How to use C++ language to optimize various functions of embedded systems
How to use C language to optimize various functions of embedded systems
An embedded system is a computer system designed and manufactured specifically for specific tasks. It usually has the characteristics of high real-time requirements, low power consumption, and limited resources. In the development process of embedded systems, how to optimize various functions has become a key task. This article will introduce how to use C language to optimize various functions of embedded systems and illustrate it through code examples.
1. Use C for memory management optimization
In embedded systems, memory management is very important. C provides some tools and techniques to optimize memory management, such as using local objects instead of global objects, using object pools instead of frequent memory allocation operations, etc. The following uses the object pool as an example for explanation.
Object pool is a technology that pre-allocates objects and stores them in a pool. When the object needs to be used, it is obtained directly from the pool and put back into the pool after use. Object pools help reduce frequent memory allocation and destruction operations and improve system performance and stability.
#include <iostream> #include <vector> class Object { public: Object() { // 对象初始化操作 } ~Object() { // 对象销毁操作 } }; class ObjectPool { private: std::vector<Object*> pool; public: Object* getObject() { if (pool.empty()) { return new Object; } else { Object* obj = pool.back(); pool.pop_back(); return obj; } } void returnObject(Object* object) { pool.push_back(object); } }; int main() { ObjectPool objPool; Object* obj = objPool.getObject(); // 使用对象... objPool.returnObject(obj); return 0; }
2. Use C for power management optimization
In embedded systems, power management is an important optimization direction. C provides some techniques to reduce system power consumption, such as using the sleep function to reduce CPU usage frequency, using the system clock to control task execution, etc. The following uses the system clock to control the execution of tasks as an example.
#include <iostream> #include <ctime> void task() { // 执行任务的代码... } int main() { const unsigned int INTERVAL_MS = 1000; // 任务执行间隔时间,单位为毫秒 std::clock_t start = std::clock(); while (true) { std::clock_t now = std::clock(); double elapsed_ms = (now - start) / (double) (CLOCKS_PER_SEC / 1000); // 计算已经过去的时间,单位为毫秒 if (elapsed_ms >= INTERVAL_MS) { start = now; task(); } else { // 等待剩余时间 unsigned int remaining_ms = INTERVAL_MS - elapsed_ms; sleep(remaining_ms); } } return 0; }
3. Use C for real-time optimization
Real-time is a key requirement for embedded systems. C provides some techniques to improve the real-time performance of the system, such as using timers, using interrupt service routines, etc. The following is an example of using a timer to trigger task execution.
#include <iostream> #include <ctime> #include <signal.h> void task() { // 执行任务的代码... } void timer_handler(int sig) { task(); } int main() { const unsigned int INTERVAL_SEC = 1; // 任务执行间隔时间,单位为秒 struct sigaction sa; struct itimerval timer; memset(&sa, 0, sizeof(sa)); sa.sa_handler = &timer_handler; sigaction(SIGALRM, &sa, NULL); timer.it_value.tv_sec = INTERVAL_SEC; timer.it_value.tv_usec = 0; timer.it_interval.tv_sec = INTERVAL_SEC; timer.it_interval.tv_usec = 0; setitimer(ITIMER_REAL, &timer, NULL); while (true) { // 等待任务的触发 sleep(1); } return 0; }
Summary:
This article introduces how to use C language to optimize various functions of embedded systems, mainly including memory management optimization, power management optimization and real-time optimization. By rationally using the tools and technologies provided by C language, the performance, stability and real-time performance of embedded systems can be improved. At the same time, the above sample code is for reference only, and specific optimization methods and technologies should be selected and applied based on actual needs and specific systems.
The above is the detailed content of How to use C++ language to optimize various functions of embedded systems. For more information, please follow other related articles on the PHP Chinese website!
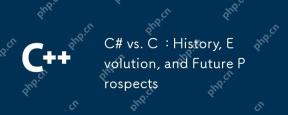
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
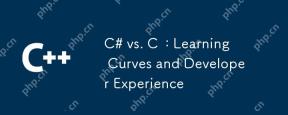
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
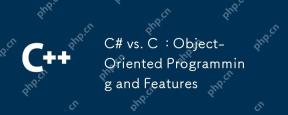
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
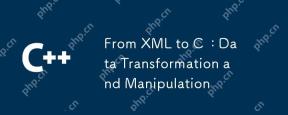
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
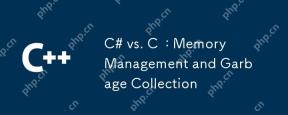
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
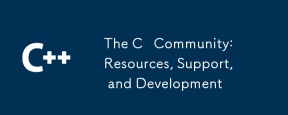
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
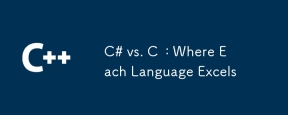
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
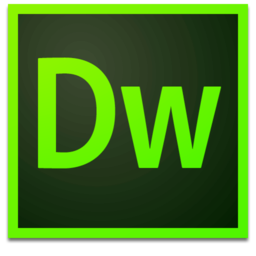
Dreamweaver Mac version
Visual web development tools
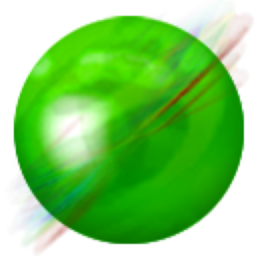
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
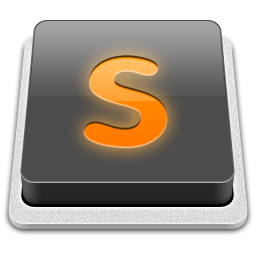
SublimeText3 Mac version
God-level code editing software (SublimeText3)