How to solve: Java file operation error: Unable to delete file
In Java programming, processing files is a common and important task. However, sometimes we may face the problem of not being able to delete files. This article will introduce some methods to solve this problem and provide relevant Java code examples.
- Check whether the file is occupied by other processes
First, we need to determine whether the file is occupied by other processes. If the file is being read or written by another program, Java will not be able to delete it. We can handle files better by using try-with-resources block.
try (FileInputStream inputStream = new FileInputStream("file.txt"); FileOutputStream outputStream = new FileOutputStream("file2.txt")) { // 操作文件的代码 } catch (IOException e) { // 处理异常 }
In this example, we use the try-with-resources block to automatically close the input and output streams. This ensures that the file is no longer occupied by another process, allowing us to delete the file.
- Check the permissions of the file
If the permissions of the file are not set correctly, Java cannot delete it. We can use the File.setWritable()
method to set the writable attribute of the file.
File file = new File("file.txt"); if (file.setWritable(true)) { if (file.delete()) { System.out.println("文件删除成功!"); } else { System.out.println("文件删除失败!"); } } else { System.out.println("无法设置文件的写权限!"); }
In this example, we first use the File.setWritable(true)
method to set the writable attribute of the file. If the setting is successful, we will try to delete the file using the File.delete()
method. If the file is deleted successfully, the console will output "File deleted successfully!", otherwise it will output "File deleted failed!".
- Using File Locking
Another common problem is that in a multi-threaded environment, multiple threads try to delete the same file at the same time. To solve this problem, we can use a file locking mechanism to ensure that only one thread can access the file.
File file = new File("file.txt"); try (FileChannel channel = new RandomAccessFile(file, "rw").getChannel()) { FileLock lock = channel.lock(); if (file.delete()) { System.out.println("文件删除成功!"); } else { System.out.println("文件删除失败!"); } lock.release(); } catch (IOException e) { e.printStackTrace(); }
In this example, we use FileChannel
and FileLock
to implement file locking. First, we use RandomAccessFile
to create a read-write file channel, and then use the channel.lock()
method to lock the file. After deleting the file, we use lock.release()
to release the file lock.
Summary:
Unable to delete files is one of the common errors in Java file operations. To solve this problem, we can check whether the file is occupied by other processes, check the permissions of the file, and use the file locking mechanism. I hope the solutions and sample code provided in this article can help readers better handle Java file operation errors.
The above is the detailed content of How to fix: Java file operation error: Unable to delete file. For more information, please follow other related articles on the PHP Chinese website!
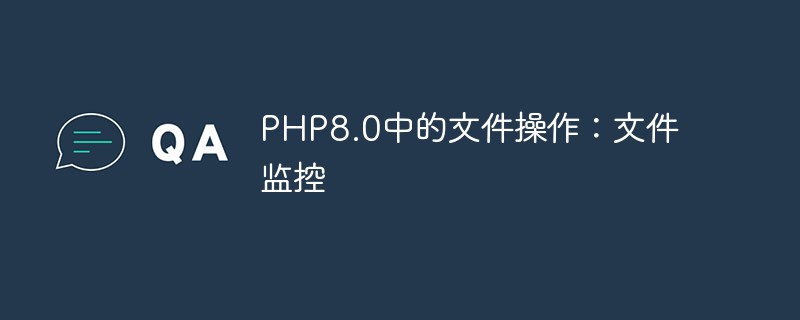
随着Web应用程序的不断发展,PHP已经成为了Web开发中最重要的编程语言之一。作为一门灵活性极强的编程语言,PHP的每个版本都带来了新的功能和优化,为了满足不同的需求应用场景。在PHP8.0版本中,新增了一个非常实用的文件操作功能,即文件监控。这个功能非常适用于那些需要对文件变化进行监控和处理的应用场景,比如文件备份、文件同步、日志监控等等。本文将带大家
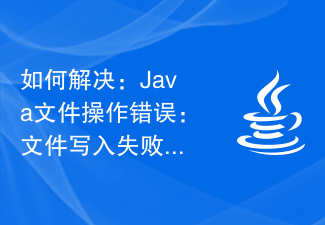
如何解决:Java文件操作错误:文件写入失败在Java编程中,经常会遇到文件操作的需求,而文件写入是其中一项重要的功能。然而,有时候我们会遇到文件写入失败的错误,这可能导致程序无法正常运行。本文将介绍一些常见原因和解决方法,帮助您解决这类问题。路径错误:一个常见的问题是文件路径错误。当我们尝试将文件写入到指定路径时,如果路径不存在或者权限不足,都会导致文件写
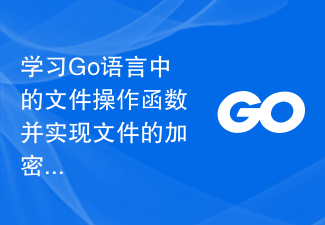
学习Go语言中的文件操作函数并实现文件的加密压缩上传下载功能Go语言是一种开源的静态类型编程语言,它以其高效性能和简洁的语法在开发领域广受欢迎。在Go语言的标准库中,提供了丰富的文件操作函数,使得对文件进行读写、加密压缩、上传下载等操作变得非常简单。本文将介绍如何使用Go语言中的文件操作函数,实现对文件进行加密压缩、上传下载的功能。首先,我们需要导入相关的三
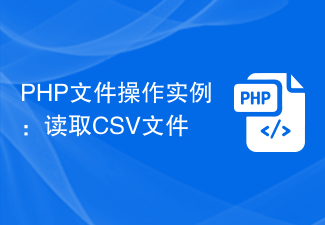
PHP是一种广泛应用于Web开发的流行编程语言。在Web应用程序中,文件操作是一个基本而常见的功能。本文将介绍如何使用PHP读取CSV文件并将其显示在HTML表格中。CSV是一种常见的文件格式,用于将表格数据导入到电子表格软件中(如Excel)。csv文件通常由许多行组成,每行由逗号分隔的值组成。第一行通常包含列头,它们描述各列值的含义。这里我们将使用PHP
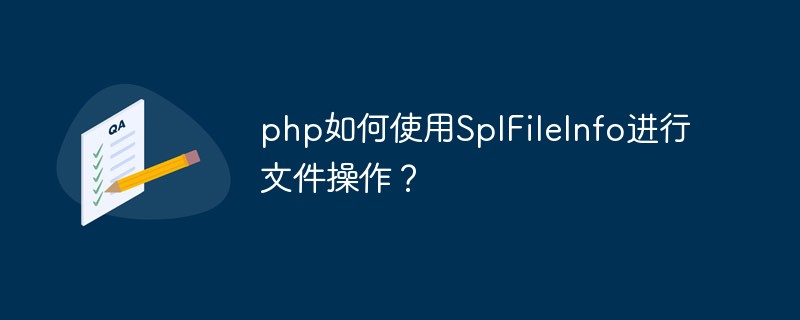
作为一种广泛使用的服务器端编程语言,PHP不仅提供了许多方便的文件处理函数,而且还提供了一些更为高级的文件操作类。其中一个比较有用的类就是SplFileInfo,它能够让我们更加灵活、高效地进行文件读写操作。本文将介绍如何使用PHP中的SplFileInfo类进行文件操作。一、SplFileInfo类的概述SplFileInfo类是PHP中的一个内置类(不需
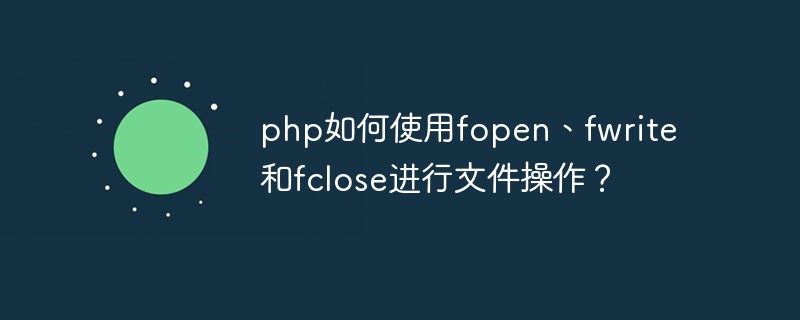
在PHP开发中,对文件的操作是非常常见的。一般情况下,我们需要进行文件的读取、写入、删除等操作。其中,文件的读取可以使用fopen函数和fread函数,文件的写入可以使用fopen函数、fwrite函数和fclose函数。本文将介绍php如何使用fopen、fwrite和fclose进行文件操作。一、fopen函数fopen函数用于打开文件,它的语法如下:r
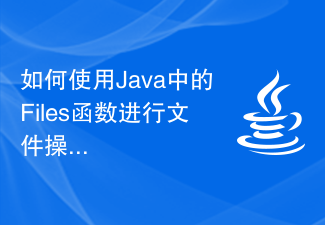
在Java编程语言中,经常需要进行文件的读取、写入、复制、删除等操作。Java提供了一组Files类的函数来进行文件操作。本文将介绍如何使用Java中的Files函数进行文件操作。导入所需的包在进行文件操作之前,首先要导入Java的io包和nio包:importjava.io.File;importjava.io.IOException;import
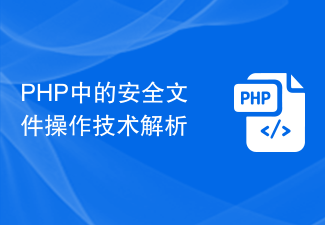
PHP是一种广泛应用于Web开发的脚本语言,众所周知,网络环境中存在着各种各样的安全风险。在PHP文件操作过程中,保证安全性显得尤为重要。本文将对PHP中的安全文件操作技术进行详细解析,以帮助开发人员加强对文件操作的安全防护。一、文件路径注入(PathTraversal)文件路径注入是指攻击者通过输入恶意参数,成功地绕过文件系统的访问控制,访问不在预期访问


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
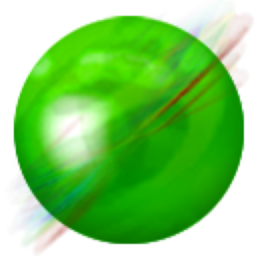
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
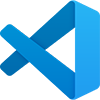
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.