How to use PHP to develop blog functions
With the rapid development of the Internet, blogs have become an important platform for people to share their opinions and experiences. As a developer, using PHP to develop blog functions allows us to customize and control the functions of the blog more freely. This article will introduce the steps to use PHP to develop blog functions, and attach code examples.
- Database design
First, we need to create a database to store blog-related data. The following is an example database design:
CREATE TABLE `blogs` ( `id` int(11) UNSIGNED AUTO_INCREMENT PRIMARY KEY, `title` varchar(255) NOT NULL, `content` text NOT NULL, `author` varchar(100) NOT NULL, `created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP, `updated_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
The above code creates a table named blogs, which contains fields such as id, title, content, author, created_at and updated_at. These fields are used to store the blog's ID, title, content, author, and creation/update time.
- Blog list page
Next, we need to create a blog list page to display all blog posts. The following is a simple sample code:
<?php // 连接数据库 $dbHost = 'localhost'; $dbUsername = 'root'; $dbPassword = 'password'; $dbName = 'blog_db'; $conn = new mysqli($dbHost, $dbUsername, $dbPassword, $dbName); // 查询博客列表 $result = $conn->query("SELECT * FROM blogs"); // 遍历查询结果并展示博客列表 if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "<h2 id="row-title">{$row['title']}</h2>"; echo "<p>{$row['content']}</p>"; echo "<p>作者:{$row['author']}</p>"; echo "<p>发布时间:{$row['created_at']}</p>"; } } else { echo "暂无博客文章"; } // 关闭数据库连接 $conn->close(); ?>
The above code first connects to the database, then queries the blogs table in the database, and displays the blog list by looping through the query results. If no blog posts are found, the message "No blog posts yet" will be displayed.
- Create a blog page
In addition to displaying existing blog posts, we also need to provide a page to create new blog posts. The following is an example code:
<?php // 连接数据库 $conn = new mysqli($dbHost, $dbUsername, $dbPassword, $dbName); // 获取表单提交的数据 if ($_SERVER["REQUEST_METHOD"] == "POST") { $title = $_POST['title']; $content = $_POST['content']; $author = $_POST['author']; // 插入数据到数据库 $conn->query("INSERT INTO blogs (title, content, author) VALUES ('$title', '$content', '$author')"); } ?> <form method="post"> <label for="title">标题:</label> <input type="text" name="title" id="title"> <br> <label for="content">内容:</label> <textarea name="content" id="content"></textarea> <br> <label for="author">作者:</label> <input type="text" name="author" id="author"> <br> <input type="submit" value="发布"> </form>
The above code first connects to the database, and then obtains the data submitted by the form through $_POST. Next, insert the data into the blogs table of the database. Finally, display a form containing title, content and author input boxes, and create a blog post through a submit button.
Summary:
Through the above steps, we can use PHP to develop blog functions. First, design and create a database to store blog data, then implement a blog list page to display existing blog posts, and finally create a blog page to create new blog posts. In actual development, we can customize and expand more functions according to needs.
The above is a brief introduction and sample code on how to use PHP to develop blog functions. I hope it will be helpful to you. If you have any questions, please leave a message to communicate with me. Happy development!
The above is the detailed content of How to use PHP to develop blog functions. For more information, please follow other related articles on the PHP Chinese website!
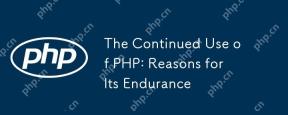
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
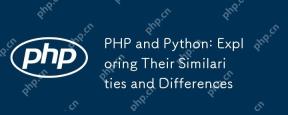
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
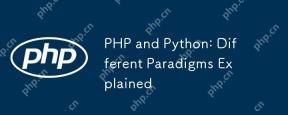
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
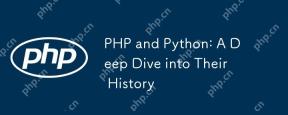
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
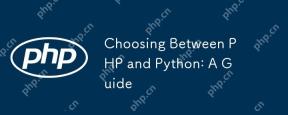
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
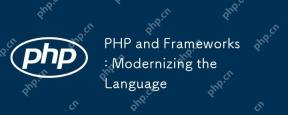
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
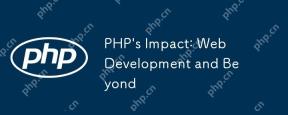
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
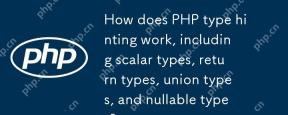
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
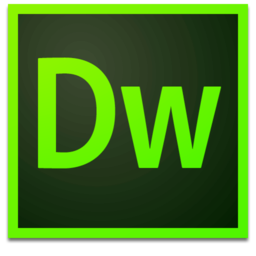
Dreamweaver Mac version
Visual web development tools
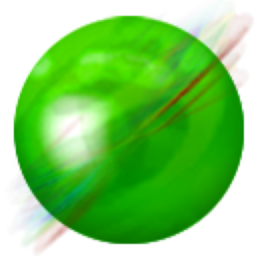
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
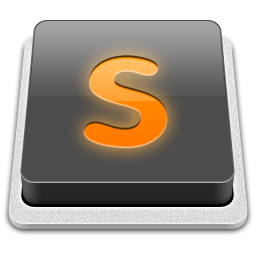
SublimeText3 Mac version
God-level code editing software (SublimeText3)