How to use C++ to program various functions of advanced embedded systems
How to use C to write various functions of advanced embedded systems
In today's rapidly developing technological era, embedded systems are increasingly used in various fields. , its demand for advanced features is also increasing. As a cross-platform programming language, C is widely used in the development of embedded systems. This article will introduce how to use C to write various functions of advanced embedded systems and give corresponding code examples.
- Multi-threaded programming
Multi-threaded programming is very useful in embedded systems, it can improve the concurrency and responsiveness of the system. C 11 introduces multi-threading support of the standard library. We can use std::thread to create and manage threads. The sample code is as follows:
#include <iostream> #include <thread> void printHello() { std::cout << "Hello from thread!" << std::endl; } int main() { std::thread t(printHello); t.join(); // 等待子线程结束 return 0; }
- File operation
Embedding Traditional systems usually require file operations, such as reading and writing configuration files, saving data, etc. C provides the fstream library for file operations. The sample code is as follows:
#include <iostream> #include <fstream> int main() { std::ofstream ofs("data.txt"); // 创建写文件流 if (ofs.is_open()) { ofs << "Hello, world!" << std::endl; ofs.close(); } std::ifstream ifs("data.txt"); // 创建读文件流 if (ifs.is_open()) { std::string line; while (std::getline(ifs, line)) { std::cout << line << std::endl; } ifs.close(); } return 0; }
- Network communication
With the development of the Internet of Things, embedded systems increasingly require network communication. C provides a sockets library for network programming. The sample code is as follows:
#include <iostream> #include <sys/socket.h> #include <arpa/inet.h> int main() { int sockfd = socket(AF_INET, SOCK_STREAM, 0); // 创建socket if (sockfd == -1) { std::cerr << "Failed to create socket." << std::endl; return -1; } struct sockaddr_in addr; addr.sin_family = AF_INET; addr.sin_port = htons(8080); addr.sin_addr.s_addr = inet_addr("127.0.0.1"); if (connect(sockfd, (struct sockaddr*)&addr, sizeof(addr)) < 0) { // 连接服务器 std::cerr << "Failed to connect to server." << std::endl; return -1; } char buffer[1024] = {0}; if (recv(sockfd, buffer, sizeof(buffer), 0) < 0) { // 接收数据 std::cerr << "Failed to receive data." << std::endl; return -1; } std::cout << "Received: " << buffer << std::endl; close(sockfd); // 关闭socket return 0; }
- Database operation
Embedded systems usually need to interact with the database, such as storage and reading data. C provides an ODBC interface to connect to the database and operate using SQL statements. The sample code is as follows:
#include <iostream> #include <sql.h> #include <sqlext.h> int main() { SQLHENV env; SQLHDBC dbc; SQLHSTMT stmt; SQLRETURN ret; SQLAllocHandle(SQL_HANDLE_ENV, SQL_NULL_HANDLE, &env); SQLSetEnvAttr(env, SQL_ATTR_ODBC_VERSION, (SQLPOINTER)SQL_OV_ODBC3, 0); SQLAllocHandle(SQL_HANDLE_DBC, env, &dbc); SQLDriverConnect(dbc, NULL, (SQLCHAR*)"DSN=mydsn;", SQL_NTS, NULL, 0, NULL, SQL_DRIVER_COMPLETE); SQLAllocHandle(SQL_HANDLE_STMT, dbc, &stmt); SQLExecDirect(stmt, (SQLCHAR*)"SELECT * FROM mytable", SQL_NTS); SQLCHAR col1[256]; SQLLEN len1; while (SQLFetch(stmt) == SQL_SUCCESS) { SQLGetData(stmt, 1, SQL_C_CHAR, col1, sizeof(col1), &len1); std::cout << col1 << std::endl; } SQLFreeHandle(SQL_HANDLE_STMT, stmt); SQLDisconnect(dbc); SQLFreeHandle(SQL_HANDLE_DBC, dbc); SQLFreeHandle(SQL_HANDLE_ENV, env); return 0; }
The above is a sample code for using C to write various functions of advanced embedded systems. By properly using functions such as multi-threading, file operations, network communications, and database operations, we can implement more advanced and complex embedded systems. Of course, in actual development, you also need to consider issues such as resource limitations and real-time performance of embedded systems. However, this article only provides you with some ideas and sample codes for writing advanced functions. I hope it will be helpful to the development of embedded systems.
The above is the detailed content of How to use C++ to program various functions of advanced embedded systems. For more information, please follow other related articles on the PHP Chinese website!
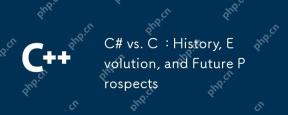
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
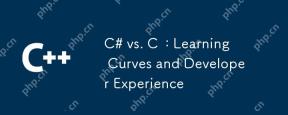
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
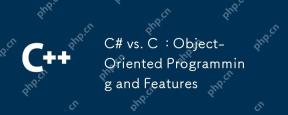
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
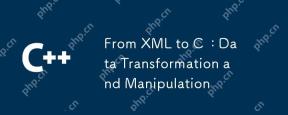
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
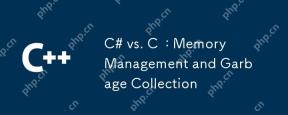
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
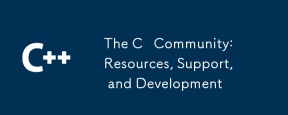
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
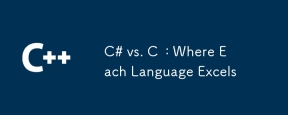
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
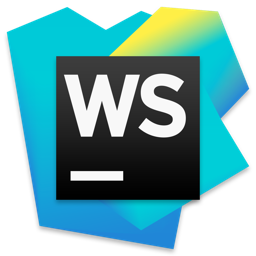
WebStorm Mac version
Useful JavaScript development tools
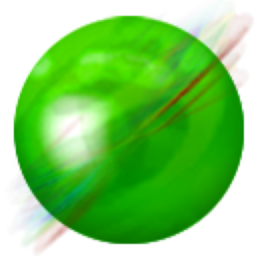
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.