Steps to build PHP commodity inventory management system
Steps to build PHP commodity inventory management system
Introduction:
With the rapid development of e-commerce, commodity inventory management has become an important question. An efficient and flexible commodity inventory management system can help companies understand inventory status in real time and improve operational efficiency. This article will introduce how to use PHP language to build a simple product inventory management system, and use sample code to help readers understand the implementation process.
Preparation work:
- Install the PHP environment: First make sure that your server environment has PHP installed. You can run php -v from the command line to verify the PHP version.
- Installing the database: This article will use the MySQL database. You need to ensure that the server environment has the MySQL service installed and running.
- Create database table: Use the following SQL statement to create a table named "goods" to store product information.
CREATE TABLE `goods` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(255) DEFAULT NULL, `price` decimal(10,2) DEFAULT NULL, `stock` int(11) DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8;
Step 1: Connect to the database
Connecting to the database in PHP code is a necessary step. Use the following code snippet to establish a connection to the MySQL database.
$servername = "localhost"; $username = "root"; $password = "password"; $dbname = "database_name"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); }
Modify the values of $servername, $username, $password and $dbname to your database address, username, password and database name respectively.
Step 2: Display the product list
Use the following code to query the product information in the database and display it on the page.
$sql = "SELECT * FROM goods"; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "ID:" . $row["id"]. " - 商品名:" . $row["name"]. " - 价格:" . $row["price"]. " - 库存:" . $row["stock"]. "<br>"; } } else { echo "暂无商品"; }
Step 3: Add products
Use the following code to implement the function of adding products.
if(isset($_POST["submit"])) { $name = $_POST["name"]; $price = $_POST["price"]; $stock = $_POST["stock"]; $sql = "INSERT INTO goods (name, price, stock) VALUES ('$name', $price, $stock)"; if ($conn->query($sql) === TRUE) { echo "商品添加成功"; } else { echo "商品添加失败:" . $conn->error; } }
Add the following code to the HTML form to enter product information.
<form method="post" action=""> <label for="name">商品名:</label> <input type="text" name="name" id="name"> <br> <label for="price">价格:</label> <input type="text" name="price" id="price"> <br> <label for="stock">库存:</label> <input type="number" name="stock" id="stock"> <br> <input type="submit" name="submit" value="添加商品"> </form>
Step 4: Update inventory
Use the following code to update the inventory function.
if(isset($_POST["update"])) { $id = $_POST["id"]; $stock = $_POST["stock"]; $sql = "UPDATE goods SET stock = $stock WHERE id = $id"; if ($conn->query($sql) === TRUE) { echo "库存更新成功"; } else { echo "库存更新失败:" . $conn->error; } }
Add the following code to the HTML form to enter the product ID and new inventory.
<form method="post" action=""> <label for="id">商品ID:</label> <input type="number" name="id" id="id"> <br> <label for="stock">新库存:</label> <input type="number" name="stock" id="stock"> <br> <input type="submit" name="update" value="更新库存"> </form>
Summary:
Through the above steps, we have successfully built a simple PHP product inventory management system. This system can display product lists, add products, and update product inventory. Of course, this is only the initial stage of the system. You can expand and optimize it according to actual needs, such as adding new functions such as deleting products and searching for products. I hope this article will be helpful to you and enable you to better understand and apply the PHP language.
The above is the detailed content of Steps to build PHP commodity inventory management system. For more information, please follow other related articles on the PHP Chinese website!
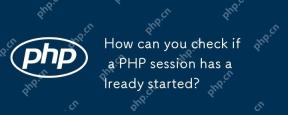
In PHP, you can use session_status() or session_id() to check whether the session has started. 1) Use the session_status() function. If PHP_SESSION_ACTIVE is returned, the session has been started. 2) Use the session_id() function, if a non-empty string is returned, the session has been started. Both methods can effectively check the session state, and choosing which method to use depends on the PHP version and personal preferences.
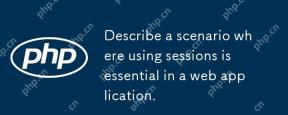
Sessionsarevitalinwebapplications,especiallyfore-commerceplatforms.Theymaintainuserdataacrossrequests,crucialforshoppingcarts,authentication,andpersonalization.InFlask,sessionscanbeimplementedusingsimplecodetomanageuserloginsanddatapersistence.
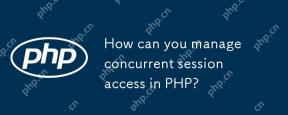
Managing concurrent session access in PHP can be done by the following methods: 1. Use the database to store session data, 2. Use Redis or Memcached, 3. Implement a session locking strategy. These methods help ensure data consistency and improve concurrency performance.
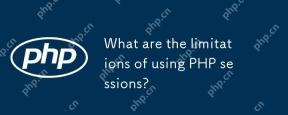
PHPsessionshaveseverallimitations:1)Storageconstraintscanleadtoperformanceissues;2)Securityvulnerabilitieslikesessionfixationattacksexist;3)Scalabilityischallengingduetoserver-specificstorage;4)Sessionexpirationmanagementcanbeproblematic;5)Datapersis
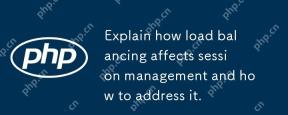
Load balancing affects session management, but can be resolved with session replication, session stickiness, and centralized session storage. 1. Session Replication Copy session data between servers. 2. Session stickiness directs user requests to the same server. 3. Centralized session storage uses independent servers such as Redis to store session data to ensure data sharing.
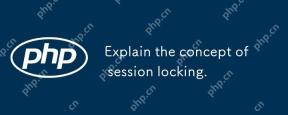
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
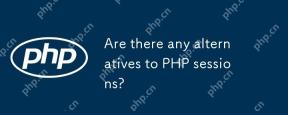
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
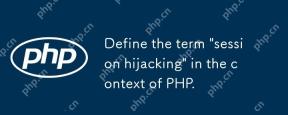
Sessionhijacking refers to an attacker impersonating a user by obtaining the user's sessionID. Prevention methods include: 1) encrypting communication using HTTPS; 2) verifying the source of the sessionID; 3) using a secure sessionID generation algorithm; 4) regularly updating the sessionID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
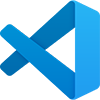
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
