


Analysis of application cases of various functional modules of C++ language in embedded systems
A case analysis of the application of various functional modules of C language in embedded systems
Abstract:
Embedded systems refer to the application of computer technology to various electronic A computer system in a device. The C language has become a commonly used language in embedded system development due to its rich functionality and flexibility. This article will be based on actual application cases, discuss the application of C language in various functional modules in embedded systems, and provide relevant code examples.
- Input/output module
In embedded systems, input/output modules play a very important role. C's iostream library provides rich input/output functions to facilitate data interaction with external devices. Let's take a simple LED control case as an example:
#include <iostream> #include <wiringPi.h> int main() { wiringPiSetupGpio(); pinMode(17, OUTPUT); while (true) { char cmd; std::cout << "请输入1(开)或0(关): "; std::cin >> cmd; if (cmd == '1') { digitalWrite(17, HIGH); } else if (cmd == '0') { digitalWrite(17, LOW); } else { std::cout << "输入无效,请重新输入!" << std::endl; } } return 0; }
The above code uses the wiringPi library to control the GPIO port output, and controls the on/off status of the LED light according to the commands entered by the user.
- Timer module
In embedded systems, the timer function is very important and can be used to implement scheduled tasks, delays and other functions. C's chrono library provides high-precision time calculation functions and can easily implement timer functions. Let's take a simple timer case as an example:
#include <iostream> #include <chrono> #include <thread> int main() { auto start = std::chrono::steady_clock::now(); while (true) { auto end = std::chrono::steady_clock::now(); auto duration = std::chrono::duration_cast<std::chrono::seconds>(end - start).count(); std::cout << "已经运行了" << duration << "秒!" << std::endl; std::this_thread::sleep_for(std::chrono::seconds(1)); } return 0; }
The above code uses the chrono library to calculate the running time of the program, and uses the sleep_for function of the thread library to achieve output every 1 second. . Through the timer function, various time-related functions can be realized, such as collecting data at regular intervals, sending data at regular intervals, etc.
- Communication module
In embedded systems, communication modules are often used for data transmission between devices, such as serial communication, network communication, etc. C provides rich communication functions and can easily interact with different external devices. The following is a simple serial communication case as an example:
#include <iostream> #include <wiringPi.h> #include <wiringSerial.h> int main() { int fd = serialOpen("/dev/ttyAMA0", 9600); if (fd == -1) { std::cout << "串口打开失败!" << std::endl; return -1; } while (true) { if (serialDataAvail(fd)) { char data = serialGetchar(fd); std::cout << "接收到的数据: " << data << std::endl; } } return 0; }
The above code uses the wiringSerial library for serial communication, opens the serial port through the serialOpen function, and uses the serialDataAvail function to determine whether the serial port has readable data. Get it and use the serialGetchar function to read the serial port data and output it. Through the application of the communication module, data interaction with other devices can be easily realized and various functions can be realized.
Conclusion:
This article takes the input/output module, timer module and communication module as examples to introduce the application of C language in various functional modules in embedded systems and provides relevant code examples. . C language has become a commonly used language in embedded system development due to its rich functionality and flexibility. By rationally applying each functional module, the functional requirements of various embedded systems can be easily realized.
The above is the detailed content of Analysis of application cases of various functional modules of C++ language in embedded systems. For more information, please follow other related articles on the PHP Chinese website!
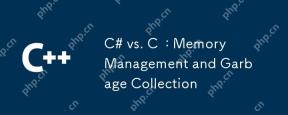
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
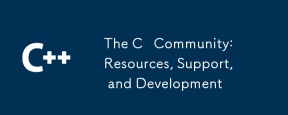
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
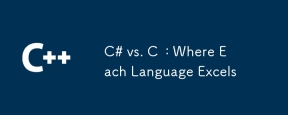
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
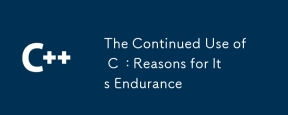
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
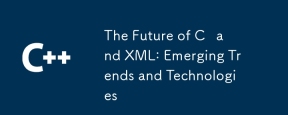
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
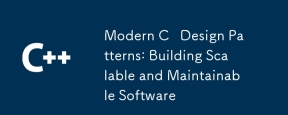
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
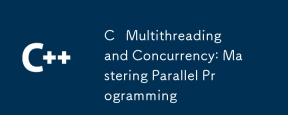
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
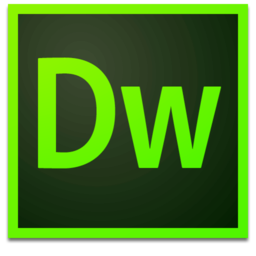
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.