How to use C++ for high-performance image matching and target tracking?
How to use C for high-performance image matching and target tracking?
Overview:
Image matching and target tracking are important research directions in the field of computer vision. They are widely used, including object recognition, detection, tracking, etc. In this article, we will introduce how to implement high-performance image matching and object tracking algorithms using the C programming language and explain in detail with code examples.
1. Image matching:
Image matching refers to finding similar feature points or corresponding feature areas between different images, thereby achieving registration or alignment between images. Commonly used image matching algorithms in C include SIFT, SURF and ORB. The following takes the ORB algorithm as an example to introduce the implementation process of image matching.
Code example:
#include <iostream> #include <opencv2/opencv.hpp> int main() { cv::Mat img1 = cv::imread("img1.jpg", cv::IMREAD_GRAYSCALE); cv::Mat img2 = cv::imread("img2.jpg", cv::IMREAD_GRAYSCALE); cv::Ptr<cv::ORB> orb = cv::ORB::create(); std::vector<cv::KeyPoint> keypoints1, keypoints2; cv::Mat descriptors1, descriptors2; orb->detectAndCompute(img1, cv::noArray(), keypoints1, descriptors1); orb->detectAndCompute(img2, cv::noArray(), keypoints2, descriptors2); cv::BFMatcher matcher(cv::NORM_HAMMING); std::vector<cv::DMatch> matches; matcher.match(descriptors1, descriptors2, matches); cv::Mat img_matches; cv::drawMatches(img1, keypoints1, img2, keypoints2, matches, img_matches); cv::imshow("Matches", img_matches); cv::waitKey(0); return 0; }
2. Target tracking:
Target tracking refers to tracking a specific target from a video sequence and achieving accurate positioning of its position in consecutive frames. Commonly used target tracking algorithms in C include MeanShift and CamShift.
Code example:
#include <iostream> #include <opencv2/opencv.hpp> int main() { cv::VideoCapture cap("video.mp4"); if (!cap.isOpened()) { std::cout << "Failed to open video file" << std::endl; return -1; } cv::Mat frame; cap >> frame; cv::Rect roi = cv::selectROI(frame); cv::Mat roi_img = frame(roi); cv::Mat hsv_roi; cv::cvtColor(roi_img, hsv_roi, cv::COLOR_BGR2HSV); cv::Mat roi_hist; int histSize[] = {16, 16}; float h_ranges[] = {0, 180}; const float* ranges[] = {h_ranges}; int channels[] = {0, 1}; cv::calcHist(&hsv_roi, 1, channels, cv::noArray(), roi_hist, 2, histSize, ranges, true, false); cv::normalize(roi_hist, roi_hist, 0, 255, cv::NORM_MINMAX); cv::TermCriteria term_crit(cv::TermCriteria::EPS | cv::TermCriteria::COUNT, 10, 1); cv::Mat frame_hsv; cv::Mat backproj; while (true) { cap >> frame; if (frame.empty()) break; cv::cvtColor(frame, frame_hsv, cv::COLOR_BGR2HSV); cv::calcBackProject(&frame_hsv, 1, channels, roi_hist, backproj, ranges); cv::RotatedRect track_box = cv::CamShift(backproj, roi, term_crit); cv::Point2f points[4]; track_box.points(points); for (int i = 0; i < 4; ++i) cv::line(frame, points[i], points[(i+1)%4], cv::Scalar(0, 255, 0), 2); cv::imshow("Tracking", frame); cv::waitKey(30); } return 0; }
Conclusion:
This article introduces how to use C for high-performance image matching and target tracking. Through code examples, the implementation process of the ORB algorithm in image matching and the CamShift algorithm in target tracking is explained in detail. I hope the content of this article will be helpful to readers in their study and practice of image processing and computer vision.
The above is the detailed content of How to use C++ for high-performance image matching and target tracking?. For more information, please follow other related articles on the PHP Chinese website!
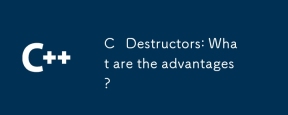
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
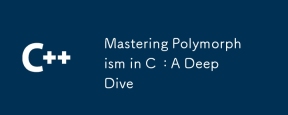
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
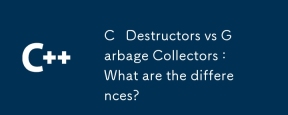
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
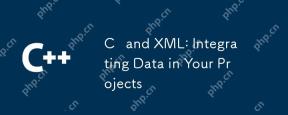
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
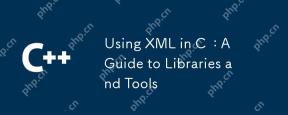
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
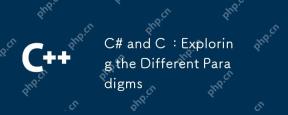
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
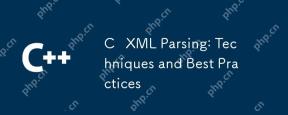
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
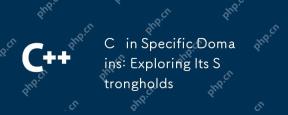
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
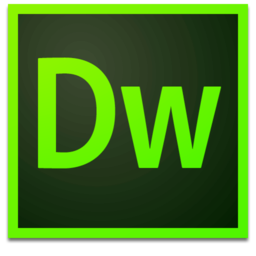
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
