


How do I create a function that calls each provided function using JavaScript?
In JavaScript, functions are first-class objects, which means they can be passed as arguments to other functions. This is a powerful feature that can be used to create some interesting patterns.
One such pattern is the "invoke-each" pattern, where a function is created that calls each provided function with the arguments it receives.
Why use Invoke-Each mode?
There are several reasons why you might want to use the invoke-each pattern.
First, it can be used as a way to abstract away the details of how a set of functions are called. This is helpful if the function is called in a complex or repetitive way.
Second, it can be used to create some kind of "pipeline" where the output of each function is passed as input to the next function in the chain. This is a convenient way to string together a series of actions.
Finally, it can be used to create a "tee" function that calls multiple functions with the same parameters and collects the results. This is useful for logging, debugging, or other purposes.
How to implement the Invoke-Each pattern?
There are several different ways to implement calling each pattern.
The most straightforward approach is to simply loop through the provided functions and call each function with the arguments -
function invokeEach(functions, args) { for (var i = 0; i < functions.length; i++) { functions[i].apply(null, args); } }
In the above code, we loop through the functions and call each function using the Function.prototype.apply() method. This method allows us to call a function with a specific this value and parameter array.
We use the apply() method here because this is a convenient way to pass parameters as an array. You can also use the Function.prototype.call() method, which allows you to pass parameters as separate values.
Example
Below is a complete working code example.
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <div id="result1"></div> <div id="result2"></div> <div id="result3"></div> <script> function foo(x) { document.getElementById("result").innerHTML = 'foo: ' + x; } function bar(x) { document.getElementById("result1").innerHTML = 'bar: ' + x; } function baz(x) { document.getElementById("result2").innerHTML = 'baz: ' + x; } function qux(x) { document.getElementById("result3").innerHTML ='qux: ' + x; } function invokeEach(functions, args) { for (var i = 0; i < functions.length; i++) { functions[i].apply(null, args); } } invokeEach([foo, bar, baz, qux], [5]); </script> </body> </html>
As you can see, the code example above defines four functions: foo(), bar(), baz(), and qux(). These functions simply print out a message using the provided parameters.
Next, we define the invokeEach() function, which accepts a function array and a parameter array. This function loops through the provided functions and calls each function with the provided arguments.
Finally, we call the invokeEach() function, passing in four functions and a single parameter 5. As expected, this results in every function being called with argument 5.
In this tutorial, we looked at the invoke-each pattern in JavaScript. This pattern can be used to create a function that calls each provided function with the arguments it receives.
We learned how to implement this pattern and some of the reasons why you might want to use it.
The above is the detailed content of How do I create a function that calls each provided function using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
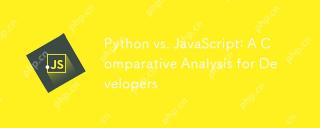
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
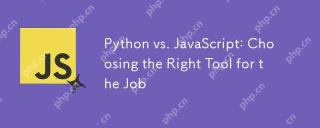
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
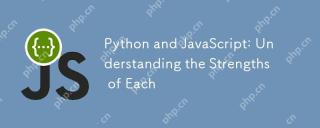
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
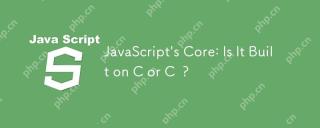
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
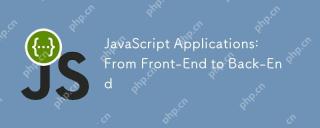
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
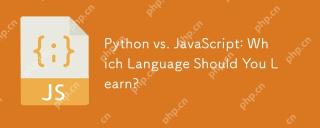
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
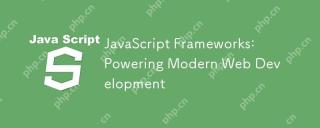
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
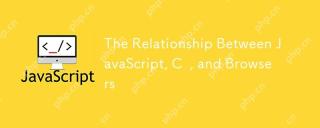
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
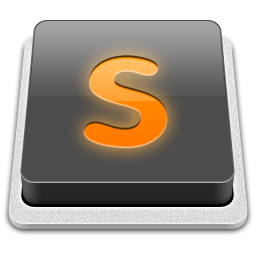
SublimeText3 Mac version
God-level code editing software (SublimeText3)
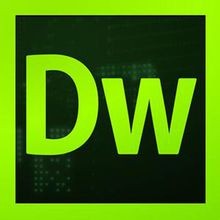
Dreamweaver CS6
Visual web development tools
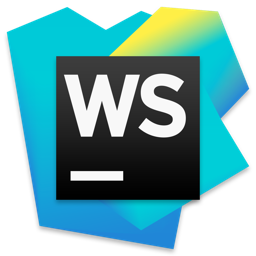
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
