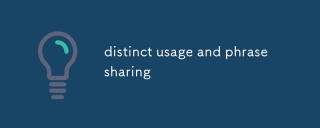
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
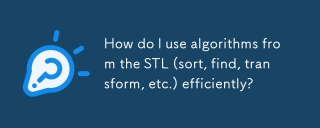
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
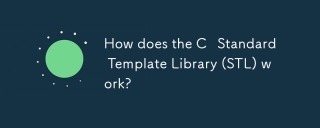
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
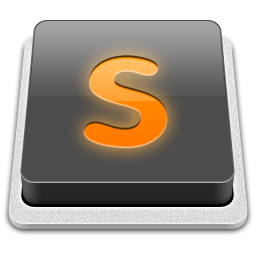
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
