How to use Python to perform pattern recognition on pictures
Introduction
With the rapid development of computer vision, image processing and pattern recognition have become popular field of study. Using computers to perform pattern recognition on images can play an important role in many applications, such as face recognition, object detection, and medical image analysis. This article will introduce how to use the Python programming language and related image processing libraries to perform pattern recognition on images, and use code examples to help readers better understand and apply pattern recognition technology.
- Install Python and related libraries
First, in order to start using Python for pattern recognition, we need to install the Python interpreter. Currently, Python 3.x is the latest version. You can download and install it from the official website (https://www.python.org).
In order to perform image processing and pattern recognition, we also need to install some Python libraries. The most commonly used ones are NumPy, OpenCV and Scikit-learn. You can use the pip command to install these libraries:
pip install numpy opencv-python scikit-learn
- Image reading and display
Before doing pattern recognition, we need to read the image and display it first come out. Python provides multiple libraries for image processing, the most commonly used of which is OpenCV. Here is a simple code example that reads an image and displays it:
import cv2 # 读取图像 image = cv2.imread('image.jpg') # 显示图像 cv2.imshow('Image', image) cv2.waitKey(0) cv2.destroyAllWindows()
In the code, we have used the cv2.imread function to read an image named image.jpg and use cv2. The imshow function displays the image. cv2.waitKey(0) is used to wait for keyboard input, and cv2.destroyAllWindows is used to close the image window.
- Image preprocessing
Before pattern recognition, we usually need to preprocess the image to improve the accuracy of pattern recognition. Image preprocessing includes image enhancement, noise reduction, size scaling and other operations.
Here is a simple code example that demonstrates how to resize an image:
import cv2 # 读取图像 image = cv2.imread('image.jpg') # 缩放图像 resized_image = cv2.resize(image, (300, 300)) # 显示缩放后的图像 cv2.imshow('Resized Image', resized_image) cv2.waitKey(0) cv2.destroyAllWindows()
In the code, we use the cv2.resize function to resize the image to a size of 300x300 and use cv2 The .imshow function displays the zoomed image.
- Feature extraction and model training
Feature extraction is one of the key steps in pattern recognition. In image processing, we usually use feature descriptors (such as grayscale histograms, gradient histograms, color histograms, etc.) to represent features in images.
The following is a simple code example showing how to use a grayscale histogram to describe image features:
import cv2 # 读取图像 image = cv2.imread('image.jpg') # 将图像转为灰度图像 gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # 计算灰度直方图 histogram = cv2.calcHist([gray_image], [0], None, [256], [0,256]) # 显示灰度直方图 import matplotlib.pyplot as plt plt.plot(histogram) plt.show()
In the code, we use the cv2.cvtColor function to convert the image to a grayscale image , and then use the cv2.calcHist function to calculate the grayscale histogram. Finally, the matplotlib library is used to display the histogram.
Before pattern recognition, it is usually necessary to use some machine learning algorithms to train the model. We can use the Scikit-learn library to train machine learning models and use the trained models for pattern recognition. We will not introduce the principles and algorithms of machine learning in detail here. Readers can refer to the official Scikit-learn documentation to learn.
Conclusion
This article introduces the basic steps of how to use Python to perform pattern recognition on images, and gives practical operations through code examples. It is hoped that through the introduction of this article, readers can understand and master the basic knowledge of image processing and pattern recognition, and further expand the application fields.
Pattern recognition is a broad research field. This article only gives some simple examples. Readers can conduct more in-depth research and learning based on their actual needs. Through continuous practice and exploration, I believe you can achieve better results in image processing and pattern recognition.
The above is the detailed content of How to use Python to perform pattern recognition on pictures. For more information, please follow other related articles on the PHP Chinese website!
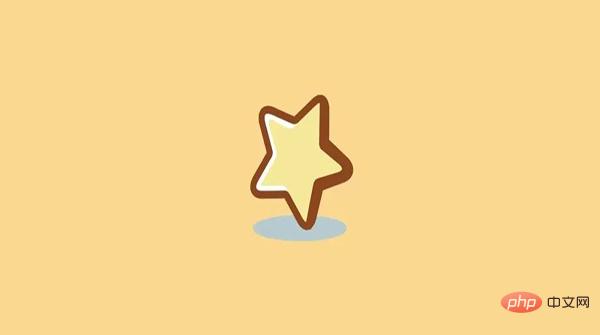
网上下载的 pdf 学习资料有一些会带有水印,非常影响阅读。比如下面的图片就是在 pdf 文件上截取出来的,今天我们就来用Python解决这个问题。安装模块PIL:Python Imaging Library 是 python 上非常强大的图像处理标准库,但是只能支持 python 2.7,于是就有志愿者在 PIL 的基础上创建了支持 python 3的 pillow,并加入了一些新的特性。pip install pillow pymupdf 可以用 python 访问扩展名为*.pdf、
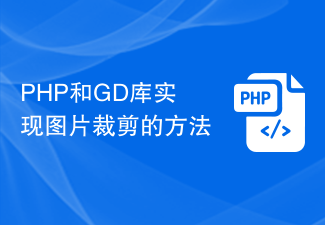
PHP和GD库实现图片裁剪的方法概述:图片裁剪是网页开发中常见的需求之一,它可以用于调整图片的尺寸,剪裁不需要的部分,以适应不同的页面布局和展示需求。在PHP开发中,我们可以借助GD库来实现图片裁剪的功能。GD库是一个强大的图形库,可提供一系列函数来处理和操控图像。代码示例:下面我们将详细介绍如何使用PHP和GD库来实现图片裁剪。首先,确保你的PHP环境已经
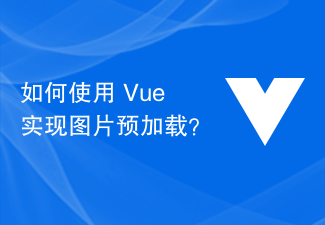
在网页开发中,图片预载是一种常见的技术,可以提升用户的体验感。当用户浏览网页时,图片可以提前下载并加载,减少图片加载时的等待时间。在Vue框架中,我们可以通过一些简单的方法来实现图片预载。本文将介绍Vue中的图片预载技术,包括预载的原理、实现的方法和使用注意事项。一、预载的原理首先,我们来了解一下图片预载的原理。传统的图片加载方式是等到图片全部下载完成才显示
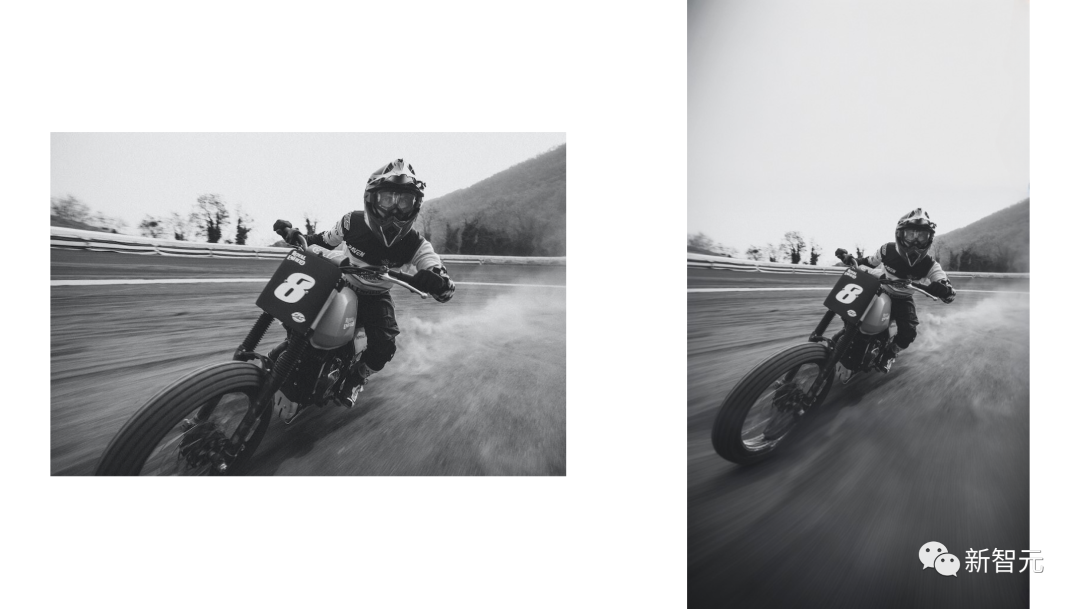
此前,PS的重建图像功能就让人无比振奋,让无数人惊呼今天,StabilityAI又放大招了。它联合Clipdrop推出了UncropClipdrop——一个终极图像比例编辑器。从Uncrop这个名字上,我们就能看出它的用途。它是一个AI生成的「外画」工具,通过创建扩展背景,这个工具可以补充任何现有照片或图像,来更改任何图像的比例。敲黑板:通过Clipdrop网站,就可以免费试用这个工具了,无需登录!比例任意调,满意为止Uncrop基于StabilityAI的文本到图像模型StableDiffus
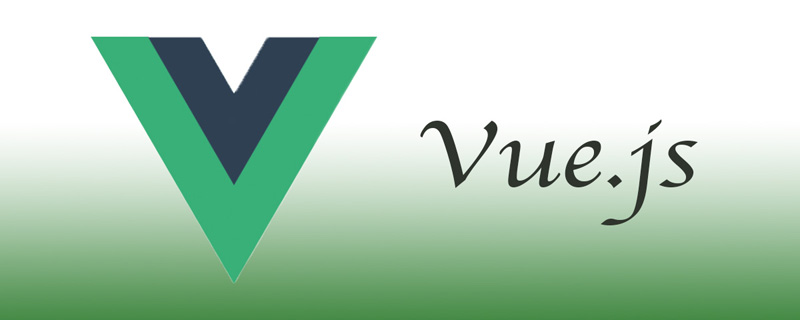
vue报错找不到图片的解决办法:1、修改配置文件,将绝对路径改为相对路径;2、将图片作为模块加载进去,并将图片放到static目录下;3、将imageUrls引入响应的vue文件中,解析引用即可。
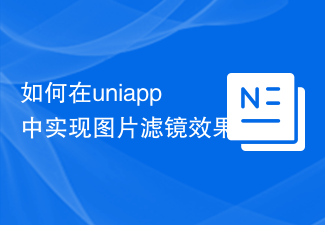
如何在uniapp中实现图片滤镜效果在移动应用开发中,图片滤镜效果是一种常见且受用户喜爱的功能之一。而在uniapp中,实现图片滤镜效果也并不复杂。本文将为大家介绍如何通过uniapp实现图片滤镜效果,并附上相关代码示例。导入图片首先,我们需要在uniapp项目中导入一张图片,以供后续滤镜效果的处理。可以在项目的资源文件夹中放置一张命名为“filter.jp
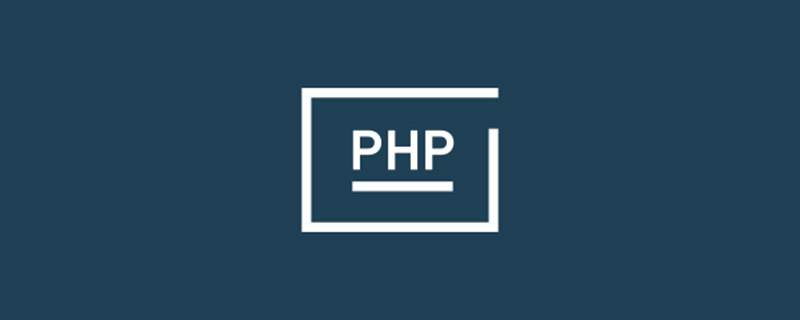
php写图片不显示不出来的解决办法:1、找到并打开php.ini文件;2、找到“extension=php_gd2.dll”,并将前面的分号去掉;3、重新启动服务器;4、在绘图前清一下缓存即可。
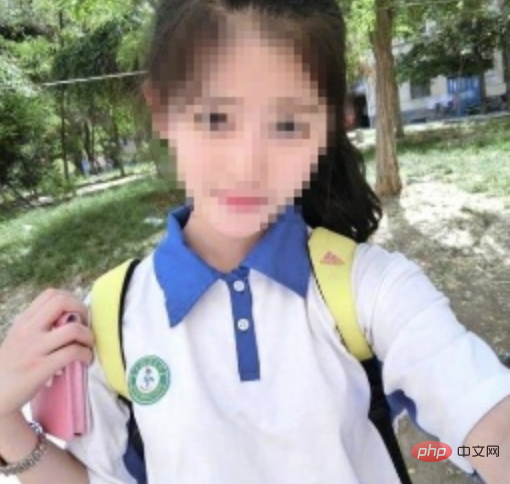
哈喽,大家好。你有没有想过用 AI 技术去除马赛克?仔细想想这个问题还挺难的,因为我们之前使用的 AI 技术,不管是人脸识别还是OCR识别,起码人工能识别出来。但如果给你一张打上马赛克的图片,你能把它复原吗?显然是很难的。如果人都无法复原,又怎能教会计算机去复原呢?还记得前几天我写的一篇《用AI生成头像》文章吗。在那篇文章中,我们训练了一个DCGAN模型,它可以从任意随机数生成一个图像。随机数作为像素生成的噪声图模型从随机数生成正常头像DCGAN包含生成器模型和判别器模型两个模型组成,生成


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
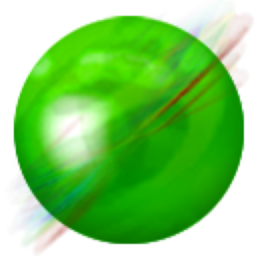
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
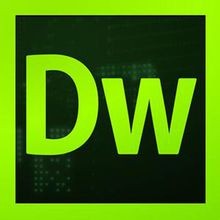
Dreamweaver CS6
Visual web development tools
