


How to deal with data compression and decompression issues in C++ big data development?
How to deal with data compression and decompression issues in C big data development?
Introduction:
In modern big data applications, data compression and decompression are a A very important technology. Data compression can reduce the space occupied by data during storage and transmission, thereby speeding up data transmission and reducing storage costs. This article will introduce how to deal with data compression and decompression issues in C big data development, and provide relevant code examples.
1. Data Compression
Data compression is the process of converting original data into a more compact format. In C, we can use various compression algorithms to compress data, such as Gzip, Deflate, etc. The following is a code example that uses the Gzip algorithm for data compression:
#include <iostream> #include <fstream> #include <sstream> #include <string> #include <cassert> #include <zlib.h> std::string compressData(const std::string& input) { z_stream zs; // z_stream is zlib's control structure memset(&zs, 0, sizeof(zs)); if (deflateInit(&zs, Z_DEFAULT_COMPRESSION) != Z_OK) throw(std::runtime_error("deflateInit failed while compressing.")); zs.next_in = (Bytef*)input.data(); zs.avail_in = input.size(); // set the z_stream's input int ret; char outbuffer[32768]; std::string outstring; // retrieve the compressed bytes blockwise do { zs.next_out = reinterpret_cast<Bytef*>(outbuffer); zs.avail_out = sizeof(outbuffer); ret = deflate(&zs, Z_FINISH); if (outstring.size() < zs.total_out) { // append the block to the output string outstring.append(outbuffer, zs.total_out - outstring.size()); } } while (ret == Z_OK); deflateEnd(&zs); if (ret != Z_STREAM_END) { // an error occurred that was not EOF std::ostringstream oss; oss << "Exception during zlib compression: (" << ret << ") " << zs.msg; throw(std::runtime_error(oss.str())); } return outstring; } int main() { std::string input = "This is a sample string to be compressed."; std::string compressed = compressData(input); std::cout << "Original size: " << input.size() << std::endl; std::cout << "Compressed size: " << compressed.size() << std::endl; return 0; }
2. Data decompression
Data decompression is the process of restoring compressed data to original data. In C, we can use the decompression function corresponding to the compression algorithm to decompress data. For example, the decompression function corresponding to Gzip is gunzip. The following is a code example using the Gzip algorithm for data decompression:
#include <iostream> #include <fstream> #include <sstream> #include <string> #include <cassert> #include <zlib.h> std::string decompressData(const std::string& input) { z_stream zs; // z_stream is zlib's control structure memset(&zs, 0, sizeof(zs)); if (inflateInit(&zs) != Z_OK) throw(std::runtime_error("inflateInit failed while decompressing.")); zs.next_in = (Bytef*)input.data(); zs.avail_in = input.size(); int ret; char outbuffer[32768]; std::string outstring; // get the decompressed bytes blockwise using repeated calls to inflate do { zs.next_out = reinterpret_cast<Bytef*>(outbuffer); zs.avail_out = sizeof(outbuffer); ret = inflate(&zs, 0); if (outstring.size() < zs.total_out) { outstring.append(outbuffer, zs.total_out - outstring.size()); } } while (ret == Z_OK); inflateEnd(&zs); if (ret != Z_STREAM_END) { // an error occurred that was not EOF std::ostringstream oss; oss << "Exception during zlib decompression: (" << ret << ") " << zs.msg; throw(std::runtime_error(oss.str())); } return outstring; } int main() { std::string decompressed = decompressData(compressed); std::cout << "Compressed size: " << compressed.size() << std::endl; std::cout << "Decompressed size: " << decompressed.size() << std::endl; return 0; }
Conclusion:
This article introduces the method of handling data compression and decompression problems in C big data development, and provides relevant code Example. Through reasonable selection of compression algorithms and decompression functions, we can effectively reduce data storage and transmission overhead and improve program performance and efficiency during big data processing. It is hoped that readers can flexibly use this knowledge in practical applications to optimize their own big data applications.
The above is the detailed content of How to deal with data compression and decompression issues in C++ big data development?. For more information, please follow other related articles on the PHP Chinese website!
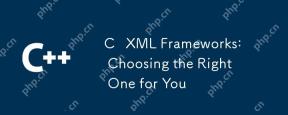
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
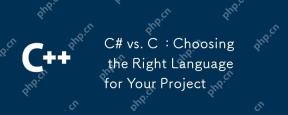
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
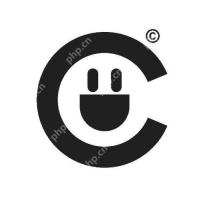
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
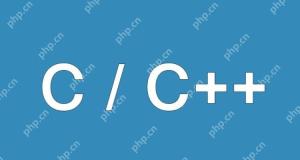
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
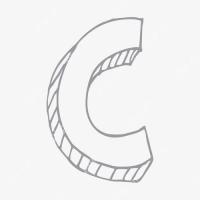
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
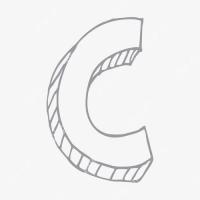
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
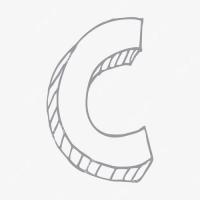
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
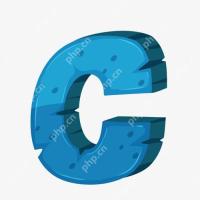
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
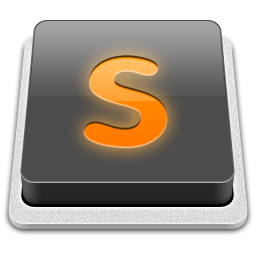
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
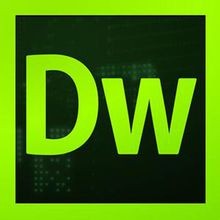
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
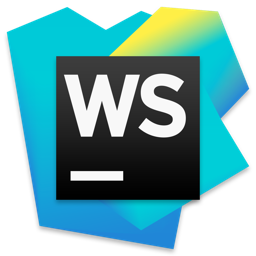
WebStorm Mac version
Useful JavaScript development tools
