


How to solve C++ compilation error: 'no matching function for call to 'function'?
Solution to C compilation error: 'no matching function for call to 'function', how to solve it?
When writing programs in C, we often encounter various compilation errors. One of the common errors is "no matching function for call to 'function'". This error usually occurs when a function is called and the compiler cannot find a matching function declaration or definition. This article details how to resolve this compilation error and provides some sample code.
First, let us look at a simple example:
#include <iostream> void add(int a, int b) { std::cout << "Sum: " << a + b << std::endl; } int main() { add(1, 2, 3); // 调用了错误的函数 return 0; }
In the above code, we define an add function to calculate the sum of two integers. In the main function, we mistakenly called the add function and passed three parameters. Since we did not provide an overloaded version of the add function that accepts three parameters, the compiler will not be able to find a matching function declaration or definition, resulting in a compilation error.
In order to solve this problem, we need to look at the error message and find the line of code that went wrong. The compiler usually provides some hints about the error in the error message, such as no matching function declaration or definition found, etc. Based on these tips, we can determine why the error occurred and modify the code accordingly.
In this example, the compiler will report an error: "no matching function for call to 'add'". This error message tells us that the add function we called did not find a matching function declaration or definition. In order to fix this error, we need to modify the parameters of the function call to ensure that they are consistent with the parameters defined by the function.
The way to fix the above error is to remove the redundant parameters so that the function call matches the function definition, as shown below:
#include <iostream> void add(int a, int b) { std::cout << "Sum: " << a + b << std::endl; } int main() { add(1, 2); return 0; }
In the modified code, we have removed the redundant parameters" 3" to match the function call to the function definition. This way, the compiler will be able to find the definition of the function named add and compile the program successfully.
In addition to mismatching function call parameters, there are other common reasons that can cause "no matching function for call to 'function'" errors. Listed below are some common situations and solutions.
- The function declaration and defined parameter types do not match.
For example, if the function declaration uses parameters of type int, and the function definition uses parameters of type float, the compiler will not be able to find a matching function declaration or definition. The solution is to ensure that the type and number of parameters are consistent between the function declaration and definition. - Function overloading conflict.
If there are multiple function overloads with the same parameter type and number, the compiler will not be able to determine which overloaded function to call. The solution is to provide function overloads with more specific parameter types, or to explicitly cast the parameters to the required type. - The function is not declared or defined.
Before calling a function, we usually need to provide a declaration or definition of the function so that the compiler can find and link the function. If the function is not declared or defined, the compiler will report an error "no matching function for call to 'function'". The solution is to provide a declaration or definition of the function.
To sum up, when we solve the C compilation error "no matching function for call to 'function'", we first need to check the error message and determine the cause of the error. We can then use the hints provided by the error message to modify the code to ensure that the function call matches the function declaration or definition. By correctly modifying the parameters of the calling function, we can successfully resolve this compilation error so that the program can compile and run successfully.
The above is the detailed content of How to solve C++ compilation error: 'no matching function for call to 'function'?. For more information, please follow other related articles on the PHP Chinese website!
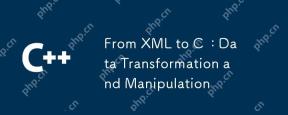
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
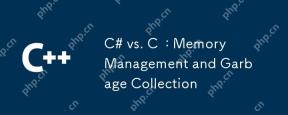
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
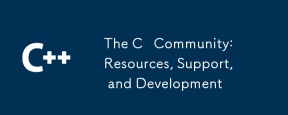
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
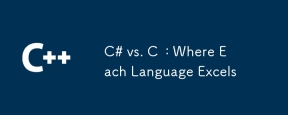
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
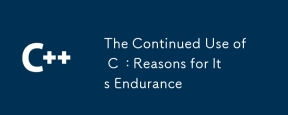
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
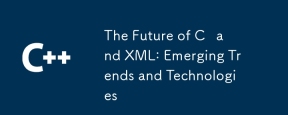
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
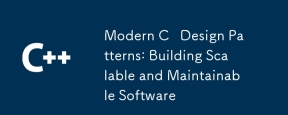
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
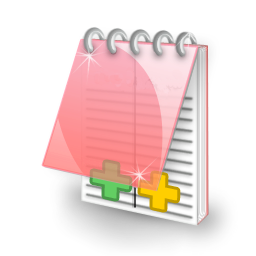
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
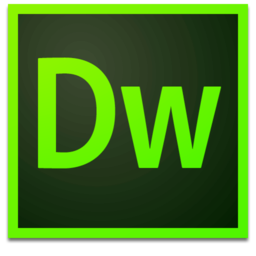
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor