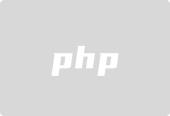
Best practices and technologies for developing embedded systems using C
Abstract:
With the widespread application of embedded systems in various fields, the development of embedded systems using C Efficient and reliable embedded systems have become an important task. This article will introduce the best practices and technologies for developing embedded systems using C, including system architecture, code optimization and debugging techniques, and demonstrate specific implementation methods through code examples.
- Introduction
With the continuous development of hardware technology, embedded systems have been widely used in various fields such as automobiles, home appliances, and medical equipment. For embedded system developers, how to use C language to develop efficient and reliable embedded systems has become an important task. This article will help readers better cope with the challenges of embedded system development by introducing best practices and technologies in actual development.
- System Architecture Design
The architecture design of embedded systems is a key part of the entire system development process. During the design process, the following principles should be followed:
- Modularization: Split the system into multiple modules, each module focuses on completing a specific function and communicates through interfaces.
- Reusability: Use existing modules and libraries as much as possible to reduce repeated development work.
- Scalability: The design should take into account possible future changes in demand for the system and minimize modification costs.
- Low coupling: Modules should be coupled as loosely as possible to reduce dependencies between modules.
The following is a simple embedded system architecture design example:
class Sensor {
public:
virtual void readData() = 0;
};
class Actuator {
public:
virtual void controlDevice() = 0;
};
class TemperatureSensor : public Sensor {
public:
void readData() override {
// 读取温度传感器数据
}
};
class DisplayActuator : public Actuator {
public:
void controlDevice() override {
// 控制显示设备
}
};
class System {
private:
Sensor* sensor;
Actuator* actuator;
public:
System(Sensor* sensor, Actuator* actuator) : sensor(sensor), actuator(actuator) {}
void update() {
sensor->readData();
actuator->controlDevice();
}
};
int main() {
TemperatureSensor* tempSensor = new TemperatureSensor();
DisplayActuator* display = new DisplayActuator();
System* system = new System(tempSensor, display);
while (true) {
system->update();
}
}
In the above example, we split the system into sensor and actuator modules, and passed abstract base classes defines their behavior. Using this modular design, we can easily expand and maintain the system.
- Code Optimization
In order to obtain better performance and resource utilization in embedded systems, code optimization is an essential task. The following are several common code optimization tips:
- Reduce memory allocation: Embedded systems have limited memory resources, so dynamic allocation of memory should be minimized. You can use static allocation or object pooling to avoid dynamic memory allocation.
- Use bit operations: Bit operations can improve the execution efficiency of your code, especially when processing large amounts of data. For example, use bitwise AND (&) and bitwise OR (|) instead of the AND and OR operators.
- Reduce function calls: Function calls will generate additional overhead. Function calls can be reduced by inlining functions or manually unrolling loops.
- Optimizing loops: In embedded systems, loops are one of the performance bottlenecks. Loops can be optimized through the judicious use of loop unrolling, loop reordering, and reducing calculations inside the loop.
The following is a simple code optimization example:
uint8_t computeChecksum(uint8_t* data, size_t length) {
uint8_t checksum = 0;
for (size_t i = 0; i < length; i++) {
checksum += data[i];
}
return checksum;
}
uint8_t computeChecksumOptimized(uint8_t* data, size_t length) {
uint8_t checksum = 0;
size_t i = 0;
for (; i + 8 < length; i += 8) {
checksum += data[i] + data[i + 1] + data[i + 2] + data[i + 3]
+ data[i + 4] + data[i + 5] + data[i + 6] + data[i + 7];
}
for (; i < length; i++) {
checksum += data[i];
}
return checksum;
}
In the above example, we combine the 8 addition operations in each loop into one by unrolling the loop, thus The amount of calculation is reduced and the execution efficiency of the code is improved.
- Debugging skills
In embedded system development, debugging is an essential task. The following are several common debugging techniques:
- Use a debugger: You can use the debugger to step through the code and observe the values of variables and the execution flow of the program in order to locate the problem.
- Add logs: Adding log output at key locations can help us track the execution process of the program and discover hidden errors.
- Simulation environment: During the debugging process, you can use the simulation environment to reproduce the problem in order to better understand and fix the problem.
- Unit testing: Writing unit tests can verify whether the functions of each module are normal and help eliminate integration problems between modules.
- Conclusion
Using C to develop embedded systems requires following some best practices and technologies, including system architecture design, code optimization and debugging skills. This article introduces these aspects and shows how to implement them through code examples. We hope that these contents will be helpful to embedded system developers so that they can develop efficient and reliable embedded systems.
The above is the detailed content of Best practices and techniques for developing embedded systems using C++. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn