Chatbot and automatic reply in PHP real-time chat system
Chatbot and automatic reply in PHP real-time chat system
Introduction:
With the popularity of social media and real-time communication, more and more websites and apps started offering live chat capabilities. In order to improve the user experience, many developers have begun to introduce chatbots and automatic reply functions into their chat systems. In this article, we will introduce how to use PHP language to implement chatbot and automatic reply functions.
Part One: Implementation of Chatbot
A chatbot is a program that can simulate human conversation. It can respond accordingly to questions entered by the user. In order to implement a chatbot, we can use Natural Language Processing (NLP) technology.
The following is a sample code for a simple chatbot:
<?php // 聊天机器人的回答列表 $bot_responses = [ '你好' => '你好!', '你叫什么名字' => '我叫小智。', '今天天气怎么样' => '今天天气晴朗,适合出门。', // 更多回答... ]; // 获取用户输入 $user_input = $_POST['message']; // 处理用户输入 function process_user_input($input) { // 去除多余的空格 $input = trim($input); // 将输入转换为小写 $input = strtolower($input); return $input; } // 检查用户输入是否在聊天机器人的回答列表中 if (array_key_exists($user_input, $bot_responses)) { $bot_answer = $bot_responses[$user_input]; } else { $bot_answer = '抱歉,我不明白你的意思。'; } // 返回聊天机器人的回答 echo $bot_answer; ?>
In the above code, we first define a list of answers for the chatbot. When the user enters a question, we process the question entered by the user and then check whether the user input is in the answer list. If the corresponding answer is found in the list, we will return that answer, otherwise return the default answer.
Part 2: Implementation of Auto-Reply
Auto-reply is a function that can automatically respond to the user's message. When implementing auto-reply functionality, we can use keyword matching to determine the user’s intent.
The following is a sample code for a simple auto-reply function:
<?php // 自动回复的关键词列表 $auto_responses = [ '你好' => '你好!如果你有什么问题,可以随时问我。', '谢谢' => '不用客气,我会随时为你效劳的。', '今天天气怎么样' => '今天天气晴朗,适合出门。', // 更多回答... ]; // 获取用户输入 $user_input = $_POST['message']; // 处理用户输入 function process_user_input($input) { // 去除多余的空格 $input = trim($input); // 将输入转换为小写 $input = strtolower($input); return $input; } // 匹配用户输入的关键词 foreach ($auto_responses as $keyword => $response) { if (stripos($user_input, $keyword) !== false) { $bot_answer = $response; break; } } // 返回自动回复的答案 echo $bot_answer; ?>
In the above code, we first define a list of keywords for auto-reply. After the user sends a message, we process the message entered by the user, and then traverse the keyword list to find keywords that match the message entered by the user. If a matching keyword is found, we will return the corresponding answer.
Conclusion:
Through the above code example, we can see how to use PHP to implement a simple chatbot and automatic reply function. Of course, this is just a basic implementation, and developers can expand and adjust it according to actual needs. Chatbots and automatic reply functions in real-time chat systems can not only improve user experience, but also reduce the work pressure of manual customer service and improve work efficiency.
The above is the detailed content of Chatbot and automatic reply in PHP real-time chat system. For more information, please follow other related articles on the PHP Chinese website!
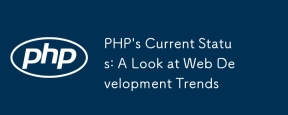
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
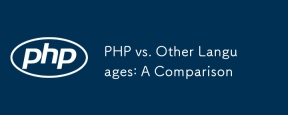
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
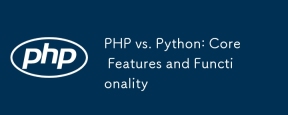
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
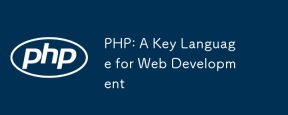
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
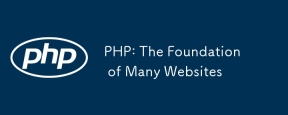
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
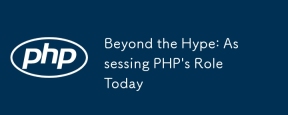
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
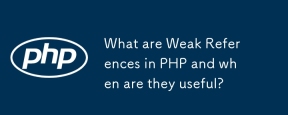
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
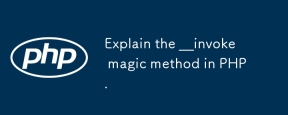
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
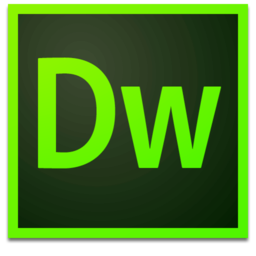
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.