


How to find the smallest element in an array using binary search algorithm in C language?
The C programming language provides two search techniques. They are as follows:
- Linear search
- Binary search
Binary search
- This method only works for Ordered list.
- The given list is divided into two equal parts.
- The given keyword is compared to the middle element of the list.
Here, three things can happen, as follows:
If the middle element matches the keyword, the search will end successfully here
If the middle element is larger than the keyword, the search will be performed on the left partition.
If the middle element is smaller than the keyword, the search will be performed on the right partition.
Input (i/p) - Unsorted list of elements, keyword.
Output (o/p) -
- Success - If keyword found
- Failure - Otherwise
key = 20 mid = (low +high) /2
Program 1
The following is a C program to find the smallest element in an array using binary search:
#include<stdio.h> int main(){ int a[50], n, i, key, flag = 0, low, mid, high; printf("enter the no: of elements:"); scanf ("%d",&n); printf("enter the elements:"); for(i=0; i<n; i++) scanf( "%d", &a[i]); printf("enter a key element:"); scanf ("%d", &key); low = 0; high = n-1; while (low<= high ){ mid = (low + high) /2; if (a[mid] == key){ flag = 1; break; } else{ if (a[mid] > key) high = mid-1; else low = mid+1; } } if (flag == 1) printf ("search is successful"); else printf("search is unsuccessful"); return 0; }
Output
When the above program is executed, it produces the following result −
Run 1: enter the no: of elements:5 enter the elements: 12 34 11 56 67 enter a key element:45 search is unsuccessful Run 2: enter the no: of elements:3 enter the elements: 12 34 56 enter a key element:34 search is successful
Program2
Given the following C program, it is found by using binary search Minimum element in the array −
#include<stdio.h> void Bmin(int *a, int i, int n){ int j, temp; temp = a[i]; j = 2 * i; while (j <= n){ if (j < n && a[j+1] > a[j]) j = j + 1; if (temp < a[j]) break; else if (temp >= a[j]){ a[j / 2] = a[j]; j = 2 * j; } } a[j/2] = temp; return; } int binarysearchmin(int *a,int n){ int i; for(i = n/2; i >= 1; i--){ Bmin(a,i,n); } return a[1]; } int main(){ int n, i, x, min; int a[20]; printf("Enter no of elements in an array</p><p>"); scanf("%d", &n); printf("</p><p>Enter %d elements: ", n); for (i = 1; i <= n; i++){ scanf("%d", &a[i]); } min = binarysearchmin(a, n); printf("\minimum element in an array is : %d", min); return 0; }
Output
When the above program is executed, it produces the following result−
Enter no of elements in an array 5 Enter 5 elements: 12 23 34 45 56 minimum element in an array is: 12
The above is the detailed content of How to find the smallest element in an array using binary search algorithm in C language?. For more information, please follow other related articles on the PHP Chinese website!
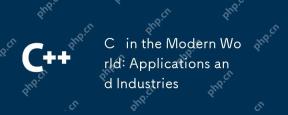
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
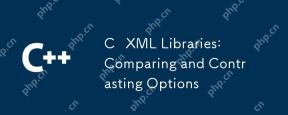
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
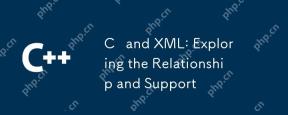
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
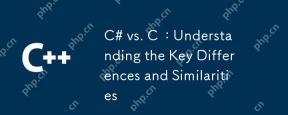
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
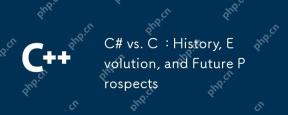
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
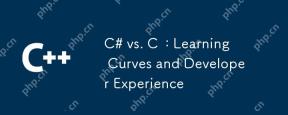
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
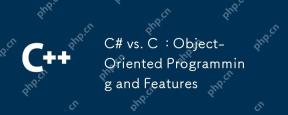
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
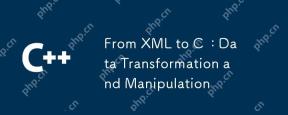
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),