How to use Vue to implement comprehensive statistical chart navigation
Introduction:
In modern Web development, using charts to display data has become a very common need. In the Vue framework, it is very simple to use the chart library to visualize data. This article will introduce how to use Vue to implement comprehensive statistical chart navigation, and provide some code examples for reference.
1. Preparation
Before we start, we need to prepare some basic environment. First, we need to install Vue.js, which can be installed through npm or yarn. Enter the following command on the command line to initialize a new Vue project:
npm install -g @vue/cli vue create chart-navigation
Enter the project directory and run the following command to add Vue Router and Chart.js:
cd chart-navigation npm install --save vue-router chart.js
2. Create the project structure
We will create the following file and folder structure:
src ├── components │ ├── BarChart.vue │ ├── LineChart.vue │ └── PieChart.vue ├── router │ └── index.js └── App.vue
3. Set up routing
In the router/index.js
file, we will set up routing to navigate to different chart component. Please follow the following sample code to set up:
import Vue from 'vue' import VueRouter from 'vue-router' import BarChart from '@/components/BarChart.vue' import LineChart from '@/components/LineChart.vue' import PieChart from '@/components/PieChart.vue' Vue.use(VueRouter) const routes = [ { path: '/bar', component: BarChart }, { path: '/line', component: LineChart }, { path: '/pie', component: PieChart } ] const router = new VueRouter({ mode: 'history', routes }) export default router
4. Create chart components
In the components
folder, we will create three components: BarChart.vue
, LineChart.vue
and PieChart.vue
. Please create these files based on the following sample code:
BarChart.vue:
<template> <div> <h1 id="柱状图">柱状图</h1> <canvas ref="chart"></canvas> </div> </template> <script> import Chart from 'chart.js'; export default { mounted() { var ctx = this.$refs.chart.getContext('2d'); new Chart(ctx, { type: 'bar', data: { labels: ['A', 'B', 'C', 'D', 'E'], datasets: [{ label: '数据', data: [10, 20, 30, 40, 50], backgroundColor: ['#FF6384', '#36A2EB', '#FFCE56', '#008000', '#800080'] }] }, options: {} }); } } </script>
LineChart.vue:
<template> <div> <h1 id="折线图">折线图</h1> <canvas ref="chart"></canvas> </div> </template> <script> import Chart from 'chart.js'; export default { mounted() { var ctx = this.$refs.chart.getContext('2d'); new Chart(ctx, { type: 'line', data: { labels: ['A', 'B', 'C', 'D', 'E'], datasets: [{ label: '数据', data: [10, 20, 30, 40, 50], borderColor: '#FF6384', fill: false }] }, options: {} }); } } </script>
PieChart.vue:
<template> <div> <h1 id="饼状图">饼状图</h1> <canvas ref="chart"></canvas> </div> </template> <script> import Chart from 'chart.js'; export default { mounted() { var ctx = this.$refs.chart.getContext('2d'); new Chart(ctx, { type: 'pie', data: { labels: ['A', 'B', 'C', 'D', 'E'], datasets: [{ label: '数据', data: [10, 20, 30, 40, 50], backgroundColor: ['#FF6384', '#36A2EB', '#FFCE56', '#008000', '#800080'] }] }, options: {} }); } } </script>
5. Using routes and components
In the App.vue
file, we will use the <router-view></router-view>
component to display the components matched by the current route. Please set it up according to the following sample code:
<template> <div> <h1 id="统计图表导航">统计图表导航</h1> <nav> <router-link to="/bar">柱状图</router-link> <router-link to="/line">折线图</router-link> <router-link to="/pie">饼状图</router-link> </nav> <router-view></router-view> </div> </template> <script> export default { } </script>
6. Completion
Now, we have completed a comprehensive statistical chart navigation using Vue Router and Chart.js. By setting corresponding paths on routes, we can navigate between different charts. Each chart component can use Chart.js to create and render the corresponding chart.
For example, when we visit http://localhost:8080/bar
, a histogram will be displayed; when we visit http://localhost:8080/line
, a line chart will be displayed; when we visit http://localhost:8080/pie
, a pie chart will be displayed.
Summary:
This article introduces how to use Vue to achieve comprehensive statistical chart navigation and provides some code examples. By using routes and components in Vue, we can easily navigate between different charts and create and render charts with Chart.js. Hope this article can be helpful to everyone.
The above is the detailed content of How to use Vue to implement comprehensive statistical chart navigation. For more information, please follow other related articles on the PHP Chinese website!
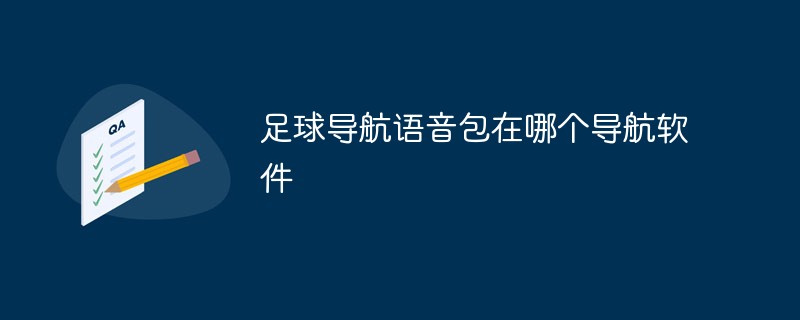
足球导航语音包在“高德导航”软件中,是高德地图车机版导航语音包的其中一种,内容为黄健翔足球解说版本的导航语音。设置方法:1、打开高德地图软件;2、点击进入“更多工具”-“导航语音”选项;3、找到“黄健翔热血语音”,点击“下载”;4、在弹出的页面,点击“使用语音”即可。
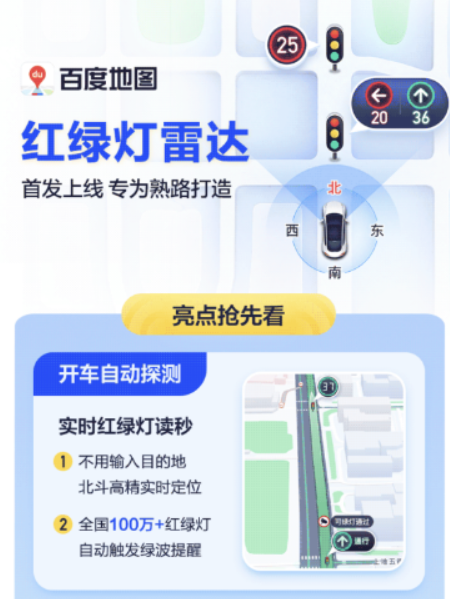
百度地图App安卓版/iOS版均已发布18.8.0版本,首次引入红绿灯雷达功能,业内领先据官方介绍,开启红绿灯雷达后,支持开车自动探测红绿灯,不用输入目的地,北斗高精可以实时定位,全国100万+红绿灯自动触发绿波提醒。除此之外,新功能还提供全程静音导航,使图区更简洁,关键信息一目了然,且无语音播报,使驾驶员更加专注驾驶百度地图于2020年10月上线红绿灯倒计时功能,支持实时读秒预判,导航会在接近红绿灯路口时,自动展示倒计时剩余秒数,让用户时刻掌握前方路况。截至2022年12月31日,红绿灯倒计时
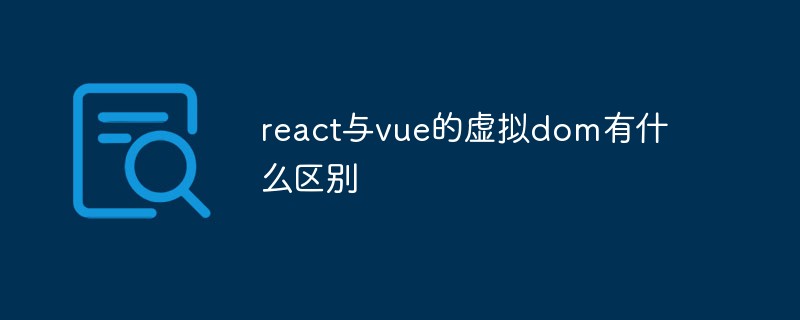
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。

在VSCode中开发Vue/React组件时,怎么实时预览组件?本篇文章就给大家分享一个VSCode 中实时预览Vue/React组件的插件,希望对大家有所帮助!
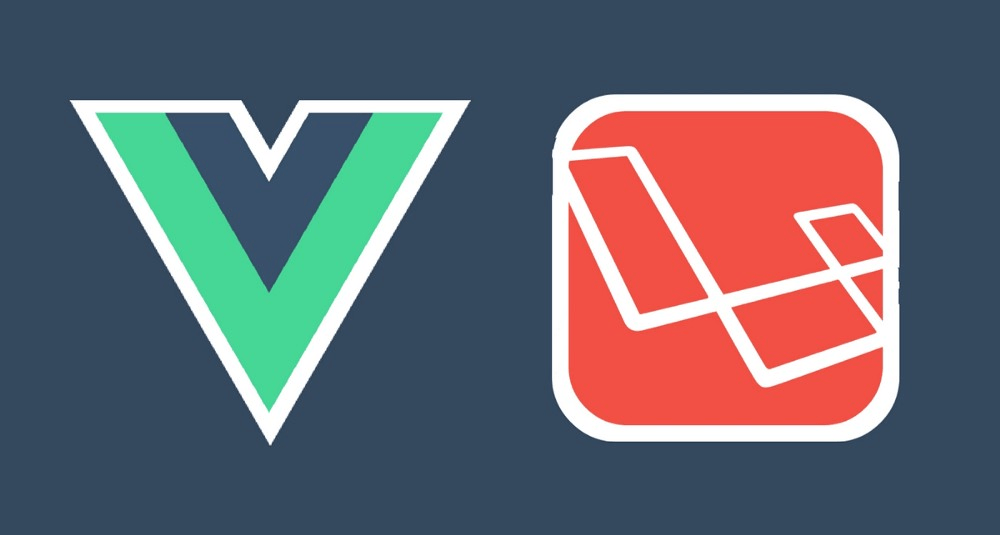
本篇文章给大家分享一个Vue+Laravel开发教程,介绍一下怎么使用 Vue.js 和 Laravel 共建一个简单的 CRUD 应用,希望对大家有所帮助!
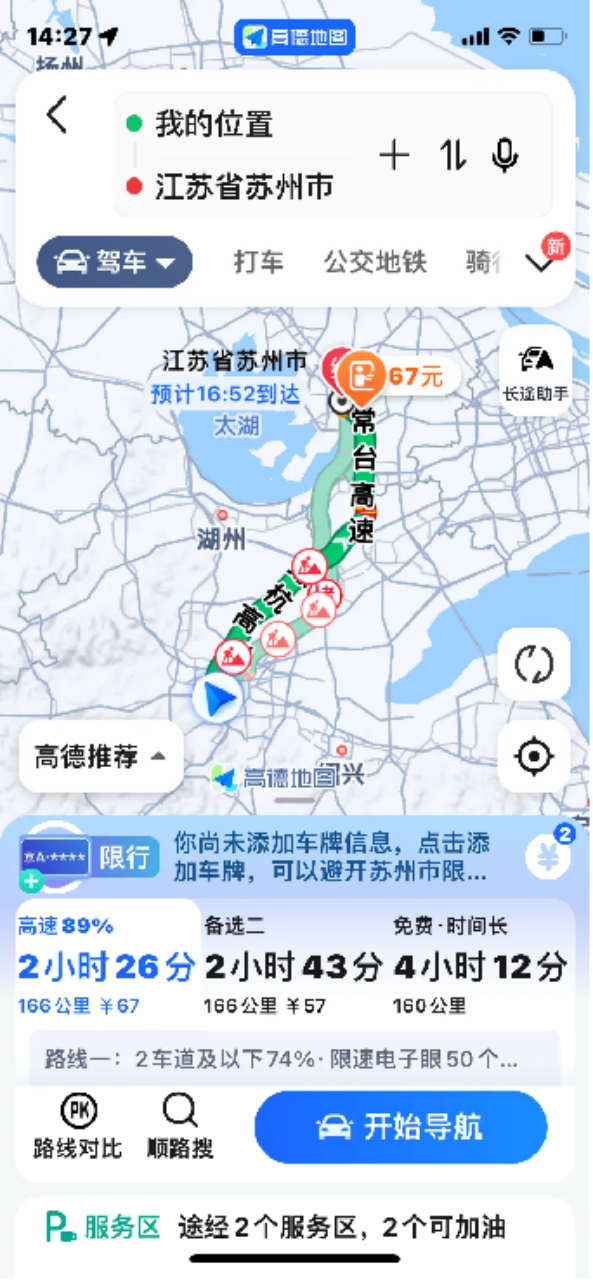
本站4月29日消息,高德地图官宣推出升级版的驾车ETA(本站注:ETA即预估到达时间,指的是用户在当前时刻出发按照给定路线前往目的地预计需要的时长)服务,该服务旨在帮助用户的路线规划时长和路况预估更为精准,辅助用户进行出行决策。该地图应用是最新升级的高德地图App,引入了“超大规模图卷积神经网络模型”,该模型可以更好地捕捉和学习交通流动规律,支持城市道路网络、高速公路系统,能以高精度刻画交通状况的时空动态变化。在此外,全新版本的地图还进一步融合了iTransformer时序预测模型,支持实时解析
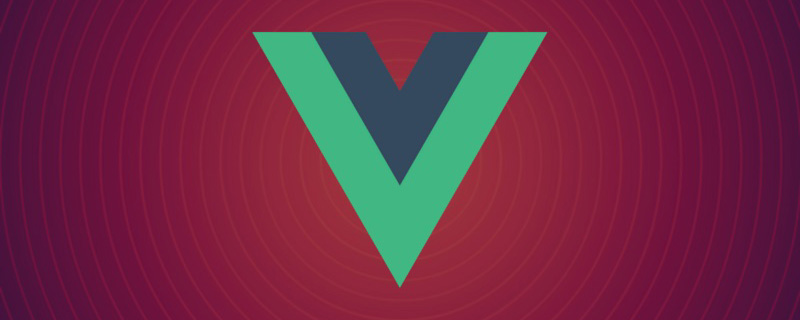
vue3为什么快?vue3的效率提升主要在哪方面?下面本篇文章就来给大家介绍一下vue3相对于vue2 做了那些优化,希望对大家有所帮助!


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
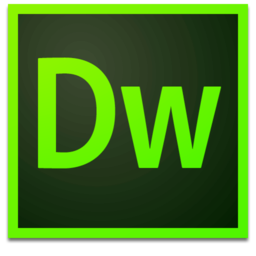
Dreamweaver Mac version
Visual web development tools
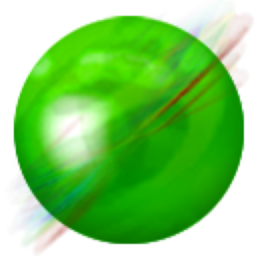
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
