


Learn and apply PHP writing standards: Methodology to improve code quality
Introduction
Writing high-quality PHP code is crucial for developers . Following PHP writing standards can help us write code that is highly readable, easy to maintain and easy to collaborate with. This article will introduce some common PHP writing specifications and use code examples to illustrate how to apply these specifications to improve code quality.
- Code indentation
Code indentation is an important part of code writing. It can make the structure of the code clearer and easier to read. In PHP writing specifications, 4 spaces are used as the standard for code indentation. For example:
function myFunction() { if ($condition) { // do something } else { // do something else } }
- Naming convention
Good naming convention can make the code more readable and maintainable. In PHP writing specifications, use camel case naming to name functions, variables, classes, and methods. Also be careful to avoid using names that are too simple or non-descriptive. For example:
$myVariable = 123; function myFunction($myParameter) { // do something } class MyClass { public function myMethod() { // do something } }
- Comment specifications
Good comments can help other developers better understand the intent and function of the code. In PHP writing specifications, comments should be placed above the code, and use // or / / to comment the code. For functions and methods, comments should be used above them to describe their functions and parameter descriptions. For example:
/** * This function calculates the sum of two numbers. * * @param int $num1 The first number. * @param int $num2 The second number. * @return int The sum of the two numbers. */ function calculateSum($num1, $num2) { return $num1 + $num2; } // This is a comment for the code below $sum = calculateSum(1, 2); echo $sum;
- Error handling and exception handling
Good error handling and exception handling are necessary steps to ensure code quality. In PHP writing standards, exceptions should be used to handle errors and exceptions. For example:
try { // some code that may throw an exception } catch (Exception $e) { // handle the exception }
- Function and Method Specifications
Writing well-written functions and methods is crucial to the readability and maintainability of your code. In PHP writing specifications, functions and methods should have clear functions and try to follow the "single responsibility principle". Also pay attention to the naming and type declaration of parameters. For example:
/** * This function calculates the sum of two numbers. * * @param int $num1 The first number. * @param int $num2 The second number. * @return int The sum of the two numbers. */ function calculateSum($num1, $num2) { return $num1 + $num2; } class MyClass { /** * This method prints a greeting message. * * @param string $name The name of the person to greet. * @return void */ public function greet($name) { echo "Hello, " . $name; } }
Conclusion
By learning and applying PHP writing standards, we can write high-quality, easy-to-read, and easy-to-maintain code. This article introduces some common PHP writing conventions and shows how to apply these conventions to improve code quality through code examples. I hope this article will be helpful to PHP developers and lead everyone to write better code.
The above is the detailed content of Learn and apply PHP writing conventions: Methodology to improve code quality. For more information, please follow other related articles on the PHP Chinese website!
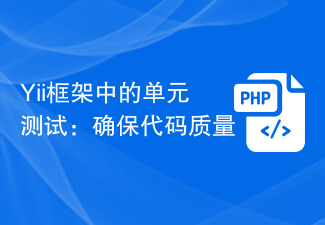
随着软件开发的日益复杂化,确保代码质量变得越来越重要。在Yii框架中,单元测试是一种非常强大的工具,可以确保代码的正确性和稳定性。在本文中,我们将深入探讨Yii框架中的单元测试,并介绍如何使用Yii框架进行单元测试。什么是单元测试?单元测试是一种软件测试方法,通常用于测试一个模块、函数或方法的正确性。单元测试通常由开发人员编写,旨在确保代码的正确性和稳定性。
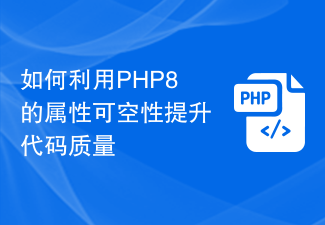
随着PHP8的发布,属性可空性成为了一个重要的新特性。这个特性使得我们可以声明一个属性可以为空,使得我们可以更好地控制我们的代码,并且可以帮助我们减少一些潜在的错误。属性可空性是什么?在PHP之前,我们只能声明属性为固定类型(例如字符串、整数、布尔等)。然而,在某些情况下,属性可能不会被初始化或者赋值为空。这意味着在调用这些属性时,我们可能会遇到一个致命的错
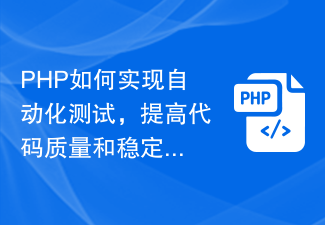
在现代软件开发过程中,自动化测试已成为了保证软件质量和稳定性的必要手段之一。其中,针对PHP开发的自动化测试技术更是越来越成熟和广泛应用。本文将从自动化测试的基本概念入手,讲解PHP自动化测试的实现方式和应用场景,以及如何通过自动化测试来提高代码质量和稳定性。一、自动化测试简介自动化测试是指将软件测试过程中繁琐、耗时的任务通过程序自动化实现,包括测试用例的
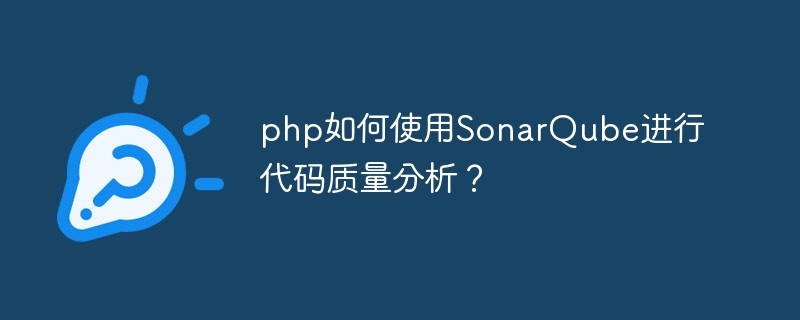
随着现代软件开发的日益复杂,代码的质量对于项目的成功至关重要。为了确保代码质量,SonarQube成为了一个广泛使用的开源代码质量平台。本文将介绍如何使用SonarQube进行针对PHP的代码质量分析。SonarQube是一个开源的代码质量管理平台,它可以帮助开发团队监测代码质量,并按时间轴提供有关代码质量,缺陷和安全性的详细信息。SonarQube的工作方
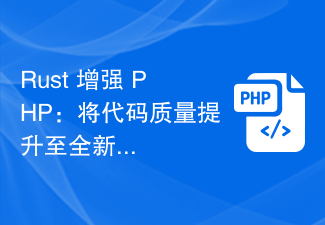
Rust增强PHP:将代码质量提升至全新水平,需要具体代码示例引言:PHP是一种广泛应用于Web开发的脚本语言,由于其灵活性和易学性,成为了很多开发者的首选。然而,PHP在一些方面存在一些缺陷,比如类型不安全、内存管理不当等问题。这就容易导致一些常见的错误和安全隐患。为了解决这些问题,一种名为Rust的编程语言成为了PHP开发者的热门选择。本
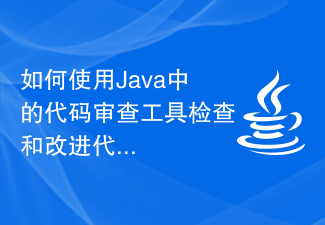
如何使用Java中的代码审查工具检查和改进代码的质量?代码质量是保证软件正常运行和可维护性的重要因素之一。为了确保代码的质量,我们可以使用代码审查工具来检查和改进代码的质量。这篇文章将介绍如何使用Java中的代码审查工具来提高代码的质量。使用Java代码审查工具可以帮助我们自动检测代码中的潜在问题,包括潜在的错误、潜在的性能问题和潜在的风险等。同时,它还可以
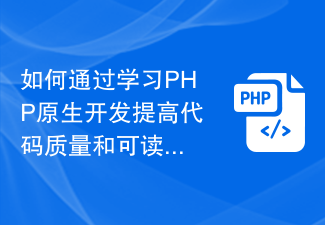
如何通过学习PHP原生开发提高代码质量和可读性引言:PHP是一种广泛应用于网站开发的脚本语言,其灵活性和易学性成为众多开发者的首选。然而,随着项目的复杂性增加,开发高质量、可维护性和可读性的代码变得至关重要。本文将介绍如何通过学习PHP原生开发来提高代码质量和可读性,并通过代码示例进行详细说明。一、遵循PHP编码规范代码缩进和格式化良好的代码缩进和格式化可以
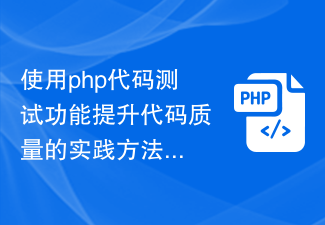
使用PHP代码测试功能提升代码质量的实践方法代码质量对于软件开发来说非常重要,它直接影响着软件的稳定性和可扩展性。而测试是保证代码质量的重要手段之一。本文将介绍使用PHP代码测试来提升代码质量的实践方法,并给出具体的代码示例。一、测试的重要性在软件开发过程中,测试可以帮助我们发现代码中的潜在错误和漏洞,避免这些问题在生产环境中产生严重后果。同时,测试还可以提


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
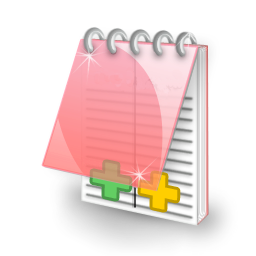
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version