How to do object-oriented programming in C?
Object-Oriented Programming (OOP) is a very common and important software development paradigm. C is a multi-paradigm programming language that includes support for object-oriented programming. In C, through the concepts of class and object, we can easily implement object-oriented programming.
First, we need to define a class. A class is a custom data type that encapsulates data members and member functions. Data members describe the properties of a class, and member functions define the behavior of the class.
The following example shows the definition of a simple class:
#include <iostream> class Shape { private: int width; int height; public: void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } int getArea() { return width * height; } }; int main() { Shape rectangle; rectangle.setWidth(10); rectangle.setHeight(5); int area = rectangle.getArea(); std::cout << "矩形的面积是:" << area << std::endl; return 0; }
In the above example, we defined a class named Shape. The Shape class has two data members: width and height, which represent the width and height of the rectangle respectively. The three member functions in the class are setWidth, setHeight and getArea. The setWidth and setHeight functions are used to set the width and height of the rectangle, while the getArea function returns the area of the rectangle.
In the main function, we create a Shape object rectangle and set its width and height by calling the setWidth and setHeight functions. Finally, we call the getArea function to calculate the area of the rectangle and output it to the console.
Through the concept of classes, we can create multiple objects to represent different instances. Each object has its own data members but shares the same member functions. This allows us to call the same member function on different objects to achieve code reuse.
In addition to encapsulating data members and member functions, classes also support inheritance and polymorphism. Inheritance allows one class to inherit members of another class, which enables code reuse and extension. Polymorphism allows using pointers or references of the parent class to point to objects of the subclass, achieving flexible programming.
The following example shows the use of inheritance and polymorphism:
#include <iostream> class Shape { protected: int width; int height; public: void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } virtual int getArea() = 0; }; class Rectangle : public Shape { public: int getArea() { return width * height; } }; class Triangle : public Shape { public: int getArea() { return width * height / 2; } }; int main() { Shape* shape1 = new Rectangle(); shape1->setWidth(10); shape1->setHeight(5); std::cout << "矩形的面积是:" << shape1->getArea() << std::endl; Shape* shape2 = new Triangle(); shape2->setWidth(10); shape2->setHeight(5); std::cout << "三角形的面积是:" << shape2->getArea() << std::endl; delete shape1; delete shape2; return 0; }
In the above example, we defined three classes: Shape, Rectangle and Triangle. Both the Rectangle and Triangle classes inherit from the Shape class, which means they inherit the setWidth, setHeight, and getArea functions from the Shape class. The Rectangle class overrides the getArea function to calculate the area of a rectangle; the Triangle class also overrides the getArea function to calculate the area of a triangle.
In the main function, we create two pointers of the Shape class, shape1 and shape2, and point to objects of the Rectangle and Triangle classes respectively. Through the shape1 and shape2 pointers, we can call the setWidth, setHeight and getArea functions. Since the getArea function is declared as a pure virtual function (virtual) in the Shape class, the shape1 and shape2 pointers will call different getArea functions according to the actual object type, achieving polymorphism.
Through the above examples, we have learned how to perform object-oriented programming in C, and mastered the basic concepts of class definition and use, inheritance and polymorphism. Object-oriented programming is a very powerful and flexible programming method. It can help us better organize and manage code, improve development efficiency and code reusability. I hope this article can help you understand and apply object-oriented programming!
The above is the detailed content of How to do object-oriented programming in C++?. For more information, please follow other related articles on the PHP Chinese website!
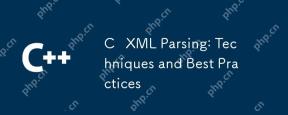
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
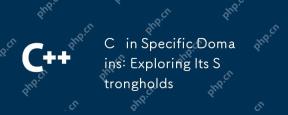
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
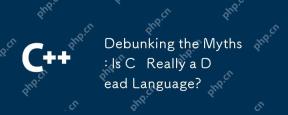
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
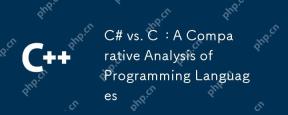
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
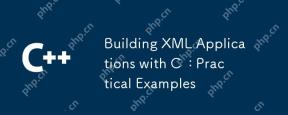
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
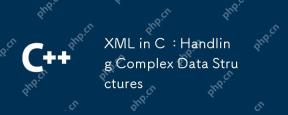
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
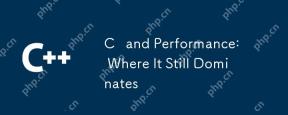
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
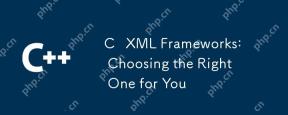
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
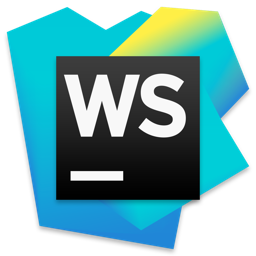
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
